


How to connect to Alibaba Cloud SMS service through PHP to implement SMS notification function
How to connect to Alibaba Cloud SMS Service through PHP to implement SMS notification function
Alibaba Cloud SMS Service is a reliable and efficient SMS sending platform that can help developers implement SMS notification functions in applications. This article will introduce how to use PHP code to connect to Alibaba Cloud SMS service to implement SMS notification function.
First, we need to create an Access Key and Access Secret for the SMS service on the Alibaba Cloud platform. Then, we can use the SDK library of Alibaba Cloud SMS Service to make interface calls through PHP code.
Step 1: Install the SDK library
Alibaba Cloud officially provides an SDK library for PHP, which we can install through Composer. Create a composer.json file in the project root directory and add the following content:
{ "require": { "aliyuncs/aliyun-sdk": "dev-master" } }
Then, execute the following command in the command line terminal to install the SDK library:
composer install
Step 2: Write PHP code
Create a PHP file, such as send_sms.php
, and then introduce the auto-loading file and namespace of the SDK library.
<?php require_once 'vendor/autoload.php'; use AliyunCoreConfig; use AliyunCoreDefaultAcsClient; use AliyunCoreProfileDefaultProfile; use AliyunApiSmsRequestV20170525SendSmsRequest;
Next, configure the Access Key, Access Secret, and other necessary parameters.
Config::load(); $accessKeyId = 'your_access_key_id'; $accessKeySecret = 'your_access_key_secret'; $profile = DefaultProfile::getProfile('cn-hangzhou', $accessKeyId, $accessKeySecret); DefaultProfile::addEndpoint('cn-hangzhou', 'cn-hangzhou', 'Dysmsapi', 'dysmsapi.aliyuncs.com'); $client = new DefaultAcsClient($profile); $request = new SendSmsRequest(); $request->setPhoneNumbers('手机号码'); $request->setSignName('短信签名'); $request->setTemplateCode('短信模板代码'); $request->setTemplateParam('{"code":"123456"}');
In the above code, replace your_access_key_id
and your_access_key_secret
with the Access Key and Access Secret you obtained on the Alibaba Cloud platform. In addition, set Mobile phone number
, SMS signature
and SMS template code
to your own actual values. If necessary, you can also set other template parameters.
Finally, call the interface of Alibaba Cloud SMS Service to send the text message.
try { $response = $client->getAcsResponse($request); // 处理响应结果 if ($response->Code == 'OK') { echo '短信发送成功!'; } else { echo '短信发送失败:' . $response->Code; } } catch (Exception $e) { echo '短信发送失败:' . $e->getMessage(); }
The above code sends a text message by calling the $client->getAcsResponse($request)
method and processes the response result. If the SMS is sent successfully, SMS sent successfully will be output!
, otherwise an error message will be output.
Step 3: Run the code
Before running the PHP code, you need to ensure that PHP and Composer have been installed in your development environment. Execute the following command in the command line terminal to run the code:
php send_sms.php
If everything goes well, you will see the message that the SMS was sent successfully.
Through the above steps, we successfully used PHP code to connect to Alibaba Cloud SMS service and implemented the SMS notification function. You can make more customizations and extensions based on actual needs, such as sending verification codes, sending marketing campaign text messages, etc.
I hope this article can provide some help for you to understand and use Alibaba Cloud SMS Service.
The above is the detailed content of How to connect to Alibaba Cloud SMS service through PHP to implement SMS notification function. For more information, please follow other related articles on the PHP Chinese website!
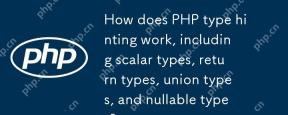
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
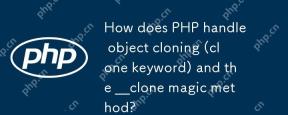
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
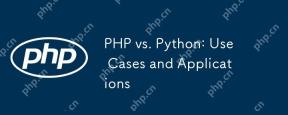
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
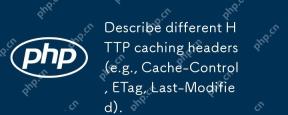
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
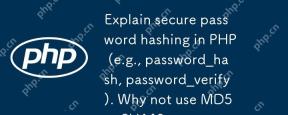
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
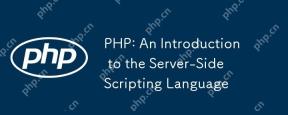
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
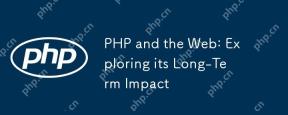
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
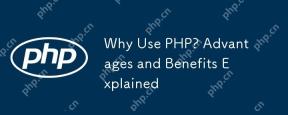
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
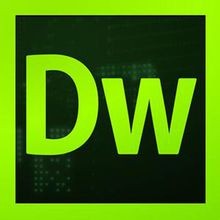
Dreamweaver CS6
Visual web development tools
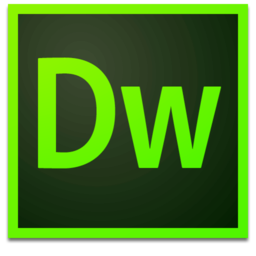
Dreamweaver Mac version
Visual web development tools