


How to implement website subscription function through PHP and Typecho
How to implement website subscription function through PHP and Typecho
With the rapid development of the Internet, more and more people are beginning to subscribe to their favorite websites in order to get updated content at any time. In order to meet user needs, website administrators need to provide a convenient subscription function. In this article, we will introduce how to use PHP and Typecho to implement the subscription function of the website.
Typecho is a simple and fast open source blog system written in PHP language. It provides a convenient plug-in mechanism that allows developers to easily extend and customize functions. We will use Typecho's plug-in mechanism to implement the subscription function of the website.
First, we need to create a Typecho plug-in to handle the subscription function. In the Typecho plug-in directory, create a new folder named Subscribe and create a file named Subscribe.php in it. The code is as follows:
<?php class Subscribe_Plugin implements Typecho_Plugin_Interface { public static function activate() { // 在这里执行插件激活时的逻辑 } public static function deactivate() { // 在这里执行插件停用时的逻辑 } public static function config(Typecho_Widget_Helper_Form $form) { // 在这里定义插件的配置项 } public static function personalConfig(Typecho_Widget_Helper_Form $form) { // 在这里定义插件的个人配置项 } public static function render() { // 在这里定义插件的前端展示逻辑 } public static function saveConfig() { // 在这里保存插件的配置项 } }
In the above code, we define a plug-in named Subscribe, implement the Typecho_Plugin_Interface interface, and provide methods such as activate, deactivate, config, personalConfig, render and saveConfig. These methods are respectively used for plug-in activation, deactivation, configuration item settings, personal configuration item settings, front-end display and the function of saving configuration items.
Next, we need to add two routes to the plug-in to handle subscription operations. In the activate method of the Subscribe.php file, add the following code:
public static function activate() { Typecho_Plugin::factory('Widget_Feed')->beforeRender = array('Subscribe_Plugin', 'subscribeHandler'); }
The above code registers the subscription handler function subscribeHandler to the beforeRender event of Widget_Feed.
Then, in the render method of the Subscribe.php file, add the following code:
public static function render() { $request = Typecho_Request::getInstance(); if ($request->is('POST')) { self::subscribeHandler(); } }
The above code is used to detect whether the user has submitted a subscription request, and if so, the subscribeHandler processing function is called.
Finally, we need to implement the subscribeHandler processing function. In the Subscribe.php file, add the following code:
public static function subscribeHandler() { $email = Typecho_Widget::widget('Widget_Options')->plugin('Subscribe')->email; $message = '感谢您订阅我们的网站!'; if (!empty($_POST['email'])) { $email = $_POST['email']; // TODO: 将邮箱地址添加到订阅列表中 $message = '订阅成功!'; } else { $message = '请输入有效的邮箱地址!'; } // 输出订阅结果 echo $message; }
The above code first obtains the email address in the plug-in configuration, and then determines whether the user has submitted a valid email address. If so, add the email address to the subscription list, otherwise prompt the user to enter a valid email address and output the subscription result.
So far, we have completed the development of the Typecho plug-in. Next, we need to install the plugin into Typecho for use.
Copy the Subscribe plug-in folder to Typecho's plug-in directory, then log in to Typecho's backend management interface, enter "Console -> Plug-ins", find the Subscribe plug-in and click the "Enable" button.
Finally, we need to add a subscription form to the front-end page of the website. Where you want the subscription form to appear, add the following code:
<form action="<?php echo Typecho_Widget::widget('Widget_Options')->index('/action/subscribe'); ?>" method="post"> <input type="email" name="email" placeholder="请输入您的邮箱地址" required> <input type="submit" value="订阅"> </form>
The above code uses the auxiliary function Typecho_Widget::widget provided by Typecho to output the subscription form, and specifies the form's submission address as Typecho's subscription processing URL.
Through the above steps, we successfully implemented the subscription function of the website using PHP and Typecho. Users can enter their email address on the front-end page to subscribe. The subscription request will be processed through the plug-in and the email address will be added to the subscription list.
The above is the detailed content of How to implement website subscription function through PHP and Typecho. For more information, please follow other related articles on the PHP Chinese website!
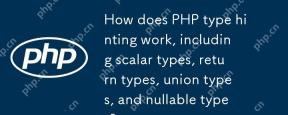
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
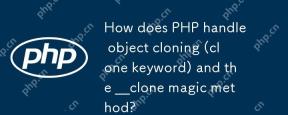
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
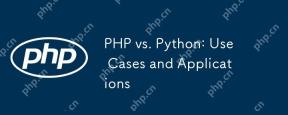
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
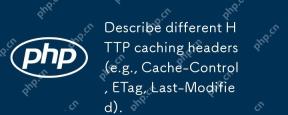
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
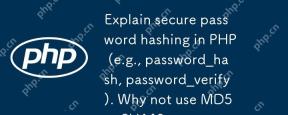
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
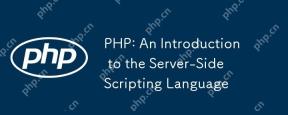
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
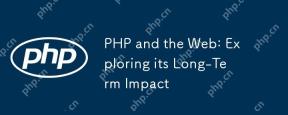
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
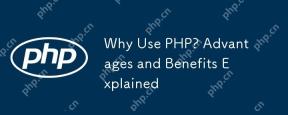
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
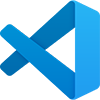
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft