How to build a great front-end user interface using Vue and Element-UI
How to build an excellent front-end user interface using Vue and Element-UI
Introduction:
In modern web development, building an excellent user interface is very important. With the continuous development of front-end frameworks, Vue has become one of the preferred front-end frameworks. When building user interfaces, Element-UI, as a set of component libraries based on Vue.js, is a very powerful and easy-to-use tool. This article will introduce how to use Vue and Element-UI to build an excellent front-end user interface, and provide corresponding code examples.
Step 1: Install Vue and Element-UI
First, we need to install Vue and Element-UI. Enter the following command on the command line to install Vue:
npm install vue
Then, we need to install Element-UI. Similarly, enter the following command on the command line to install Element-UI:
npm install element-ui
Step 2: Create a Vue project
After installing Vue and Element-UI, we can start creating a Vue project. Enter the following command at the command line:
vue create my-project
This will create a Vue project named "my-project". During the project creation process, you can make some choices as needed, such as selecting a preset configuration or configuring custom configuration options.
Step 3: Use Element-UI components
Using Element-UI components in Vue projects is very simple. Add the following code to the main.js file in the project:
import Vue from 'vue'; import ElementUI from 'element-ui'; import 'element-ui/lib/theme-chalk/index.css'; Vue.use(ElementUI); new Vue({ render: h => h(App), }).$mount('#app');
Here we first import Vue and Element-UI, and use Vue.use() to register all Element-UI components. Then in the Vue instance, we register Element-UI as a global component.
Now, we can use various components provided by Element-UI in Vue components. For example, we can add a button component to the App.vue file:
<template> <div> <el-button type="primary">这是一个按钮</el-button> </div> </template> <script> export default { name: 'App', } </script>
In the above code, we use the el-button component provided by Element-UI and set the button type to primary.
Step 4: Build the user interface
By using the components provided by Element-UI, we can easily build the user interface. The following are some commonly used Element-UI components and their usage examples:
- Button button component
<template> <div> <el-button type="primary">主要按钮</el-button> <el-button type="success">成功按钮</el-button> <el-button type="warning">警告按钮</el-button> <el-button type="danger">危险按钮</el-button> </div> </template> <script> export default { name: 'App', } </script>
- Input input box component
<template> <div> <el-input placeholder="请输入内容"></el-input> </div> </template> <script> export default { name: 'App', } </script>
- Table Table Component
<template> <div> <el-table :data="tableData"> <el-table-column prop="name" label="姓名"></el-table-column> <el-table-column prop="age" label="年龄"></el-table-column> <el-table-column prop="address" label="地址"></el-table-column> </el-table> </div> </template> <script> export default { name: 'App', data() { return { tableData: [ { name: '张三', age: 20, address: '北京' }, { name: '李四', age: 25, address: '上海' }, { name: '王五', age: 30, address: '广州' }, ] } } } </script>
By using the above example, we can see how to use Element-UI components to build a great user interface. You can also use other components provided by Element-UI according to actual needs, such as form components, date pickers, pop-up components, etc.
Summary:
This article introduces how to use Vue and Element-UI to build an excellent front-end user interface. By using the rich component library provided by Element-UI, we can easily build beautiful and feature-rich user interfaces. I hope this article can help everyone. If you have any questions, please feel free to leave a message. Good luck building great front-end user interfaces with Vue and Element-UI!
The above is the detailed content of How to build a great front-end user interface using Vue and Element-UI. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
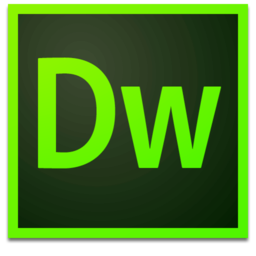
Dreamweaver Mac version
Visual web development tools
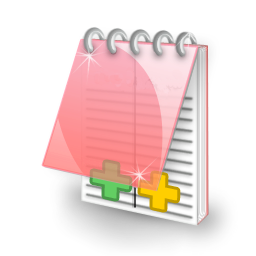
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
