Use Python and WebDriver to automatically handle web pop-ups
Use Python and WebDriver to automatically process web page pop-up windows
Introduction:
When conducting web automation testing, you often encounter pop-up windows on the web page. These pop-up windows may be prompt boxes and confirmation boxes. , input box, etc. For the processing of these pop-up windows, we can use Python and WebDriver to automate operations to improve testing efficiency. This article will introduce how to use Python and WebDriver to automatically handle web page pop-ups, and attach code examples.
1. Install Python and WebDriver
- Install Python
First, we need to install the Python interpreter. We can download the latest version of Python from the official website (https://www.python.org/). During the installation process, be sure to check the "Add Python to PATH" option. - Install WebDriver
WebDriver is a browser automation tool that can simulate user operations in the browser. Common WebDrivers include Chrome Driver, Firefox Driver, etc. We can choose the appropriate WebDriver to install according to actual needs. Taking the Chrome Driver as an example, we can download the corresponding version of the Chrome Driver from the Chrome official website (https://sites.google.com/a/chromium.org/chromedriver/).
2. Use WebDriver to automatically process web page pop-ups
The following is a sample code that demonstrates how to use Python and WebDriver to automatically process web page pop-ups.
from selenium import webdriver from selenium.webdriver.common.alert import Alert # 创建WebDriver对象 driver = webdriver.Chrome("path_to_chromedriver") # 打开网页 driver.get("https://www.example.com") # 处理提示框 alert = Alert(driver) alert.accept() # 处理确认框 confirm = Alert(driver) confirm.dismiss() # 处理输入框 prompt = Alert(driver) prompt.send_keys("Hello, World!") prompt.accept() # 关闭WebDriver对象 driver.quit()
Code explanation:
- First, we need to import the
webdriver
module and theAlert
class. Thewebdriver
module provides related methods for operating the browser, and theAlert
class is used to handle pop-up windows. - Create
WebDriver
object, here we use Chrome Driver as an example.path_to_chromedriver
needs to be replaced with the actual Chrome Driver path. - Use the
get
method to open the web page that needs to be tested. - Use the
accept
method of theAlert
class to accept/confirm the prompt box. - Use the
dismiss
method of theAlert
class to cancel the confirmation box. - Use the
send_keys
method of theAlert
class to enter text in the input box. - Use the
accept
method of theAlert
class to accept/confirm the input box. - Finally, use the
quit
method to close the WebDriver object.
Summary:
This article introduces how to use Python and WebDriver to automatically handle web page pop-ups, and demonstrates specific operations through code examples. In this way, we can improve the efficiency of automated testing and reduce the time and workload of manual operations. When you need to deal with web page pop-ups, you can refer to the method in this article to implement it. I hope this article is helpful to your work in automated testing.
The above is the detailed content of Use Python and WebDriver to automatically handle web pop-ups. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
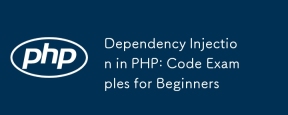
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
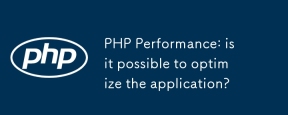
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
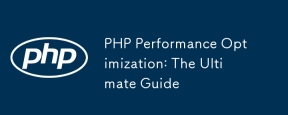
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
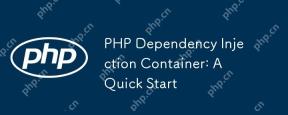
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
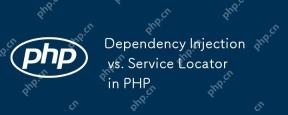
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
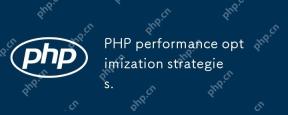
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
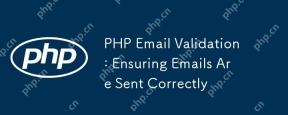
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
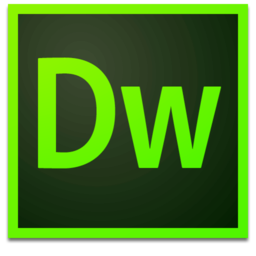
Dreamweaver Mac version
Visual web development tools
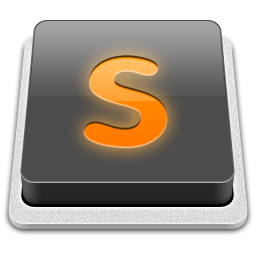
SublimeText3 Mac version
God-level code editing software (SublimeText3)
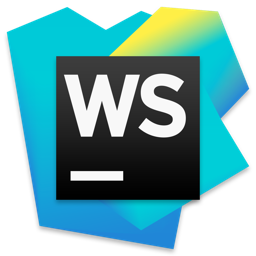
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
