Vue and Canvas: How to achieve gorgeous animation effects
Introduction:
In Web development, animation effects are one of the important factors that are pleasing to the eye. Vue is a popular JavaScript framework, while Canvas is an HTML element used for drawing graphics and animations. This article will introduce how to combine Vue and Canvas to achieve gorgeous animation effects, and provide code examples for readers' reference.
-
New Vue project
First, we need to create a Vue project. Run the following command in the command line:vue create vue-canvas-animation
Then, select the default configuration to complete the project creation process. Enter the project directory and start the development server:
cd vue-canvas-animation npm run serve
By accessing http://localhost:8080 in the browser, you will see the initial interface of the Vue project.
-
Add Canvas element
In the src directory of the Vue project, create a new component file CanvasAnimation.vue. In this file, we will use the Canvas element to achieve animation effects. In the template tag, add the following code:<template> <div> <canvas ref="canvas"></canvas> </div> </template>
This code will create an empty Canvas element. Through the ref attribute, we can reference the element in the Vue component.
- Using Vue's life cycle hooks and Canvas API
In the Vue component, we can use the life cycle hook function to control the rendering and destruction of the page. Here, we will use the created hook function to initialize the Canvas and draw the animation in the mounted hook function.
First, we import the CanvasAnimation.vue file in the <script> tag and add the following code: </script>
<script> import { onMounted, reactive } from 'vue'; export default { name: 'CanvasAnimation', setup() { const canvasRef = reactive(null); // 用于引用Canvas元素的响应式数据 onMounted(() => { const canvas = canvasRef.value; const ctx = canvas.getContext('2d'); // 获取Canvas的2D绘图上下文 // 编写绘制动画的代码 // ... }); return { canvasRef, }; }, }; </script>
In this example, we use the Composition API of Vue 3 , the reactive function declares canvasRef as responsive data. Then, in the onMounted hook function, we obtain the context object ctx of the Canvas for subsequent drawing animation.
- Drawing animation
In the previous step, we have obtained the context object ctx of Canvas. Next, we can use the Canvas API to draw animations.
In the onMounted hook function, add the following code:
const drawAnimation = () => { requestAnimationFrame(drawAnimation); // 循环调用绘制函数,实现动画效果 // 在这里编写绘制动画的代码 }; drawAnimation(); // 调用绘制函数,启动动画
Through the requestAnimationFrame function, we can loop the drawing function to achieve animation effects. In the drawing function, we can use the methods of the ctx object to draw graphics, change styles and handle animation logic.
Here is a simple sample code for drawing a moving ball:
const drawAnimation = () => { requestAnimationFrame(drawAnimation); ctx.clearRect(0, 0, canvas.width, canvas.height); // 清除画布 ctx.beginPath(); ctx.arc(ball.x, ball.y, ball.radius, 0, Math.PI * 2); // 绘制小球 ctx.fillStyle = ball.color; ctx.fill(); // 移动小球 ball.x += ball.speedX; ball.y += ball.speedY; // 碰撞检测 if (ball.x + ball.radius > canvas.width || ball.x - ball.radius < 0) { ball.speedX *= -1; } if (ball.y + ball.radius > canvas.height || ball.y - ball.radius < 0) { ball.speedY *= -1; } }; const ball = { x: 100, y: 100, radius: 20, speedX: 2, speedY: 1, color: '#ff0000', };
The above is the detailed content of Vue and Canvas: How to achieve gorgeous animation effects. For more information, please follow other related articles on the PHP Chinese website!
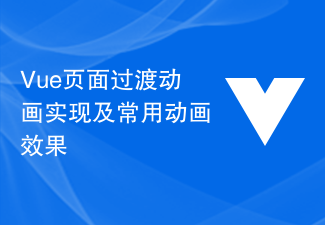
Vue是一款流行的JavaScript框架,它通过数据驱动的方式,协助开发者构建交互性强、数据呈现美观的单页Web应用。Vue内置了许多有用的特性,其中之一就是页面过渡动画。在本文中,我们将介绍如何使用Vue的过渡动画功能,并且讨论最常见的动画效果。实现Vue页面过渡动画Vue的页面过渡动画是通过Vue的<transition>与<tr
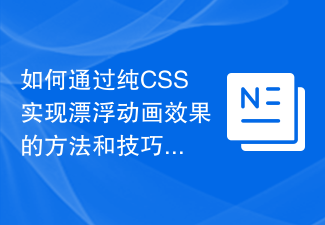
如何通过纯CSS实现漂浮动画效果的方法和技巧在现代网页设计中,动画效果已成为吸引用户眼球的重要元素之一。而其中一种常见的动画效果就是漂浮效果,它可以给网页增加一种动感和活力,使得用户体验更加丰富和有趣。本文将介绍如何通过纯CSS实现漂浮动画效果,并提供一些代码示例供参考。一、使用CSS的transition属性来实现漂浮效果CSS的transition属性可
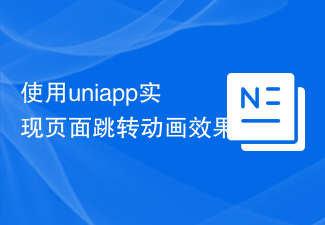
标题:使用uniapp实现页面跳转动画效果近年来,移动应用的用户界面设计已经成为吸引用户的重要因素之一。页面跳转动画效果在提升用户体验和可视化效果方面起着重要的作用。本文将介绍如何使用uniapp实现页面跳转动画效果,并提供具体的代码示例。uniapp是一个基于Vue.js开发的跨平台应用开发框架,可以通过一套代码编译生成小程序、H5、App等多个平台的应用
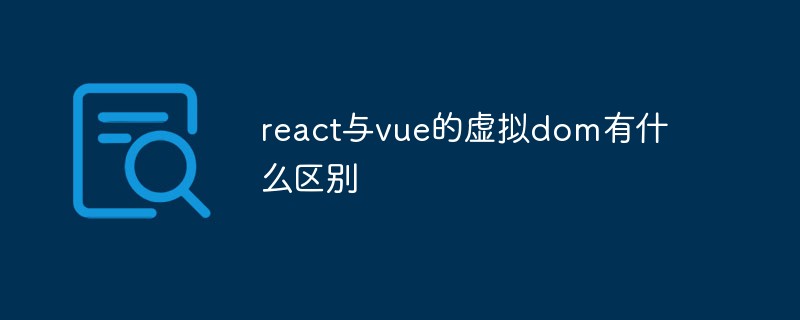
react与vue的虚拟dom没有区别;react和vue的虚拟dom都是用js对象来模拟真实DOM,用虚拟DOM的diff来最小化更新真实DOM,可以减小不必要的性能损耗,按颗粒度分为不同的类型比较同层级dom节点,进行增、删、移的操作。
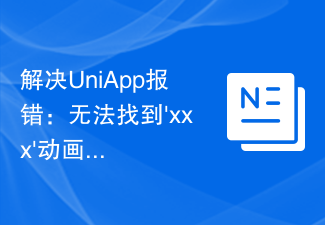
解决UniApp报错:无法找到'xxx'动画效果的问题UniApp是一种基于Vue.js框架的跨平台应用开发框架,可以用于开发微信小程序、H5、App等多个平台的应用。在开发过程中,我们经常会使用到动画效果来提升用户体验。然而,有时候会遇到一个报错:无法找到'xxx'动画效果。这个报错会导致动画无法正常运行,给开发带来不便。本文将介绍几种解决这个问题的方法。

在VSCode中开发Vue/React组件时,怎么实时预览组件?本篇文章就给大家分享一个VSCode 中实时预览Vue/React组件的插件,希望对大家有所帮助!
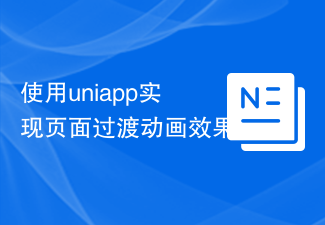
随着移动互联网的快速发展,越来越多的程序员开始使用uniapp构建跨平台应用。在移动应用开发中,页面过渡动画对用户体验升级起着非常重要的作用。通过页面过渡动画,能够有效地增强用户体验,提高用户留存率和满意度。因此,下面就来分享一下如何使用uniapp实现页面过渡动画效果,同时提供具体代码示例。一、uniapp介绍uniapp是DCloud开发团队推出的一款基
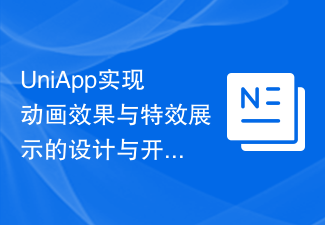
UniApp实现动画效果与特效展示的设计与开发指南一、引言UniApp是一个基于Vue.js的跨平台开发框架,它能够帮助开发者快速、高效地开发出适配多个平台的应用程序。在移动应用开发中,动画效果和特效展示往往能够增强用户体验,提升应用的吸引力。本文将介绍如何在UniApp中实现动画效果与特效展示。二、动画效果的实现在UniApp中,可以使用全局动画库uni-


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
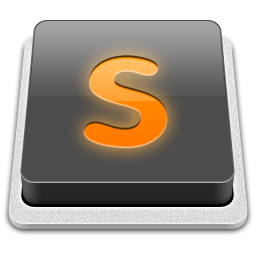
SublimeText3 Mac version
God-level code editing software (SublimeText3)
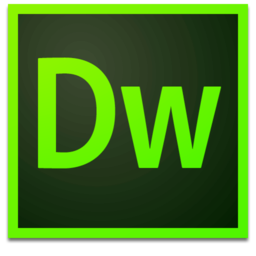
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use
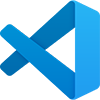
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version
