


Teach you step by step how to use Python to connect to Qiniu Cloud interface to achieve audio conversion and editing
Teach you step by step how to use Python to connect to the Qiniu Cloud interface to achieve audio conversion and editing
In the field of audio processing and conversion, Qiniu Cloud is a very powerful and convenient cloud service provider. By connecting to Qiniu Cloud's interface, we can easily implement audio format conversion, editing, compression and other operations. This article will teach you step by step how to use Python to write code, connect to Qiniu Cloud interface, and realize audio conversion and editing functions.
First, we need to create a storage space on Qiniu Cloud and upload the audio files to be processed in the storage space. Then, we need to install the corresponding Python library. Execute the following command in the terminal:
pip install qiniu pip install requests
After the installation is complete, we can start writing code. First, we need to import the relevant libraries and configure the Access Key and Secret Key of Qiniu Cloud.
import qiniu import requests access_key = 'YOUR_ACCESS_KEY' secret_key = 'YOUR_SECRET_KEY'
Next, we need to get the URL of the audio file on Qiniu Cloud. Assume that our storage space on Qiniu Cloud is named bucket_name
and the file name is file_name
.
bucket_name = 'YOUR_BUCKET_NAME' file_name = 'YOUR_FILE_NAME' url = 'http://{}.qiniudn.com/{}'.format(bucket_name, file_name)
Next, we can use Qiniu Cloud’s audio processing interface to implement audio conversion and editing functions. Specific interface documents can be found in the Qiniu Cloud official documentation. Here, we take converting audio as an example.
def convert_audio(url, format): pipeline = 'your_pipeline' fops = 'avthumb/{}'.format(format) save_as = qiniu.urlsafe_base64_encode('{}.{}'.format(file_name, format)) persistent_ops = '{}|saveas/{}'.format(fops, save_as) notify_url = '' pfop = qiniu.PersistentFop(access_key, secret_key) ret, info = pfop.execute(bucket_name, file_name, persistent_ops, pipeline, notify_url) if ret['persistentId']: print('转换任务已提交,任务ID:{}'.format(ret['persistentId'])) else: print('转换失败:{}'.format(info))
In the convert_audio
method, we need to pass in the URL of the audio file on Qiniu Cloud and the format to be converted. pipeline
is the name of the pipeline for Qiniu Cloud audio processing, which can be created in the Qiniu Cloud console. fops
is the specific conversion operation. Here we use the avthumb
command to convert the audio format. save_as
is the saving path of the converted audio, using the saveas
command of Qiniu Cloud. persistent_ops
is the final audio processing instruction. The pfop.execute
method is used to submit the conversion task, and the returned persistentId
can be used to query the status of the conversion task.
Finally, we can call the convert_audio
method in the main function and pass in the corresponding parameters.
if __name__ == '__main__': convert_audio(url, 'mp3')
In this article, we teach you step by step how to use Python to connect to the Qiniu Cloud interface to implement audio conversion and editing functions. Through Qiniu Cloud's powerful audio processing interface, we can easily implement audio format conversion, as well as more complex editing, compression and other operations. I hope this article can be helpful to your learning and application in audio processing.
import qiniu import requests access_key = 'YOUR_ACCESS_KEY' secret_key = 'YOUR_SECRET_KEY' bucket_name = 'YOUR_BUCKET_NAME' file_name = 'YOUR_FILE_NAME' url = 'http://{}.qiniudn.com/{}'.format(bucket_name, file_name) def convert_audio(url, format): pipeline = 'your_pipeline' fops = 'avthumb/{}'.format(format) save_as = qiniu.urlsafe_base64_encode('{}.{}'.format(file_name, format)) persistent_ops = '{}|saveas/{}'.format(fops, save_as) notify_url = '' pfop = qiniu.PersistentFop(access_key, secret_key) ret, info = pfop.execute(bucket_name, file_name, persistent_ops, pipeline, notify_url) if ret['persistentId']: print('转换任务已提交,任务ID:{}'.format(ret['persistentId'])) else: print('转换失败:{}'.format(info)) if __name__ == '__main__': convert_audio(url, 'mp3')
The above is the detailed content of Teach you step by step how to use Python to connect to Qiniu Cloud interface to achieve audio conversion and editing. For more information, please follow other related articles on the PHP Chinese website!
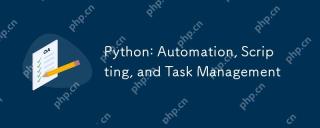
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
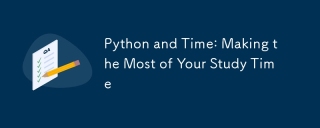
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
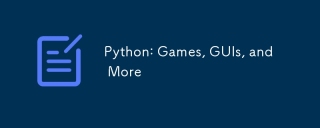
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
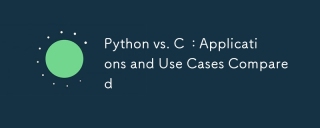
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
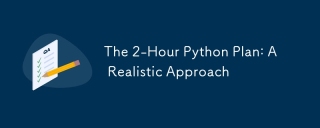
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
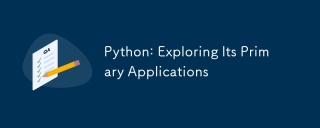
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
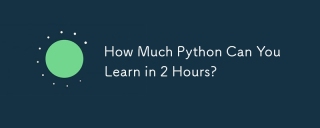
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
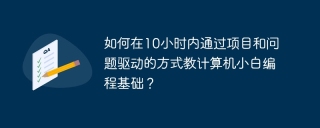
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!