How to use vue and Element-plus to implement data statistics and analysis
How to use Vue and Element Plus to implement data statistics and analysis
Introduction:
In the modern data-driven society, data analysis and statistics have become very important tasks. In order to analyze data and display statistical results more effectively, front-end developers can use the Vue framework and Element Plus component library to implement data statistics and analysis functions. This article will introduce how to use Vue and Element Plus to implement data statistics and analysis, and provide relevant code examples.
1. Preparation
First, make sure you have installed Vue and Element Plus. Use Vue CLI to quickly build a Vue project and install Element Plus.
# 安装Vue CLI npm install -g @vue/cli # 创建一个Vue项目 vue create data-analysis # 进入项目目录 cd data-analysis # 安装Element Plus npm install element-plus@next --save
2. Data preparation
Before performing data statistics and analysis, you need to prepare the corresponding data. Data can be obtained from the backend API or fake data can be used. For convenience, we use fake data in this article.
// data.json { "users": [ { "id": 1, "name": "张三", "age": 20, "gender": "男" }, { "id": 2, "name": "李四", "age": 25, "gender": "女" }, { "id": 3, "name": "王五", "age": 30, "gender": "男" } ] }
3. Data display
Use the table component of Element Plus to display data conveniently. In the Vue component, import the corresponding component and use data binding to display the data in the table.
<template> <el-table :data="users" style="width: 100%"> <el-table-column prop="name" label="姓名"></el-table-column> <el-table-column prop="age" label="年龄"></el-table-column> <el-table-column prop="gender" label="性别"></el-table-column> </el-table> </template> <script> export default { data() { return { users: [] }; }, mounted() { // 获取数据 this.users = require("./data.json").users; } }; </script>
4. Data statistics
In the Vue component, you can use calculated properties to count data, such as calculating the number of users, average age, etc.
<template> <div> <h1 id="用户统计">用户统计</h1> <p>用户人数:{{ userCount }}</p> <p>平均年龄:{{ avgAge }}</p> </div> </template> <script> export default { data() { return { users: [] }; }, computed: { userCount() { return this.users.length; }, avgAge() { let totalAge = 0; for (let user of this.users) { totalAge += user.age; } return totalAge / this.users.length; } }, mounted() { // 获取数据 this.users = require("./data.json").users; } }; </script>
5. Data Analysis
In addition to basic statistical data, you can use Element Plus’ chart component to analyze and display data. You need to install vue-echarts and echarts plug-ins first.
npm install vue-echarts echarts --save
In the Vue component, import the corresponding component and use data binding to display the data in the chart.
<template> <el-card> <div style="height: 400px"> <chart :options="chartOptions" /> </div> </el-card> </template> <script> import { reactive } from "vue"; import Chart from "vue-echarts"; export default { components: { Chart }, data() { return { users: [], chartOptions: reactive({ xAxis: { type: "category", data: [] }, yAxis: { type: "value" }, series: [ { data: [], type: "bar" } ] }) }; }, mounted() { // 获取数据 this.users = require("./data.json").users; // 统计性别人数 let genders = {}; for (let user of this.users) { if (genders[user.gender]) { genders[user.gender]++; } else { genders[user.gender] = 1; } } this.chartOptions.xAxis.data = Object.keys(genders); this.chartOptions.series[0].data = Object.values(genders); } }; </script>
6. Summary
Using Vue and Element Plus can easily implement data statistics and analysis functions. In this article, we explain how to display data, statistics, and analytics, and provide relevant code examples. I hope this article can help you implement data statistics and analysis functions in your Vue project.
The above is the detailed content of How to use vue and Element-plus to implement data statistics and analysis. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive JavaScript framework suitable for building efficient and maintainable front-end applications. Its key features include: 1. Responsive data binding, 2. Component development, 3. Virtual DOM. Through these features, Vue.js simplifies the development process, improves application performance and maintainability, making it very popular in modern web development.

Vue.js and React each have their own advantages and disadvantages, and the choice depends on project requirements and team conditions. 1) Vue.js is suitable for small projects and beginners because of its simplicity and easy to use; 2) React is suitable for large projects and complex UIs because of its rich ecosystem and component design.

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.

Vue.js' role in web development is to act as a progressive JavaScript framework that simplifies the development process and improves efficiency. 1) It enables developers to focus on business logic through responsive data binding and component development. 2) The working principle of Vue.js relies on responsive systems and virtual DOM to optimize performance. 3) In actual projects, it is common practice to use Vuex to manage global state and optimize data responsiveness.

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
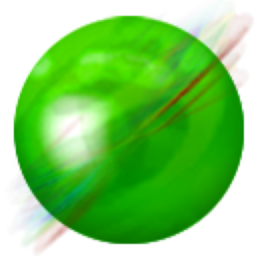
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
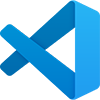
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment