How to optimize memory usage in Vue applications
How to optimize memory usage in Vue applications
With the popularity of Vue, more and more developers are beginning to use Vue to build applications. However, in larger Vue applications, memory usage can become an issue due to DOM manipulation and Vue's reactive system. This article will introduce some tips and suggestions on how to optimize memory usage in Vue applications.
- Reasonable use of v-if and v-for
It is very common to use v-if and v-for directives in Vue applications. However, excessive use of these two instructions may result in excessive memory usage. Therefore, you need to pay attention to the following points when using it:
- Use v-if instead of v-show: v-show only controls the display and hiding of elements through CSS, rather than actually deleting and creating the DOM. element. Therefore, when a component is no longer needed, it should be completely removed from the DOM using v-if to free up memory.
- Use the key attribute appropriately: When using v-for, add a unique key attribute to each list item. Vue tracks the changes of each list item through the key attribute. If the key attribute is not provided, Vue will use an unpredictable way to handle old nodes, which may lead to high memory usage.
The following is a sample code:
<template> <div> <div v-if="showHello">Hello</div> <ul> <li v-for="item in items" :key="item.id">{{ item.name }}</li> </ul> </div> </template> <script> export default { data() { return { showHello: true, items: [ { id: 1, name: "item 1" }, { id: 2, name: "item 2" }, // ... ], }; }, }; </script>
- Destroy the component in time
In Vue, the life cycle of the component is managed by the Vue instance. When a component is no longer needed, you should ensure that it is destroyed promptly to free up memory.
When destroying a component, you need to pay attention to the following points:
- Manually unbind the event listener: If a component listens to events of other components or DOM, when the component is destroyed, These event listeners need to be manually unbound to prevent memory leaks.
- Cancel requests and clean up timers: If a component sends asynchronous requests or sets timers, when the component is destroyed, these requests should be canceled and the timers cleaned up to prevent invalid network requests and memory usage.
The following is a sample code:
<template> <div> <Button v-if="showButton" @click="onClick">Click me</Button> <!-- ... --> </div> </template> <script> import Button from "@/components/Button.vue"; export default { data() { return { showButton: true, }; }, methods: { onClick() { // 处理点击事件 }, }, beforeDestroy() { // 手动解绑事件监听器、取消请求和清理定时器 }, components: { Button, }, }; </script>
- Using lazy loading and asynchronous components
In large Vue applications, the page may Contains many components, loading all of them may result in high initial load times and memory usage. Therefore, you can use lazy loading and asynchronous components to load components on demand.
In Vue, lazy loading can be achieved through the dynamic import of Vue Router and the dynamic import function of Webpack. Using lazy loading and asynchronous components can split the code and load the corresponding components only when needed, thereby reducing initial loading time and memory usage.
The following is a sample code:
const Home = () => import("@/components/Home.vue"); const About = () => import("@/components/About.vue"); const Contact = () => import("@/components/Contact.vue"); const routes = [ { path: "/", component: Home }, { path: "/about", component: About }, { path: "/contact", component: Contact }, // ... ];
- Performance analysis using Vue Devtools
Vue Devtools is a browser extension tool for Vue debugging . It provides a series of functions, including component hierarchy tree, Vue instance, event tracking, etc. Using Vue Devtools can help us view and analyze the memory and performance of the application, and find possible memory leaks and performance bottlenecks.
You can get Vue Devtools through the Chrome browser extension store or by visiting the official website of Vue Devtools.
To sum up, by using v-if and v-for properly, destroying components in time, using lazy loading and asynchronous components, and using Vue Devtools for performance analysis, we can optimize memory usage in Vue applications. These tips and suggestions will help us build more efficient and stable Vue applications.
(Note: The above code examples are for reference only, and the specific implementation may change according to the needs of the project and the technology stack.)
The above is the detailed content of How to optimize memory usage in Vue applications. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
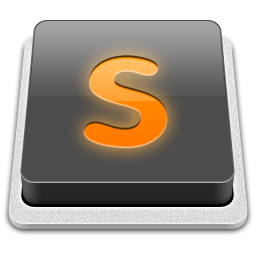
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
