


Qiniu Cloud Data Processing and Management Guide: How does the Java SDK implement data operations and analysis?
Introduction:
With the advent of the big data era, data processing and analysis are becoming more and more important. As an enterprise focusing on cloud storage and data services, Qiniu Cloud provides a wealth of data processing and analysis functions to facilitate users to process and analyze massive data. This article will introduce how to use Qiniu Cloud's Java SDK to implement data operations and analysis.
1. Preparation
Before we start, we need to prepare some necessary tools and environment:
- Apply for Qiniu Cloud account and create storage space.
-
Install Java SDK. Dependencies can be managed through Maven. Add the following dependencies to the pom.xml file:
<dependency> <groupId>com.qiniu</groupId> <artifactId>qiniu-java-sdk</artifactId> <version>[7.2.0,)</version> </dependency>
- Configure Access Key and Secret Key. In the Qiniu Cloud console, click the avatar in the upper right corner, select Key Management, and you can find the Access Key and Secret Key.
2. Data upload
Using Qiniu Cloud’s Java SDK, you can easily upload data to the storage space. The following is a simple sample code:
import com.qiniu.common.QiniuException; import com.qiniu.http.Response; import com.qiniu.storage.Configuration; import com.qiniu.storage.UploadManager; import com.qiniu.util.Auth; public class UploadExample { public static void main(String[] args) { // Access Key和Secret Key String accessKey = "your-access-key"; String secretKey = "your-secret-key"; // 创建Auth对象 Auth auth = Auth.create(accessKey, secretKey); // 存储空间名称 String bucketName = "your-bucket-name"; // 上传文件路径 String filePath = "/path/to/file"; // 生成上传凭证 String uploadToken = auth.uploadToken(bucketName); // 上传文件 Configuration config = new Configuration(); UploadManager uploadManager = new UploadManager(config); try { Response response = uploadManager.put(filePath, null, uploadToken); // 处理上传成功的逻辑 System.out.println("上传成功"); } catch (QiniuException e) { // 处理上传失败的逻辑 System.out.println("上传失败,错误信息:" + e.error()); } } }
In the sample code, you need to replace the Access Key, Secret Key and storage space name with your own information. Then generate the upload credentials through the Auth object, and then upload the file through UploadManager.
3. Data processing
Qiniu Cloud provides a wealth of data processing functions, including image processing, audio and video processing, document conversion, etc. The following is a sample code for image processing:
import com.qiniu.common.QiniuException; import com.qiniu.http.Response; import com.qiniu.processing.OperationManager; import com.qiniu.util.Auth; public class ImageProcessExample { public static void main(String[] args) { // Access Key和Secret Key String accessKey = "your-access-key"; String secretKey = "your-secret-key"; // 创建Auth对象 Auth auth = Auth.create(accessKey, secretKey); // 存储空间名称 String bucketName = "your-bucket-name"; // 待处理的图片URL String imageUrl = "http://your-domain.com/path/to/image.jpg"; // 图片处理参数 String imageParams = "imageView2/0/w/500/h/500"; // 创建操作管理器 OperationManager operationManager = new OperationManager(auth); try { // 对图片进行处理 String processedUrl = operationManager.pfop(bucketName, imageUrl, imageParams); // 处理成功后会返回处理后的图片URL System.out.println("处理成功,处理后的图片URL:" + processedUrl); } catch (QiniuException e) { // 处理失败的逻辑 System.out.println("处理失败,错误信息:" + e.response.error); } } }
In the sample code, you need to replace the Access Key, Secret Key and storage space name with your own information. Then the operation manager OperationManager is generated through the Auth object, and then the pfop method is called to process the image.
Conclusion:
This article introduces how to use Qiniu Cloud’s Java SDK to implement data operations and analysis. Through the rich functions of Qiniu Cloud, data can be easily uploaded and processed. Hope this article is helpful to you!
The above is the detailed content of Qiniu Cloud Data Processing and Management Guide: How does the Java SDK implement data operations and analysis?. For more information, please follow other related articles on the PHP Chinese website!
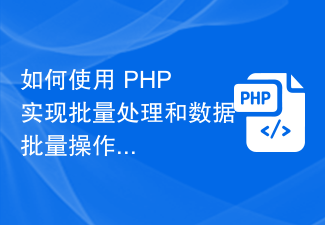
如何使用PHP实现批量处理和数据批量操作在开发Web应用程序过程中,经常会遇到需要同时处理多条数据的情况。为了提高效率和减少数据库请求的次数,我们可以使用PHP来实现批量处理和数据批量操作。本文将介绍如何使用PHP来实现这些功能,并附加代码示例以供参考。批量处理数据当需要对大量数据进行相同的操作时,可以使用PHP的循环结构来进行批量处理。
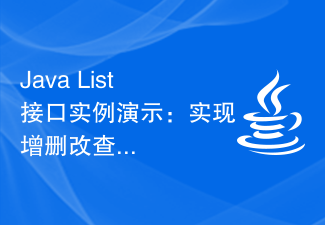
JavaList接口是Java中常用的数据结构之一,可以方便地实现数据的增删改查操作。本文将通过一个示例来演示如何使用JavaList接口来实现数据的增删改查操作。首先,我们需要在代码中引入List接口的实现类,常见的有ArrayList和LinkedList。这两个类都实现了List接口,具有类似的功能但底层实现方式不同。ArrayList是基于数组实
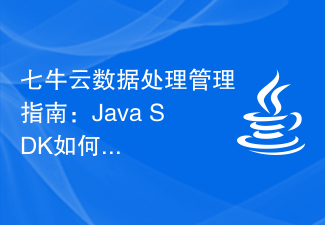
七牛云数据处理管理指南:JavaSDK如何实现数据操作和分析?引言:随着大数据时代的到来,数据处理和分析变得越来越重要。七牛云作为一家专注于云存储和数据服务的企业,提供了丰富的数据处理和分析功能,方便用户处理和分析海量数据。本文将介绍如何使用七牛云的JavaSDK来实现数据操作和分析。一、准备工作在开始之前,我们需要准备一些必要的工具和环境:申请七牛云账
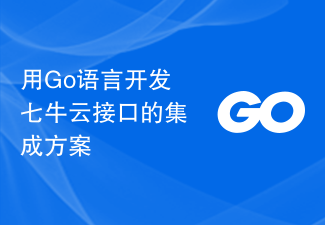
用Go语言开发七牛云接口的集成方案引言:随着云计算的普及,越来越多的企业开始将数据存储在云上。七牛云作为一家主要的云存储服务提供商,为用户提供了稳定高效的对象存储服务。本文将介绍如何使用Go语言来开发七牛云接口的集成方案,并附上代码示例。一、概述七牛云的接口提供了丰富的功能,包括文件上传、下载、删除、查看文件列表等。为了方便开发者使用这些功能,我们可以使用G
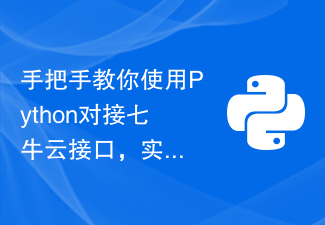
手把手教你使用Python对接七牛云接口,实现音频切割在音频处理领域,七牛云是一个非常优秀的云存储平台,提供了丰富的接口来对音频进行各种处理。本文将以Python为例,手把手教你如何对接七牛云接口,实现音频切割的功能。首先,我们需要安装相应的Python库,用于与七牛云进行交互。在命令行中输入以下命令进行安装:pipinstallqiniu安装完成后,我
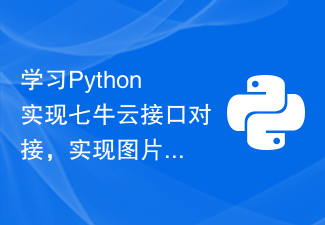
学习Python实现七牛云接口对接,实现图片滤镜合成摘要:随着云计算和大数据技术的快速发展,云存储和云服务成为了现代应用开发中不可或缺的一部分。七牛云作为一家领先的云服务提供商,为开发者提供了丰富的云存储及相关服务。本文将介绍如何使用Python语言对接七牛云接口,并实现图片滤镜合成的功能。同时,将通过代码示例,帮助读者更好地理解实现过程。1.安装依赖库在开
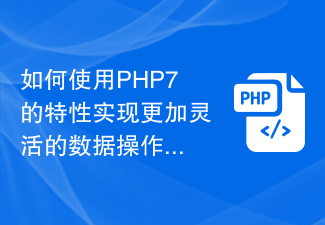
如何使用PHP7的特性实现更加灵活的数据操作和处理?随着PHP7的发布,PHP编程语言又迈入了一个新的阶段。PHP7带来了许多令人兴奋的特性,特别是在数据操作和处理方面,提供了更多的灵活性和效率。本文将介绍如何利用PHP7的特性来实现更加灵活的数据操作和处理,以及一些具体的代码示例。类型声明在PHP7中,我们可以通过使用类型声明,明确函数或方法的参数和返回值
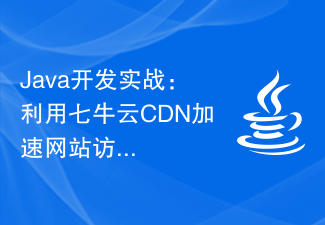
Java开发实战:利用七牛云CDN加速网站访问引言:随着互联网的发展,网站的访问速度成为了用户体验的重要因素之一。为了提高网站的访问速度,许多开发者选择利用内容分发网络(CDN)进行加速。七牛云(QiniuCloud)作为国内领先的云服务提供商,提供了一套完整的云加速解决方案。本文将介绍如何利用七牛云CDN加速网站访问,并附上Java代码示例。一、申请七牛


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
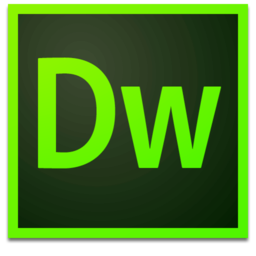
Dreamweaver Mac version
Visual web development tools
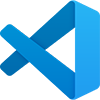
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
