


The elegant combination of Vue and Excel: how to achieve batch processing and export of data
The elegant combination of Vue and Excel: How to implement batch processing and export of data
In daily development work, we often encounter the need to process large amounts of data, and Excel, as a powerful Spreadsheet software is often used for data processing and analysis. So, how to use the Vue framework to implement batch processing and export of data? This article will give you a detailed introduction to how to achieve this requirement through Vue.
1. Preparation
Before we start, we need to install some dependency packages. Install the xlsx
and file-saver
packages through the npm command, which are used to read and write Excel files respectively, and save the files.
npm install xlsx file-saver --save
2. Batch processing of data
First, we need to define a data table in the Vue component for displaying and editing data. Suppose our data is a two-dimensional array, where each row represents an item and each column represents a different attribute of the item.
export default { data() { return { data: [ ['项目名称', '项目描述', '开始时间', '结束时间'], ['项目A', '这是项目A的描述', '2022-01-01', '2022-03-01'], ['项目B', '这是项目B的描述', '2022-04-01', '2022-06-01'], ['项目C', '这是项目C的描述', '2022-07-01', '2022-09-01'], ] } } }
Next, we can use the table
tag to render the data table and traverse the data through the v-for
instruction.
<table> <tr v-for="(row, index) in data" :key="index"> <td v-for="(cell, cellIndex) in row" :key="cellIndex">{{ cell }}</td> </tr> </table>
Then, we can add some action buttons to process the data. For example, we can add a delete button at the end of each row and delete the data in that row when the button is clicked.
<table> <tr v-for="(row, index) in data" :key="index"> <td v-for="(cell, cellIndex) in row" :key="cellIndex">{{ cell }}</td> <td> <button @click="deleteRow(index)">删除</button> </td> </tr> </table>
In the method of the Vue component, we define the deleteRow
method to implement the deletion function.
methods: { deleteRow(index) { this.data.splice(index, 1); } }
In this way, we have implemented the batch processing function of data. The user can delete a certain row in the data table by clicking the delete button.
3. Export of data
Next, we will introduce how to export data to an Excel file. In the Vue component, we define a method named exportData
.
import XLSX from 'xlsx'; import { saveAs } from 'file-saver'; methods: { exportData() { const worksheet = XLSX.utils.json_to_sheet(this.data); const workbook = XLSX.utils.book_new(); XLSX.utils.book_append_sheet(workbook, worksheet, 'Sheet1'); const excelBuffer = XLSX.write(workbook, { bookType: 'xlsx', type: 'array' }); const data = new Blob([excelBuffer], { type: 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet' }); saveAs(data, 'data.xlsx'); } }
By using the xlsx
library, we convert the data into an Excel worksheet and add the worksheet to the workbook. Then, we convert the workbook into binary data and save it as an Excel file through the file-saver
library.
Finally, add an export button to the template in the Vue component and bind it to the exportData
method.
<button @click="exportData">导出Excel</button>
Now, when the user clicks the export button, the browser will automatically download an Excel file named data.xlsx
, which contains all the data in the data table.
4. Summary
Through the introduction of this article, we have learned how to use the Vue framework to implement batch processing and export functions of data. By defining data tables and operation buttons, we can add, delete, and modify data. By using the two libraries xlsx
and file-saver
, we can export the data to Excel files and save and share the data conveniently. This elegant combination brings great convenience to our development work.
We can further extend this example, such as adding data editing and sorting functions, or importing data from Excel files into Vue applications. I believe that through continuous learning and practice, we can discover more possibilities in the combination of Vue and Excel, and further improve our development efficiency and user experience.
The above is the detailed content of The elegant combination of Vue and Excel: how to achieve batch processing and export of data. For more information, please follow other related articles on the PHP Chinese website!

WhentheVue.jsVirtualDOMdetectsachange,itupdatestheVirtualDOM,diffsit,andappliesminimalchangestotherealDOM.ThisprocessensureshighperformancebyavoidingunnecessaryDOMmanipulations.

Vue.js' VirtualDOM is both a mirror of the real DOM, and not exactly. 1. Create and update: Vue.js creates a VirtualDOM tree based on component definitions, and updates VirtualDOM first when the state changes. 2. Differences and patching: Comparison of old and new VirtualDOMs through diff operations, and apply only the minimum changes to the real DOM. 3. Efficiency: VirtualDOM allows batch updates, reduces direct DOM operations, and optimizes the rendering process. VirtualDOM is a strategic tool for Vue.js to optimize UI updates.

Vue.js and React each have their own advantages in scalability and maintainability. 1) Vue.js is easy to use and is suitable for small projects. The Composition API improves the maintainability of large projects. 2) React is suitable for large and complex projects, with Hooks and virtual DOM improving performance and maintainability, but the learning curve is steeper.

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
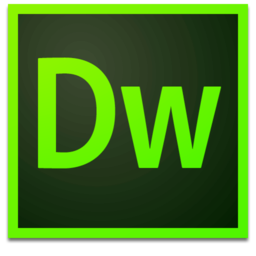
Dreamweaver Mac version
Visual web development tools
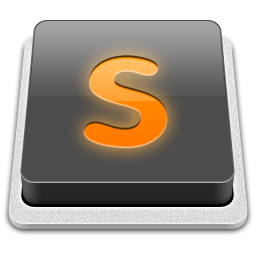
SublimeText3 Mac version
God-level code editing software (SublimeText3)
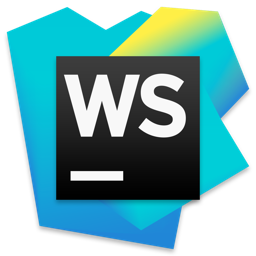
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
