


Vue and Canvas: How to implement image beautification and skin resurfacing functions
Vue and Canvas: How to implement the beautification and dermabrasion functions of pictures
In recent years, the beautification and dermabrasion functions have become standard functions of many mobile phone camera applications. These functions not only make users more confident when taking selfie photos, but also improve the quality of the pictures to a certain extent. This article will introduce how to use Vue and Canvas technology to realize the beautification and skin resurfacing functions of pictures.
1. Understand Vue and Canvas
Briefly introduce Vue and Canvas. Vue is a progressive framework for building user interfaces that renders data into DOM views and updates the view in real time when the data changes. Canvas is a newly added drawing tag in HTML5, which can be used to draw graphics, animations, etc.
2. Preparation work
Before starting the implementation, we need to prepare some basic work.
- Create a Vue project and introduce the Canvas component:
Enter the following command on the command line to create a Vue project:
vue create beautify-app
Then, in src/components Create a component named Canvas.vue in the directory, which means that we will implement the functions of Canvas in this component.
- Add image upload function:
Add an input tag in Canvas.vue to receive image files uploaded by users.
<template> <div> <input type="file" @change="handleImageUpload" /> </div> </template>
3. Implement the beautification function
Next, we will implement the beautification function of the picture. First, we need to introduce a filter image for beauty in Canvas.vue.
- Add filter picture:
Add a picture named beauty.png in the assets directory, which is used as a beauty filter.
- Draw pictures:
In the mounted hook function, we draw the uploaded picture on the canvas.
mounted() { this.canvas = this.$refs.canvas; this.context = this.canvas.getContext('2d'); const image = new Image(); image.src = this.imageUrl; image.onload = () => { this.context.drawImage(image, 0, 0, this.canvas.width, this.canvas.height); }; },
- Apply filters:
After drawing the picture, we use the getImageData
method and putImageData
method of Canvas to Image filters are applied to uploaded images.
applyFilter() { const filterImage = new Image(); filterImage.src = '@/assets/beautify.png'; filterImage.onload = () => { const imageData = this.context.getImageData(0, 0, this.canvas.width, this.canvas.height); const filterContext = this.createFilterContext(filterImage, imageData); this.context.putImageData(filterContext, 0, 0); }; },
Among them, the createFilterContext
method is used to create the context of the filter effect and return it.
4. Realize the dermabrasion function
Next, we will realize the dermabrasion function. The realization of the skin resurfacing function mainly relies on the pixel processing method of Canvas.
- Get pixel data:
We can use the getImageData
method of Canvas to get the pixel data of the image.
const imageData = this.context.getImageData(0, 0, this.canvas.width, this.canvas.height); const data = imageData.data;
- Processing pixel data:
We can process each pixel by traversing the pixel data. Here we can blur the pixels using the Gaussian blur algorithm.
for (let i = 0, len = data.length; i < len; i += 4) { const red = data[i]; const green = data[i + 1]; const blue = data[i + 2]; const alpha = data[i + 3]; // 磨皮处理 // ... }
- Drawing pixel data:
Finally, we use the putImageData
method to draw the processed pixel data onto the Canvas.
const resultImageData = new ImageData(data, imageData.width, imageData.height); this.context.putImageData(resultImageData, 0, 0);
5. Improve the function
After completing the above steps, we can realize the beautification and skin resurfacing functions of the picture by calling methods.
- Upload pictures:
In the handleImageUpload
method, we use the URL.createObjectURL
method to generate a pointer to the user to upload the picture URL.
handleImageUpload(event) { const file = event.target.files[0]; this.imageUrl = URL.createObjectURL(file); },
- Apply filters and smoothing:
In the click event of the button, we call the applyFilter
method and applySmoothing# respectively. ##Methods to apply filters and dermabrasion.
<button @click="applyFilter">应用滤镜</button> <button @click="applySmoothing">应用磨皮</button>6. SummaryThrough the combination of Vue and Canvas, we can easily realize the beautification and skin resurfacing functions of pictures. By processing Canvas pixels, we can develop different beautification algorithms according to needs to provide a better user experience. The following is a complete Canvas.vue code example:
<template> <div> <input type="file" @change="handleImageUpload" /> <button @click="applyFilter">应用滤镜</button> <button @click="applySmoothing">应用磨皮</button> <canvas ref="canvas" :width="canvasWidth" :height="canvasHeight"></canvas> </div> </template> <script> export default { data() { return { imageUrl: '', canvas: null, context: null, canvasWidth: 400, canvasHeight: 300, }; }, mounted() { this.canvas = this.$refs.canvas; this.context = this.canvas.getContext('2d'); }, methods: { handleImageUpload(event) { const file = event.target.files[0]; this.imageUrl = URL.createObjectURL(file); const image = new Image(); image.src = this.imageUrl; image.onload = () => { this.context.drawImage(image, 0, 0, this.canvas.width, this.canvas.height); }; }, applyFilter() { const filterImage = new Image(); filterImage.src = '@/assets/beautify.png'; filterImage.onload = () => { const imageData = this.context.getImageData(0, 0, this.canvas.width, this.canvas.height); const filterContext = this.createFilterContext(filterImage, imageData); this.context.putImageData(filterContext, 0, 0); }; }, applySmoothing() { const imageData = this.context.getImageData(0, 0, this.canvas.width, this.canvas.height); const data = imageData.data; for (let i = 0, len = data.length; i < len; i += 4) { const red = data[i]; const green = data[i + 1]; const blue = data[i + 2]; const alpha = data[i + 3]; // 磨皮处理 // ... } const resultImageData = new ImageData(data, imageData.width, imageData.height); this.context.putImageData(resultImageData, 0, 0); }, createFilterContext(filterImage, imageData) { const filterCanvas = document.createElement('canvas'); filterCanvas.width = this.canvas.width; filterCanvas.height = this.canvas.height; const filterContext = filterCanvas.getContext('2d'); const pattern = filterContext.createPattern(filterImage, 'repeat'); filterContext.fillStyle = pattern; filterContext.fillRect(0, 0, filterCanvas.width, filterCanvas.height); const filterImageData = filterContext.getImageData(0, 0, filterCanvas.width, filterCanvas.height); const filterData = filterImageData.data; const data = imageData.data; for (let i = 0, len = data.length; i < len; i += 4) { data[i] = data[i] * filterData[i] / 255; data[i + 1] = data[i + 1] * filterData[i + 1] / 255; data[i + 2] = data[i + 2] * filterData[i + 2] / 255; } return imageData; }, }, }; </script>In this example, we achieve the beautification and beauty of the image by drawing the uploaded image, and applying filters and skinning. Microdermabrasion function. By flexibly using the APIs of Vue and Canvas, we can customize different beauty algorithms according to needs and provide a better user experience. I hope this article can help you understand how to use Vue and Canvas to implement the beautification and skin-smoothing functions of pictures. Happy programming!
The above is the detailed content of Vue and Canvas: How to implement image beautification and skin resurfacing functions. For more information, please follow other related articles on the PHP Chinese website!

Vue.js' VirtualDOM is both a mirror of the real DOM, and not exactly. 1. Create and update: Vue.js creates a VirtualDOM tree based on component definitions, and updates VirtualDOM first when the state changes. 2. Differences and patching: Comparison of old and new VirtualDOMs through diff operations, and apply only the minimum changes to the real DOM. 3. Efficiency: VirtualDOM allows batch updates, reduces direct DOM operations, and optimizes the rendering process. VirtualDOM is a strategic tool for Vue.js to optimize UI updates.

Vue.js and React each have their own advantages in scalability and maintainability. 1) Vue.js is easy to use and is suitable for small projects. The Composition API improves the maintainability of large projects. 2) React is suitable for large and complex projects, with Hooks and virtual DOM improving performance and maintainability, but the learning curve is steeper.

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
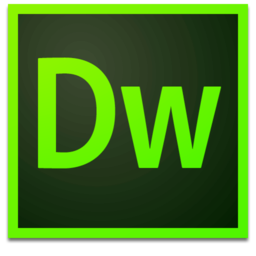
Dreamweaver Mac version
Visual web development tools
