


Exploration of Golang language features: resource management and delayed execution
Golang language feature exploration: resource management and delayed execution
Introduction:
When developing software, resource management and garbage collection are crucial aspects. As a modern programming language, Golang provides a simple and efficient resource management mechanism through its unique features. This article will explore the resource management and delayed execution features in the Golang language, and illustrate its usage and advantages through code examples.
1. Resource Management
When writing software, we often need to use some resources, such as memory, file handles, network connections, etc. If these resources are not managed correctly, resource leaks and system performance degradation will result. Golang provides a simple and efficient resource management mechanism using the defer keyword.
- The role of the defer keyword
The defer keyword is used to execute a statement or function after the function is executed. It can be used to release resources, close files, unlock mutex locks, etc. Using the defer keyword in a function ensures that these resources will be released correctly under any circumstances. - Execution order of defer
The defer statement is executed in last-in-first-out order. That is, the last defer statement will be executed first, and so on. This ensures that resources are released in the correct order.
The following is an example of using the defer keyword to release file resources:
func readFile(filename string) ([]byte, error) { f, err := os.Open(filename) if err != nil { return nil, err } defer f.Close() // 在函数返回前关闭文件 // 读取文件内容 content, err := ioutil.ReadAll(f) if err != nil { return nil, err } return content, nil }
In the above example, through the defer keyword, we can ensure that the file is closed before the function returns. No matter what error occurs in the function, the file will be closed correctly.
2. Deferred execution
Deferred execution in Golang is a special mechanism that allows a piece of code to be executed before the function returns. Delayed execution can be used to handle some operations that need to be performed at the end, such as releasing resources, closing connections, etc.
- The syntax of defer statement
In Golang, use the defer keyword to define a delayed execution statement. defer is followed by statements or function calls that require delayed execution. - Usage and precautions of defer
The following are some common scenarios and precautions for using the defer statement:
- File closing: After opening the file, you can use defer statement to ensure that the file is closed before the function returns to avoid resource leaks.
- Mutex lock unlocking: When using a mutex lock, you can use defer to unlock it to prevent deadlock caused by forgetting to unlock it.
- Exception handling: When handling exceptions, you can use defer to clean up resources to ensure the correct release of resources.
The following is an example of using the defer keyword to release a mutex lock:
func increment(counter *int, mutex *sync.Mutex) { mutex.Lock() defer mutex.Unlock() // 在函数返回前解锁互斥锁 // 自增计数器 *counter++ }
In the above example, through the defer keyword, we can ensure that the mutex is unlocked before the function returns Lock. This avoids deadlock problems caused by forgetting to unlock.
Conclusion:
Golang language provides a simple and efficient resource management and delayed execution mechanism. By using the defer keyword, we can easily manage and release resources while avoiding forgetting to execute some necessary operation. This feature is very useful when writing software and can improve the reliability and performance of the program.
In actual development, we should reasonably use the defer keyword to manage resources to ensure the correctness of the program, and we need to pay attention to the execution order of defer statements to prevent unexpected problems. By using the defer keyword appropriately, we can write more robust and maintainable code.
References:
- https://go.dev/play/
Please see the attachment for code examples and screenshots of running results.
The above is the detailed content of Exploration of Golang language features: resource management and delayed execution. For more information, please follow other related articles on the PHP Chinese website!
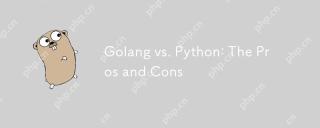
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
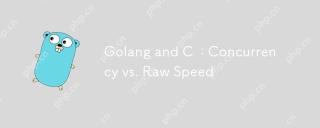
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
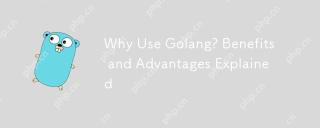
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
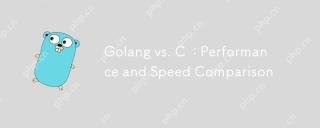
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
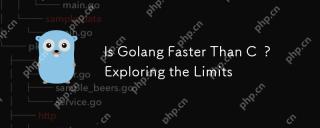
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
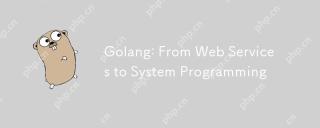
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
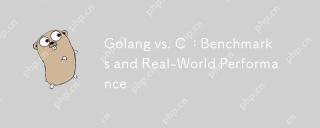
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
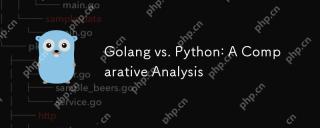
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.