PHP and GD Library Tutorial: How to Convert Picture to Grayscale
PHP and GD library tutorial: How to convert images to grayscale
Overview:
In web development, sometimes you need to perform some processing on images, such as converting color images to grayscale images . In PHP, we can use the GD library to implement this function. The GD library is a graphics processing library for PHP that provides some commonly used image processing functions to easily manipulate images.
Steps:
The following are the steps to convert the image to grayscale:
- Install the GD library:
First, make sure your PHP environment has the GD library installed . If the GD library is not installed, you can enable the GD library by modifying the php.ini file or installing an extension. - Create an image resource:
Use the function provided by the GD library to create an image resource. We will use the imagecreatefromjpeg() function to create image resources from JPEG format image files.
$originalImage = imagecreatefromjpeg('path/to/original_image.jpg');
- Get the image size:
Use the imagesx() and imagesy() functions to get the width and height of the image.
$width = imagesx($originalImage); $height = imagesy($originalImage);
- Create a grayscale image resource:
Use the imagecreatetruecolor() function to create a new grayscale image resource.
$grayImage = imagecreatetruecolor($width, $height);
- Convert to grayscale:
Use the imagecopy() function to convert the RGB value of each pixel in the original image resource into a grayscale value, and convert the converted Pixels are copied to grayscale image resources.
for ($x = 0; $x < $width; $x++) { for ($y = 0; $y < $height; $y++) { $rgb = imagecolorat($originalImage, $x, $y); $r = ($rgb >> 16) & 0xFF; $g = ($rgb >> 8) & 0xFF; $b = $rgb & 0xFF; $gray = round(($r + $g + $b) / 3); $grayColor = imagecolorallocate($grayImage, $gray, $gray, $gray); imagesetpixel($grayImage, $x, $y, $grayColor); } }
- Save grayscale images:
Use the imagejpeg() function to save grayscale image resources as image files in JPEG format.
imagejpeg($grayImage, 'path/to/gray_image.jpg');
Complete code example:
$originalImage = imagecreatefromjpeg('path/to/original_image.jpg'); $width = imagesx($originalImage); $height = imagesy($originalImage); $grayImage = imagecreatetruecolor($width, $height); for ($x = 0; $x < $width; $x++) { for ($y = 0; $y < $height; $y++) { $rgb = imagecolorat($originalImage, $x, $y); $r = ($rgb >> 16) & 0xFF; $g = ($rgb >> 8) & 0xFF; $b = $rgb & 0xFF; $gray = round(($r + $g + $b) / 3); $grayColor = imagecolorallocate($grayImage, $gray, $gray, $gray); imagesetpixel($grayImage, $x, $y, $grayColor); } } imagejpeg($grayImage, 'path/to/gray_image.jpg'); imagedestroy($originalImage); imagedestroy($grayImage);
Summary:
Through the above steps, we can easily convert a color image into a grayscale image. Using the functions of the GD library, you can easily create image resources, obtain image dimensions, convert to grayscale, and save images. In actual development, we can perform more processing and operations on images according to specific needs. Hope this tutorial helps you!
The above is the detailed content of PHP and GD Library Tutorial: How to Convert Picture to Grayscale. For more information, please follow other related articles on the PHP Chinese website!
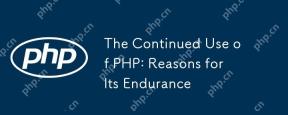
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
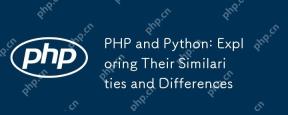
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
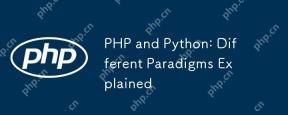
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
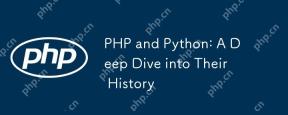
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
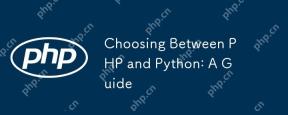
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
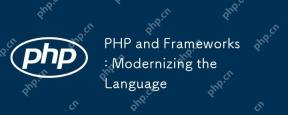
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
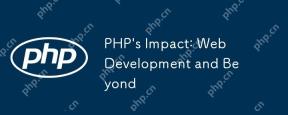
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
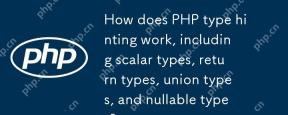
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
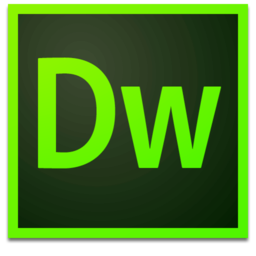
Dreamweaver Mac version
Visual web development tools
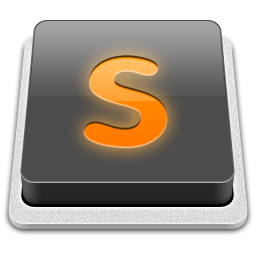
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
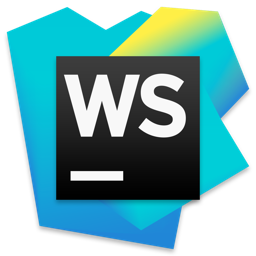
WebStorm Mac version
Useful JavaScript development tools