


How does PHP ZipArchive check the compression ratio of files in a compressed package?
PHP ZipArchive is a class used to create and decompress ZIP files. In applications, sometimes we need to know the compression ratio of each file in the compressed package to understand the compression effect and performance. This article will introduce how to use the PHP ZipArchive class to view the compression rate of files in a compressed package.
First, we need to create a ZipArchive object and open a ZIP file. The code is as follows:
$zip = new ZipArchive; $zipFile = 'path/to/archive.zip'; if ($zip->open($zipFile) === true) { // 在这里实现对压缩包文件压缩率的查看 } else { echo '无法打开ZIP文件'; }
Next, we can iterate through all the files in the compressed package and calculate the compression ratio by getting the pre-compression and post-compression sizes of each file. The code is as follows:
$totalSizeBefore = 0; $totalSizeAfter = 0; for ($i = 0; $i < $zip->numFiles; $i++) { $fileName = $zip->getNameIndex($i); $stat = $zip->statIndex($i); $sizeBefore = $stat['size']; $sizeAfter = $stat['comp_size']; $totalSizeBefore += $sizeBefore; $totalSizeAfter += $sizeAfter; $compressionRate = ($sizeBefore - $sizeAfter) / $sizeBefore * 100; // 计算压缩率 echo "文件名:{$fileName}<br/>"; echo "压缩前大小:{$sizeBefore} bytes<br/>"; echo "压缩后大小:{$sizeAfter} bytes<br/>"; echo "压缩率:{$compressionRate}%<br/>"; echo "<br/>"; } $averageCompressionRate = ($totalSizeBefore - $totalSizeAfter) / $totalSizeBefore * 100 / $zip->numFiles; // 计算平均压缩率 echo "总压缩前大小:{$totalSizeBefore} bytes<br/>"; echo "总压缩后大小:{$totalSizeAfter} bytes<br/>"; echo "平均压缩率:{$averageCompressionRate}%<br/>"; $zip->close();
The above code first defines the $totalSizeBefore and $totalSizeAfter variables, which are used to calculate the total size of all files before and after compression. Then it loops through all the files in the compressed package, obtains the size before and after compression of each file, and calculates the compression ratio. Finally, the average compression ratio of all files is calculated and the total pre- and post-compression size is output.
Note that the above code is only suitable for obtaining the file compression ratio in an existing ZIP file. If you want to get the compression ratio of the file when creating the ZIP file, you can use the file_get_contents function to get the contents of the file before adding the file to the ZIP file, and then call the gzcompress function to compress, calculate the size before and after compression and calculate the compression ratio.
In general, by using the methods provided by the PHP ZipArchive class, we can easily view the compression ratio of the files in the compressed package. This feature not only helps to understand the compression effect of the compressed package, but also evaluates the compression performance and optimizes the compression algorithm.
The above is the detailed content of How does PHP ZipArchive check the compression ratio of files in a compressed package?. For more information, please follow other related articles on the PHP Chinese website!
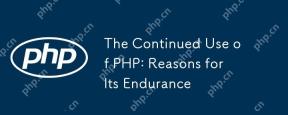
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
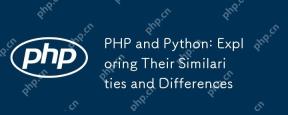
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
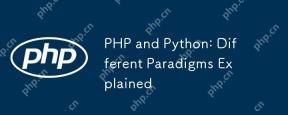
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
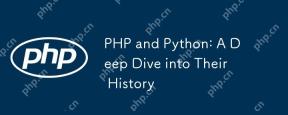
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
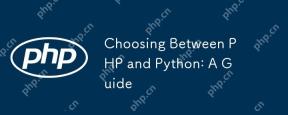
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
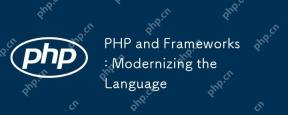
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
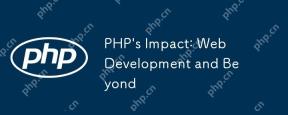
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
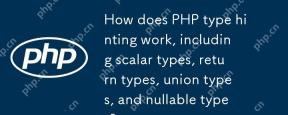
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
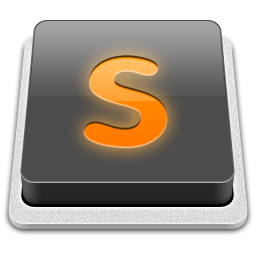
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor