


Configuring Linux systems to support multi-threaded programming
In the current development of computer applications, multi-threaded programming has become very common. Multithreaded programming allows programs to perform multiple tasks simultaneously, thereby improving system performance and responsiveness. This article will introduce how to configure a Linux system to support multi-threaded programming and give some code examples.
- Install necessary software packages
First, we need to install some necessary software packages for multi-threaded programming on Linux systems. These packages can be installed using the following command:
sudo apt-get update sudo apt-get install build-essential sudo apt-get install libpthread-stubs0-dev
The build-essential package provides the tools and libraries required for compilation and linking. The libpthread-stubs0-dev package provides header files and static libraries related to the POSIX thread library.
- Writing multi-threaded programs
Next, we will write a simple multi-threaded program to demonstrate how to perform multi-threaded programming on a Linux system. We will use C language and POSIX thread library to write this program. Please save the following code as main.c file.
#include <stdio.h> #include <pthread.h> #define NUM_THREADS 5 void *threadFunc(void *arg) { int threadNum = *(int*)arg; printf("This is thread %d ", threadNum); pthread_exit(NULL); } int main() { pthread_t tid[NUM_THREADS]; int i; for (i = 0; i < NUM_THREADS; i++) { int *threadNum = malloc(sizeof(int)); *threadNum = i; pthread_create(&tid[i], NULL, threadFunc, threadNum); } for (i = 0; i < NUM_THREADS; i++) { pthread_join(tid[i], NULL); } return 0; }
In this program, we define a threadFunc function, which serves as the entry point for each thread. In this function, we simply print out the thread's number.
In the main function, we use the pthread_create function to create NUM_THREADS threads and pass their numbers to the threadFunc function. Then, we use the pthread_join function to wait for the completion of all threads.
- Compile and run the program
We can use the following command to compile this program:
gcc -o program_name main.c -lpthread
Here, the -lpthread option is used to link the POSIX thread library .
After successful compilation, we can run the program:
./program_name
When running the program, we will see the output showing the number of each thread.
Summary
This article introduces how to configure a Linux system to support multi-threaded programming and gives a simple multi-threaded programming example. By taking full advantage of multi-threaded programming, we can improve the performance and responsiveness of our systems. I hope this article will help you with multi-threaded programming on Linux systems.
The above is the detailed content of Configuring Linux systems to support multi-threaded programming. For more information, please follow other related articles on the PHP Chinese website!
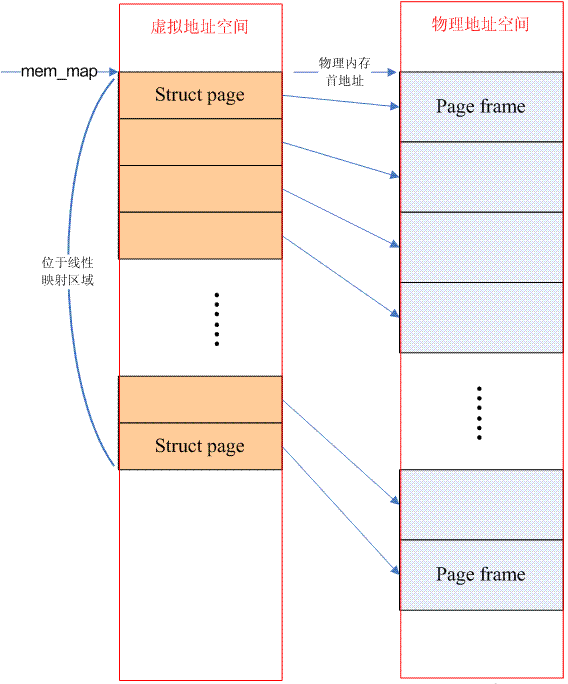
你是否曾经遇到过在Linux系统中出现的各种内存问题?比如内存泄漏、内存碎片等等。这些问题都可以通过深入理解Linux内存模型得到解决。一、前言在linux内核中支持3中内存模型,分别是flatmemorymodel,Discontiguousmemorymodel和sparsememorymodel。所谓memorymodel,其实就是从cpu的角度看,其物理内存的分布情况,在linuxkernel中,使用什么的方式来管理这些物理内存。另外,需要说明的是:本文主要focus在sharememo
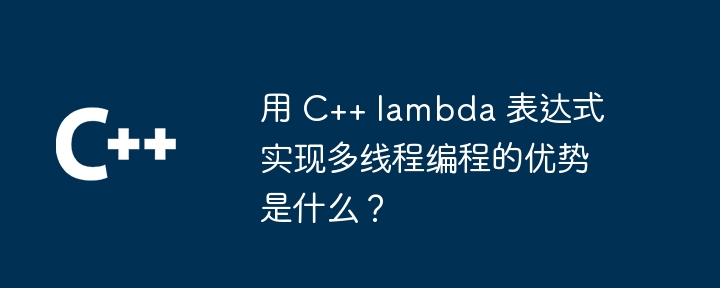
lambda表达式在C++多线程编程中的优势包括:简洁性、灵活性、易于传参和并行性。实战案例:使用lambda表达式创建多线程,在不同线程中打印线程ID,展示了该方法的简洁和易用性。
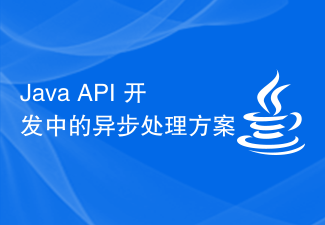
随着Java技术的不断发展,JavaAPI已经成为许多企业开发的主流方案之一。在JavaAPI开发过程中,常常需要对大量的请求和数据进行处理,但是传统的同步处理方式无法满足高并发、高吞吐量的需求。因此,异步处理成为了JavaAPI开发中的重要解决方案之一。本文将介绍JavaAPI开发中常用的异步处理方案及其使用方法。一、Java异
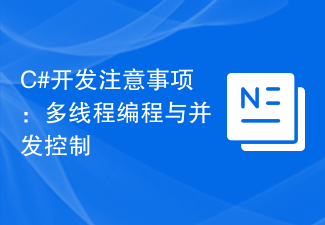
在C#开发中,面对不断增长的数据和任务,多线程编程和并发控制显得尤为重要。本文将从多线程编程和并发控制两个方面,为大家介绍一些在C#开发中需要注意的事项。一、多线程编程多线程编程是一种利用CPU多核心资源提高程序效率的技术。在C#程序中,多线程编程可以使用Thread类、ThreadPool类、Task类以及Async/Await等方式实现。但在进行多线程编
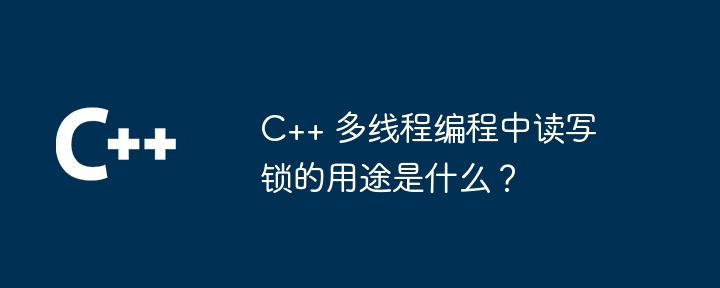
多线程中,读写锁允许多个线程同时读取数据,但只允许一个线程写入数据,以提高并发性和数据一致性。C++中的std::shared_mutex类提供了以下成员函数:lock():获取写入访问权限,当没有其他线程持有读取或写入锁时成功。lock_read():获取读取访问权限,可与其他读取锁或写入锁同时持有。unlock():释放写入访问权限。unlock_shared():释放读取访问权限。
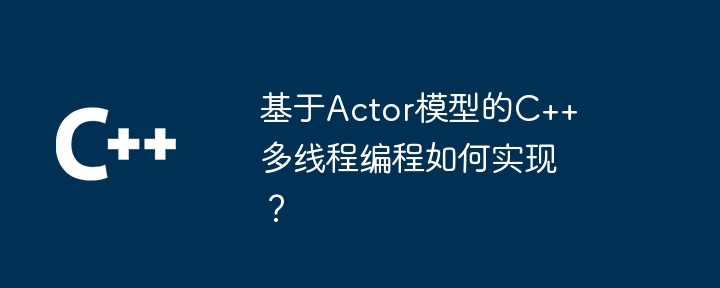
基于Actor模型的C++多线程编程实现:创建表示独立实体的Actor类。设置存储消息的消息队列。定义Actor从队列接收并处理消息的方法。创建Actor对象,启动线程来运行它们。通过消息队列发送消息到Actor。这种方法提供了高并发性、可扩展性和隔离性,非常适合需要处理大量并行任务的应用程序。
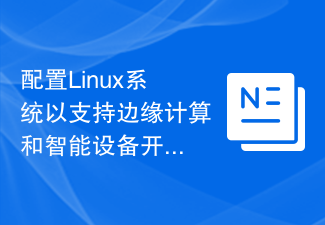
配置Linux系统以支持边缘计算和智能设备开发随着边缘计算和智能设备的快速发展,越来越多的开发者开始将注意力转向如何在Linux系统上进行边缘计算和智能设备开发。本文将介绍如何配置Linux系统以支持这两个方面的开发,并提供一些代码示例。一、安装Linux系统首先,我们需要选择适合边缘计算和智能设备开发的Linux发行版,例如Ubuntu或Debian。可以
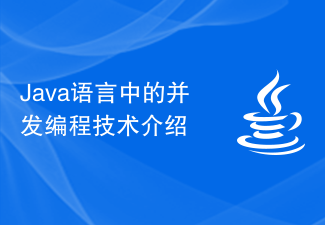
Java是一种广泛应用于开发各种程序的编程语言,它的并发编程技术受到广泛关注。随着多核处理器的普及和Web应用程序的开发,Java语言中并发编程的重要性愈加凸显。本文旨在介绍Java语言中的并发编程技术。1.什么是并发编程在计算机科学中,并发是指两个或多个独立的计算进程同时存在于计算机系统中的现象。并发编程是指设计和实现并发系统的程序技术,目的是解决多个任务


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
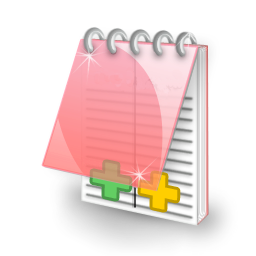
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
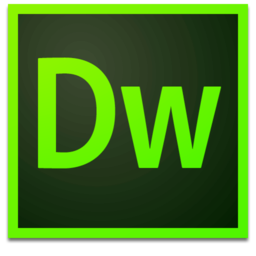
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
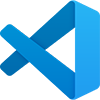
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
