


Python calls the Alibaba Cloud interface to implement data backup and recovery functions
Python calls the Alibaba Cloud interface to implement data backup and recovery functions
In recent years, data backup and recovery have become an indispensable and important link in the construction of enterprise informatization. With the popularity of cloud computing and the rise of cloud service providers such as Alibaba Cloud, cloud data backup and recovery has become a more efficient and reliable choice. This article will introduce how to use Python to call Alibaba Cloud's API interface to implement data backup and recovery functions.
Alibaba Cloud provides a wealth of API interfaces, including the interface of Alibaba Cloud OSS (Object Storage Service). OSS is a distributed object storage service provided by Alibaba Cloud. It is suitable for scenarios where large amounts of unstructured data are stored and accessed.
First, we need to create an OSS Bucket in Alibaba Cloud to store backup data. In the Alibaba Cloud console, select the OSS service, then click "Create Bucket" and follow the prompts to complete the creation.
Next, we need to install the aliyun-python-sdk-oss package. Execute the following command in the command line:
pip install aliyun-python-sdk-oss
After the installation is complete, we can start writing Python code for data backup. First, import the required libraries:
import os from aliyunsdkcore import client from aliyunsdkcore.profile import region_provider from aliyunsdkcore.auth.credentials import StsTokenCredential from aliyunsdkoss.request import PutObjectRequest
Then, set the access key and secret key of Alibaba Cloud:
access_key_id = '<Your Access Key ID>' access_key_secret = '<Your Access Key Secret>'
Next, set the OSS area and Endpoint:
region_provider.add_endpoint('oss', '<Your OSS Region>', '<Your OSS Endpoint>')
Then , create an OSS client:
credential = StsTokenCredential(access_key_id, access_key_secret, '') clt = client.AcsClient(region_id="<Your OSS Region>", credential=credential)
Next, define a function for backing up data:
def backup_data(bucket_name, file_path): request = PutObjectRequest.PutObjectRequest() request.set_BucketName(bucket_name) request.set_Key(os.path.basename(file_path)) request.set_FilePath(file_path) response = clt.do_action_with_exception(request) print(response)
The above function accepts two parameters, bucket_name
means to back up to Bucket name, file_path
indicates the file path to be backed up. The function will upload the file to the specified Bucket.
To perform data backup, just call the backup_data
function and pass in the corresponding parameters:
backup_data('my-bucket', '/path/to/backup/file.txt')
Next, let’s implement the data recovery function. Select the corresponding Bucket in the Alibaba Cloud console and find the files that need to be restored. Click "Download" to get the download link for the file.
Next, we write Python code to perform data recovery. First, import the required libraries:
from aliyunsdkcore.request import GetObjectRequest
Then, define a function to restore data:
def restore_data(bucket_name, file_name, save_path): request = GetObjectRequest.GetObjectRequest() request.set_BucketName(bucket_name) request.set_Key(file_name) response = clt.do_action_with_exception(request) with open(save_path, 'wb') as f: f.write(response)
The above function accepts three parameters, bucket_name
represents the Bucket to be restored Name, file_name
indicates the name of the file to be restored, save_path
indicates the path to save the recovered file. The function will download the specified file from the Bucket and save it locally.
To perform data recovery, just call the restore_data
function and pass in the corresponding parameters:
restore_data('my-bucket', 'file.txt', '/path/to/save/file.txt')
At this point, we have implemented using Python to call Ali Cloud interface for data backup and recovery functions. By calling Alibaba Cloud's API interface, we can easily complete data backup and recovery and improve data security and reliability. Hope this article will be helpful to you.
The above is the detailed content of Python calls the Alibaba Cloud interface to implement data backup and recovery functions. For more information, please follow other related articles on the PHP Chinese website!
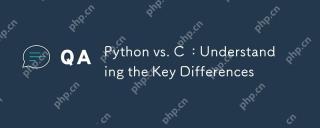
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
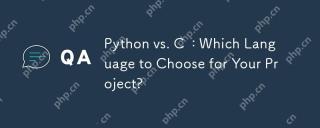
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
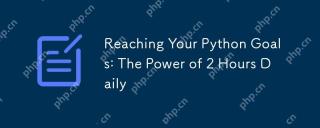
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
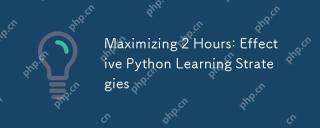
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
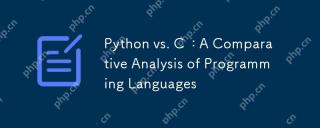
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
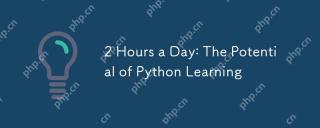
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
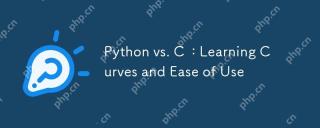
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
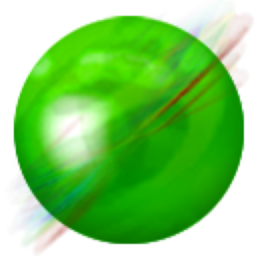
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
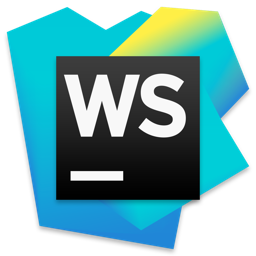
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor