


How to find multiple values in an array in PHP and return the corresponding key names
How to find multiple values in an array and return the corresponding key name in PHP
In PHP development, we often encounter the need to find multiple values in an array and find the key of the corresponding value name situation. This article will introduce a method to achieve this function and provide corresponding code examples.
First, let's look at the structure of the array we are looking for. Suppose we have an associative array that stores some user information, such as username, age, and gender. An example is as follows:
$user_info = array( 'john' => array( 'age' => 25, 'gender' => 'male' ), 'amy' => array( 'age' => 32, 'gender' => 'female' ), 'peter' => array( 'age' => 28, 'gender' => 'male' ), 'susan' => array( 'age' => 30, 'gender' => 'female' ) );
Now, suppose we need to find a user whose age is 32 years old and whose gender is female. We hope to get the key name of the user, which is 'amy'. The following is a code example to implement this function:
function searchUser($array, $conditions) { foreach ($array as $key => $value) { $match = 0; foreach ($conditions as $condition_key => $condition_value) { if ($value[$condition_key] == $condition_value) { $match++; } } if ($match == count($conditions)) { return $key; } } return null; } $conditions = array( 'age' => 32, 'gender' => 'female' ); $result = searchUser($user_info, $conditions); if ($result) { echo "找到匹配的用户,键名为:" . $result; } else { echo "未找到匹配的用户"; }
In the above code, we define a function named searchUser
, which accepts two parameters: the array to be searched and the search conditions .
Inside the function, we use two levels of nested loops. The outer loop traverses each element of the array, and the inner loop traverses each condition in the search condition array.
In the inner loop, we compare whether the value of the corresponding key in the array is equal to the current search condition. If they are equal, we increment the value of a counter $match
. If the value of $match
is equal to the length of the search condition array, it means that all conditions are matched, and we return the key name of the current loop.
If no matching user is found, we end up returning null
.
In the main program, we define an array of search conditions $conditions
, and then call the searchUser
function to find matching users. Finally, based on the returned results, we output the corresponding prompt information.
Through the above code example, we can realize the function of finding multiple values in an array and returning the corresponding key name.
Summary:
In PHP development, sometimes we need to find multiple values in an array and return the corresponding key names. By using a double loop and appropriate judgment conditions, we can achieve this functionality. The above code example provides an implementation method for your reference and use. I hope this article will be helpful to everyone in PHP array search.
The above is the detailed content of How to find multiple values in an array in PHP and return the corresponding key names. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
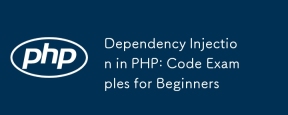
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
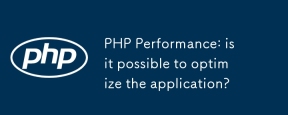
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
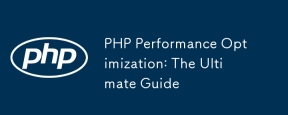
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
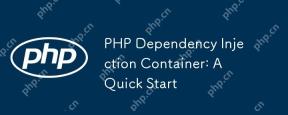
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
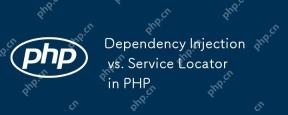
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
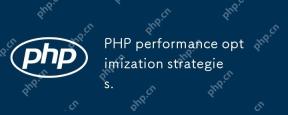
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
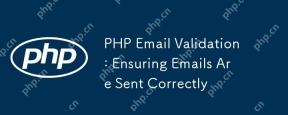
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
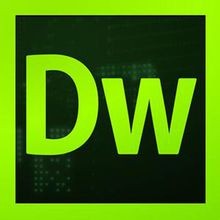
Dreamweaver CS6
Visual web development tools
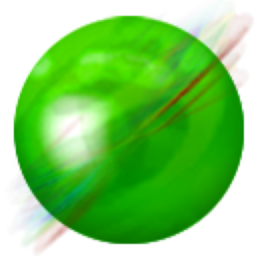
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
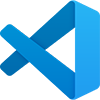
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
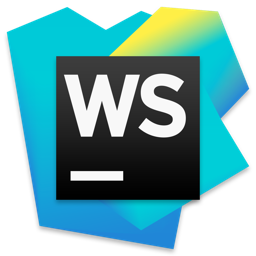
WebStorm Mac version
Useful JavaScript development tools
