How to use vue's keep-alive for front-end performance optimization
How to use vue’s keep-alive for front-end performance optimization
In modern web applications, front-end performance optimization is a crucial task. An efficient front-end application can not only improve user experience, but also save server resources and bandwidth. In Vue.js, using keep-alive components to optimize page performance is a common method.
Vue.js is a popular JavaScript framework for building user interfaces. It provides a component called keep-alive that caches rendered component instances in order to preserve their state when switching or avoid re-rendering. This can provide a great performance improvement for components that need to be switched frequently, such as lists, tabs, etc.
Below we will demonstrate how to use Vue's keep-alive component to optimize the performance of a simple list component.
First, we create a new component called List.vue to display a simple list.
<template> <div> <ul> <li v-for="item in list">{{ item }}</li> </ul> </div> </template> <script> export default { data() { return { list: ['item 1', 'item 2', 'item 3'] }; } }; </script>
In this component, we use the v-for directive to render each item in the list array as a li element.
Next, we use the keep-alive component in the parent component to cache instances of the List component.
<template> <div> <button @click="toggleList">Toggle List</button> <keep-alive> <List v-if="showList" key="list" /> </keep-alive> </div> </template> <script> import List from './List.vue'; export default { components: { List }, data() { return { showList: true }; }, methods: { toggleList() { this.showList = !this.showList; } } }; </script>
In the parent component, we added a button to toggle the display state of the list. When the button is clicked, we show or hide the list by modifying the value of showList. However, since the List component is wrapped in a keep-alive component, even if the list is hidden, its instance will still be retained in memory instead of being destroyed and recreated.
The advantage of this is that when we display the list again, it will retain its previous state without the need to re-render. This is useful for large lists or components that require complex calculations.
In addition to retaining state when switching components, keep-alive also provides some other life cycle hook functions. For example, we can use activated hooks to perform some actions when the component is activated.
<template> <div> <!-- ... --> </div> </template> <script> export default { activated() { console.log('List component activated'); } }; </script>
In this example, when the List component is displayed, a message will be printed to the console. This is useful for scenarios where you need to load data or perform other operations while the component is displayed.
To summarize, using Vue’s keep-alive component can significantly improve the performance of front-end applications. It avoids unnecessary re-rendering by caching component instances and provides multiple lifecycle hook functions to perform specific actions when needed.
When dealing with large lists or components that require frequent switching, using keep-alive is a very effective performance optimization method. I hope this article will help you use Vue.js for performance optimization in front-end development.
The above is the detailed content of How to use vue's keep-alive for front-end performance optimization. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is loved by developers because it is easy to use and powerful. 1) Its responsive data binding system automatically updates the view. 2) The component system improves the reusability and maintainability of the code. 3) Computing properties and listeners enhance the readability and performance of the code. 4) Using VueDevtools and checking for console errors are common debugging techniques. 5) Performance optimization includes the use of key attributes, computed attributes and keep-alive components. 6) Best practices include clear component naming, the use of single-file components and the rational use of life cycle hooks.

Vue.js is a progressive JavaScript framework suitable for building efficient and maintainable front-end applications. Its key features include: 1. Responsive data binding, 2. Component development, 3. Virtual DOM. Through these features, Vue.js simplifies the development process, improves application performance and maintainability, making it very popular in modern web development.

Vue.js and React each have their own advantages and disadvantages, and the choice depends on project requirements and team conditions. 1) Vue.js is suitable for small projects and beginners because of its simplicity and easy to use; 2) React is suitable for large projects and complex UIs because of its rich ecosystem and component design.

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.

Vue.js' role in web development is to act as a progressive JavaScript framework that simplifies the development process and improves efficiency. 1) It enables developers to focus on business logic through responsive data binding and component development. 2) The working principle of Vue.js relies on responsive systems and virtual DOM to optimize performance. 3) In actual projects, it is common practice to use Vuex to manage global state and optimize data responsiveness.

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
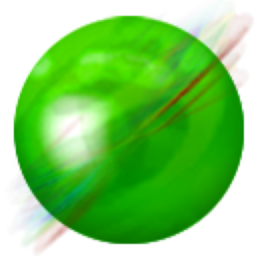
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
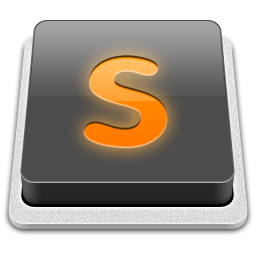
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
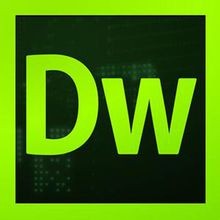
Dreamweaver CS6
Visual web development tools