How to use Vue and Excel to automatically filter and export data
With the advent of the Internet and big data era, data analysis and export have become an important task. As a common data processing tool, Excel is widely used. This article will introduce how to use Vue and Excel to realize automatic filtering and export of data, and attach code examples. Hope it can be helpful to readers.
First, we need to introduce the related libraries of Vue and Excel. In a Vue project, you can use npm or yarn to install these libraries. The specific operations are as follows:
// 安装vue和vue-router库 npm install vue npm install vue-router // 安装exceljs库 npm install exceljs
After the installation is completed, introduce these libraries into the Vue entry file (usually main.js):
import Vue from 'vue' import VueRouter from 'vue-router' import ExcelJS from 'exceljs' Vue.use(VueRouter) Vue.prototype.$ExcelJS = ExcelJS
Next, we need to create a page to display the data , and implement data filtering and export functions. You can create a new component named DataExport.vue and implement related functions in this component. First, we need to add a table for displaying data to the template:
<template> <div> <table> <thead> <tr> <th>姓名</th> <th>性别</th> <th>年龄</th> </tr> </thead> <tbody> <tr v-for="item in filteredData" :key="item.id"> <td>{{ item.name }}</td> <td>{{ item.sex }}</td> <td>{{ item.age }}</td> </tr> </tbody> </table> <button @click="exportData">导出数据</button> </div> </template>
Then, in the Script part, we need to define the data and filtering methods:
<script> export default { data() { return { data: [ { id: 1, name: '张三', sex: '男', age: 25 }, { id: 2, name: '李四', sex: '女', age: 30 }, { id: 3, name: '王五', sex: '男', age: 28 }, // 此处省略其他数据 ], filter: { name: '', sex: '', age: '' } } }, computed: { filteredData() { let filteredData = this.data if (this.filter.name) { filteredData = filteredData.filter(item => item.name.includes(this.filter.name)) } if (this.filter.sex) { filteredData = filteredData.filter(item => item.sex === this.filter.sex) } if (this.filter.age) { filteredData = filteredData.filter(item => item.age === parseInt(this.filter.age)) } return filteredData } }, methods: { exportData() { const workbook = new this.$ExcelJS.Workbook() const worksheet = workbook.addWorksheet('数据') // 添加表头 worksheet.addRow(['姓名', '性别', '年龄']) // 添加数据 this.filteredData.forEach(item => { worksheet.addRow([item.name, item.sex, item.age]) }) // 导出Excel文件 workbook.xlsx.writeBuffer().then(buffer => { const blob = new Blob([buffer], { type: 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet' }) const url = URL.createObjectURL(blob) const link = document.createElement('a') link.href = url link.download = '数据导出.xlsx' link.click() }) } } } </script>
In the above code, we define A data array containing some data. The filter object is used to store filter conditions entered by the user. Automatic filtering of data is achieved through the computed property filteredData. In the exportData method, use the ExcelJS library to create an Excel workbook, import the filtered data into the worksheet, and then export the Excel file.
Finally, introduce and use the DataExport component in the page that uses this component:
<template> <div> <!-- 此处省略其他内容 --> <DataExport/> </div> </template> <script> import DataExport from './DataExport.vue' export default { components: { DataExport }, // 此处省略其他代码 } </script>
At this point, we have completed the automatic filtering and exporting of data using Vue and Excel. Readers can adjust and optimize the code according to their actual needs. I hope this article can be helpful to everyone, thank you for reading!
The above is the detailed content of How to use Vue and Excel to automatically filter and export data. For more information, please follow other related articles on the PHP Chinese website!
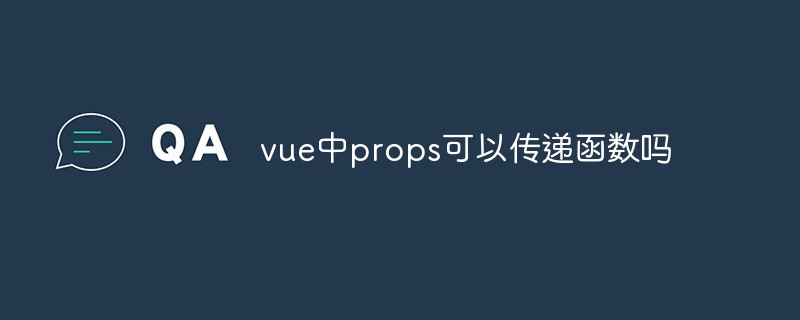
vue中props可以传递函数;vue中可以将字符串、数组、数字和对象作为props传递,props主要用于组件的传值,目的为了接收外面传过来的数据,语法为“export default {methods: {myFunction() {// ...}}};”。
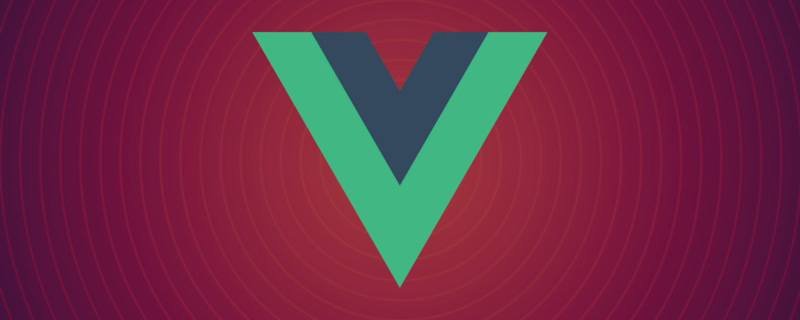
本篇文章带大家聊聊vue指令中的修饰符,对比一下vue中的指令修饰符和dom事件中的event对象,介绍一下常用的事件修饰符,希望对大家有所帮助!
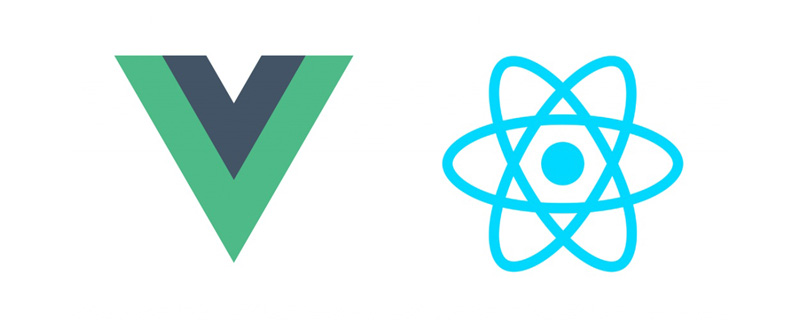
如何覆盖组件库样式?下面本篇文章给大家介绍一下React和Vue项目中优雅地覆盖组件库样式的方法,希望对大家有所帮助!
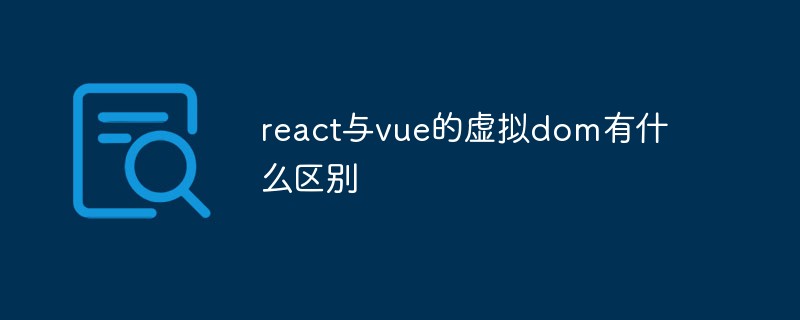
react与vue的虚拟dom没有区别;react和vue的虚拟dom都是用js对象来模拟真实DOM,用虚拟DOM的diff来最小化更新真实DOM,可以减小不必要的性能损耗,按颗粒度分为不同的类型比较同层级dom节点,进行增、删、移的操作。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
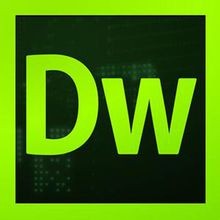
Dreamweaver CS6
Visual web development tools
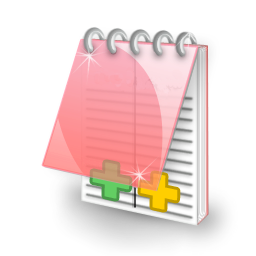
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
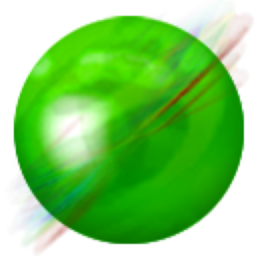
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
