


How to build a highly scalable logging system using PHP and REDIS
How to use PHP and REDIS to build a highly scalable logging system
Introduction:
In modern web application development, the logging system is a very important component, which can record the operation of the system Status, error information, user behavior, etc., to facilitate developers to track and troubleshoot problems. This article will introduce how to use PHP and REDIS to build a highly scalable logging system.
1. What is REDIS?
REDIS is an open source in-memory database that supports a variety of data structures, including strings, hashes, lists, sets, and ordered sets. The characteristic of REDIS is that data is stored in memory, so the reading and writing speed is very fast.
2. Why choose REDIS as log storage?
In traditional logging systems, files or databases are usually used to store log information. However, with the development of Internet applications, the amount of log data is increasing, and traditional storage methods face some challenges, such as slow disk IO speed and difficulty in horizontal expansion. As an in-memory database, REDIS can provide fast read and write speeds and high scalability, and is very suitable for storing log information.
3. Install and configure REDIS
First, you need to install the REDIS server and ensure that it is running normally. You can download the latest version of REDIS from the REDIS official website (https://redis.io/), and then install and configure it according to the official documents.
4. Use PHP to connect to REDIS
In PHP, you can use the Predis library (https://github.com/nrk/predis) to connect to the REDIS server. Predis is a REDIS client implemented in PHP and provides many convenient methods.
First, use the Composer command to install the Predis library:
composer require predis/predis
Then, you can use the following code to connect to the REDIS server:
<?php require "vendor/autoload.php"; // 创建REDIS连接 $client = new PredisClient([ 'scheme' => 'tcp', 'host' => '127.0.0.1', 'port' => 6379, ]); // 检查REDIS是否连接成功 if ($client->connect()) { echo "REDIS连接成功!"; } else { echo "REDIS连接失败!"; }
5. Build the log system
After completion After the installation and configuration of REDIS and the connection of PHP to REDIS, we can start building a highly scalable logging system.
First, we need to define a LOG class to encapsulate log-related operations:
<?php class Log { protected $client; public function __construct($client) { $this->client = $client; } public function write($message, $level = 'info') { $timestamp = time(); $data = [ 'time' => date('Y-m-d H:i:s', $timestamp), 'level' => $level, 'message' => $message, ]; $this->client->rpush('logs', json_encode($data)); } public function read() { $logs = $this->client->lrange('logs', 0, -1); foreach ($logs as $log) { $data[] = json_decode($log, true); } return $data; } }
In the above code, we define a LOG class, which has write() and read( ) two methods. The write() method is used to write logs to a list in the REDIS server, and each log is stored in JSON format; the read() method is used to read all logs from the REDIS server and return them.
Next, we can use the following code to test the function of the log system:
<?php require "vendor/autoload.php"; // 创建REDIS连接 $client = new PredisClient([ 'scheme' => 'tcp', 'host' => '127.0.0.1', 'port' => 6379, ]); // 创建LOG对象 $log = new Log($client); // 写入一条日志 $log->write('This is a test log message.', 'info'); // 读取所有日志 $logs = $log->read(); // 打印日志 foreach ($logs as $log) { echo "{$log['time']} [{$log['level']}] {$log['message']} "; }
Run the above code, the log information will be written to the REDIS database and read out for printing.
6. High scalability
In actual applications, the amount of log data in the log system may be very large. In order to improve the performance and scalability of the system, we can split the log data into multiple REDIS list. For example, log data can be stored in separate lists based on date. When you need to query the logs of a certain date, you only need to read the corresponding REDIS list.
7. Summary
This article introduces how to use PHP and REDIS to build a highly scalable log system. First, we learned about the features and advantages of REDIS; then, we explained how to install and configure REDIS, and use the Predis library to connect to the REDIS server; then, we built a LOG class to encapsulate log-related operations; finally, we Tested the functionality of the logging system. I hope this article will help you understand how to use PHP and REDIS to build a highly scalable logging system.
The above is the detailed content of How to build a highly scalable logging system using PHP and REDIS. For more information, please follow other related articles on the PHP Chinese website!
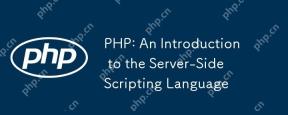
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
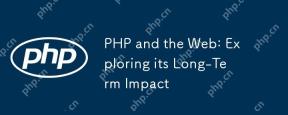
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
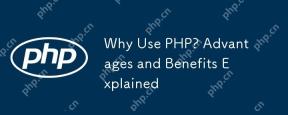
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
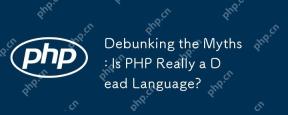
PHP is not dead. 1) The PHP community actively solves performance and security issues, and PHP7.x improves performance. 2) PHP is suitable for modern web development and is widely used in large websites. 3) PHP is easy to learn and the server performs well, but the type system is not as strict as static languages. 4) PHP is still important in the fields of content management and e-commerce, and the ecosystem continues to evolve. 5) Optimize performance through OPcache and APC, and use OOP and design patterns to improve code quality.
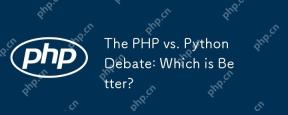
PHP and Python have their own advantages and disadvantages, and the choice depends on the project requirements. 1) PHP is suitable for web development, easy to learn, rich community resources, but the syntax is not modern enough, and performance and security need to be paid attention to. 2) Python is suitable for data science and machine learning, with concise syntax and easy to learn, but there are bottlenecks in execution speed and memory management.
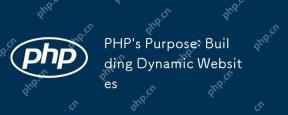
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
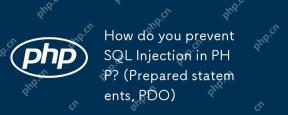
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.