How to deal with PHP error: Call to undefined function?
How to deal with PHP error: Call to undefined function?
In the process of using PHP development, we often encounter various error reports. One of the common errors is "Call to undefined function", which means that an undefined function is called. This kind of error may cause the code to fail and cause trouble to developers. This article explains how to handle this error and provides some code examples.
-
Check whether the function is correctly defined:
When a "Call to undefined function" error occurs, first check whether the definition of the function actually exists. This may be caused by a misspelling of the function name or an incorrect definition of the function. Make sure the function name is in the same case as when it was defined, and that the function is defined before it is called.// 函数定义 function myFunction() { // 函数体 } // 函数调用 myFunction();
-
Check whether the file where the function is located is correctly imported:
If the function is defined in another file, then you need to confirm whether the file is correctly imported. Use PHP's include or require statement to introduce the file into the current file, making sure the file path is correct.// 引入文件 require_once('functions.php'); // 函数调用 myFunction();
-
Check whether the PHP version supports this function:
Some functions are introduced in a specific PHP version, so if the PHP version used is lower, an error "Call" will be reported to undefined function". You can use thefunction_exists
function to check to make sure the function exists in the current PHP version.// 检查函数是否存在 if (function_exists('myFunction')) { // 函数调用 myFunction(); } else { echo "Function does not exist"; }
-
Check whether the extension where the function is located has been loaded:
Some functions are provided through the PHP extension library. If the corresponding extension is not loaded, an error "Call to undefined function" will be reported ". You can use theextension_loaded
function to check to make sure the extension is loaded.// 检查扩展是否加载 if (extension_loaded('my_extension')) { // 函数调用 myFunction(); } else { echo "Extension not loaded"; }
-
Check whether the function is in the namespace:
If the function is defined in the namespace, you need to specify the complete namespace path when calling the function.// 函数定义在命名空间 MyNamespace 中 namespace MyNamespace; function myFunction() { // 函数体 } // 函数调用 MyNamespacemyFunction();
-
Use try-catch block to handle exceptions:
If you cannot determine whether the function exists, you can use try-catch block to catch the exception and handle it accordingly.try { myFunction(); } catch (Error $e) { echo "Function call failed: " . $e->getMessage(); }
The above are several common methods and code examples for handling "Call to undefined function" errors. Depending on the situation, choose the appropriate method to solve the problem. Remember, carefully checking the details of function definitions, file imports, PHP versions, and extension loading when writing and debugging code can effectively reduce the occurrence of such errors.
The above is the detailed content of How to deal with PHP error: Call to undefined function?. For more information, please follow other related articles on the PHP Chinese website!
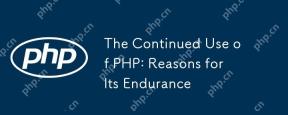
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
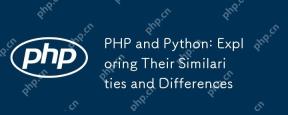
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
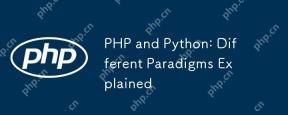
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
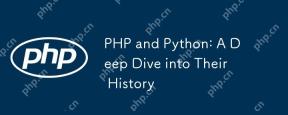
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
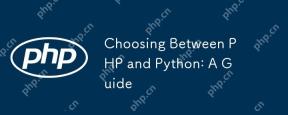
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
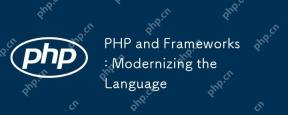
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
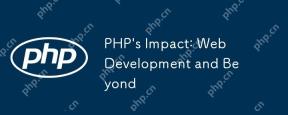
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
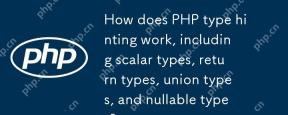
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
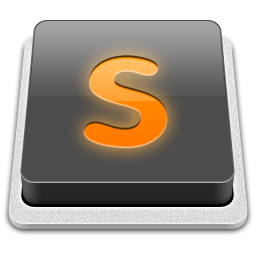
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor