What are the data transfer methods for Vue component communication?
In Vue development, component communication is a very important part. Through component communication, data transfer and interaction between different components can be achieved. Vue provides a variety of ways to implement component communication, including props, emit, provide/inject, Vuex, etc. This article explains these different data transfer methods and provides corresponding code examples.
- props and $emit
props are used by the parent component to pass data to the child component, and the child component receives the passed data through props. $emit is used by the child component to pass data to the parent component. The child component triggers events and passes data to the parent component through $emit.
<child-component :message="message" @update="updateMessage"></child-component>
<p>父组件收到子组件传递过来的数据:{{message}}</p>
<script><br> import ChildComponent from './ChildComponent'</script>
export default {
components: { ChildComponent }, data() { return { message: '' } }, methods: { updateMessage(newMessage) { this.message = newMessage } }
}
<input type="text" v-model="message" />
<button @click="sendMessage">传递数据给父组件</button>
<script><br> export default {</script>
data() { return { message: '' } }, methods: { sendMessage() { this.$emit('update', this.message) } }
}
script>
- provide/inject
provide and inject are a pair of options for passing data across hierarchical components. Data is provided through the parent component and data is injected into the child component. accomplish. The provide option provides data, and the inject option injects data.
<p>父组件提供数据:{{message}}</p>
<child-component></child-component>
<script><br> import ChildComponent from './ChildComponent'</script>
export default {
components: { ChildComponent }, provide() { return { message: 'Hello World!' } }
}
<p>子组件注入数据:{{message}}</p>
<script><br> export default {</script>
inject: ['message']
}
script>
- Vuex
Vuex is the state management mode officially provided by Vue, which is used to centrally manage data shared between components. Data transfer and interaction between components are realized through Vuex's state, getters, mutations, actions, etc.
// store.js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
message: 'Hello World!'
},
getters: {
getMessage: state => state.message
},
mutations: {
updateMessage(state, newMessage) { state.message = newMessage }
},
actions: {
changeMessage({ commit }, payload) { commit('updateMessage', payload) }
}
})
// ParentComponent.vue
<child-component></child-component>
<p>父组件获取数据:{{message}}</p>
<button @click="changeMessage">更改数据</button>
<script><br> import ChildComponent from './ChildComponent'<br> import { mapGetters, mapActions } from 'vuex'</script>
export default {
components: { ChildComponent }, computed: { ...mapGetters(['getMessage']), message() { return this.getMessage } }, methods: { ...mapActions(['changeMessage']) }
}
// ChildComponent.vue
<p>子组件获取数据:{{message}}</p>
<script><br> import { mapGetters } from 'vuex'</script>
export default {
computed: { ...mapGetters(['getMessage']), message() { return this.getMessage } }
}
The above are several common data transfer methods for Vue component communication. Each method has its own applicable scenarios. Choose the appropriate one according to actual needs. method for data transfer. By rationally using these methods, flexible and efficient communication between components can be achieved, improving development efficiency and code quality.
The above is the detailed content of What are the data transfer methods for Vue component communication?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is loved by developers because it is easy to use and powerful. 1) Its responsive data binding system automatically updates the view. 2) The component system improves the reusability and maintainability of the code. 3) Computing properties and listeners enhance the readability and performance of the code. 4) Using VueDevtools and checking for console errors are common debugging techniques. 5) Performance optimization includes the use of key attributes, computed attributes and keep-alive components. 6) Best practices include clear component naming, the use of single-file components and the rational use of life cycle hooks.

Vue.js is a progressive JavaScript framework suitable for building efficient and maintainable front-end applications. Its key features include: 1. Responsive data binding, 2. Component development, 3. Virtual DOM. Through these features, Vue.js simplifies the development process, improves application performance and maintainability, making it very popular in modern web development.

Vue.js and React each have their own advantages and disadvantages, and the choice depends on project requirements and team conditions. 1) Vue.js is suitable for small projects and beginners because of its simplicity and easy to use; 2) React is suitable for large projects and complex UIs because of its rich ecosystem and component design.

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.

Vue.js' role in web development is to act as a progressive JavaScript framework that simplifies the development process and improves efficiency. 1) It enables developers to focus on business logic through responsive data binding and component development. 2) The working principle of Vue.js relies on responsive systems and virtual DOM to optimize performance. 3) In actual projects, it is common practice to use Vuex to manage global state and optimize data responsiveness.

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
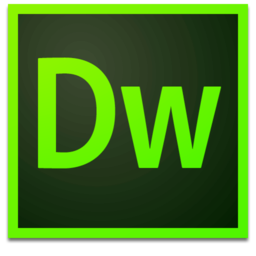
Dreamweaver Mac version
Visual web development tools
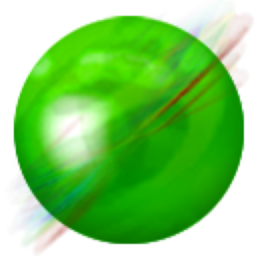
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software