


Second-hand recycling website developed using PHP supports popular tag navigation
The second-hand recycling website developed using PHP supports popular tag navigation
With the prosperity of second-hand transactions, second-hand recycling websites have become an indispensable part of people's daily lives. In order to improve the user experience and make it easier for users to find the items they are interested in, we need to add a function that supports popular tag navigation to the website. This article will use PHP as an example to introduce how to implement this function.
1. Create database and data table
First, we need to create a database and data table to store tag information. Open the MySQL management tool, create a database named "recycle", and create a data table named "tags" in the database. The structure of the data table is as follows:
CREATE TABLE `tags` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(50) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
2. Add tag data
Add some tag data to the database for subsequent use. You can add some sample data by executing the following SQL statement:
INSERT INTO `tags` (`name`) VALUES ('手机'); INSERT INTO `tags` (`name`) VALUES ('电脑'); INSERT INTO `tags` (`name`) VALUES ('服装'); INSERT INTO `tags` (`name`) VALUES ('家居'); INSERT INTO `tags` (`name`) VALUES ('书籍');
3. Page design
Next, we need to design a page to display popular tag navigation. The following is a simple example:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>二手回收网站</title> </head> <body> <h1 id="热门标签">热门标签</h1> <ul> <?php // 连接数据库 $conn = new mysqli("localhost", "root", "password", "recycle"); if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 查询标签数据 $sql = "SELECT * FROM tags"; $result = $conn->query($sql); // 输出标签列表 if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo "<li><a href='search.php?tag=".$row["name"]."'>".$row["name"]."</a></li>"; } } else { echo "暂无标签"; } // 关闭数据库连接 $conn->close(); ?> </ul> </body> </html>
4. Search page
In the tag navigation page, we add a link to each tag pointing to a search page, which is retrieved from the URL Get the tag parameter and use this parameter to search for items. The following is a simple example:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>搜索结果</title> </head> <body> <?php // 获取标签参数 $tag = $_GET["tag"]; // 连接数据库 $conn = new mysqli("localhost", "root", "password", "recycle"); if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 查询匹配标签的物品 $sql = "SELECT * FROM items WHERE tags LIKE '%".$tag."%'"; $result = $conn->query($sql); // 输出搜索结果 if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo "名称:" . $row["name"] . "<br>"; echo "价格:" . $row["price"] . "<br>"; echo "描述:" . $row["description"] . "<br><br>"; } } else { echo "未找到相关物品"; } // 关闭数据库连接 $conn->close(); ?> </body> </html>
The above is the implementation process of supporting popular tag navigation for a second-hand recycling website developed using PHP. By adding the popular tag navigation function, users can quickly find the items they are interested in, which improves the usability and user experience of the website. Of course, the above is just a simple example, you can expand and improve it according to your actual needs.
The above is the detailed content of Second-hand recycling website developed using PHP supports popular tag navigation. For more information, please follow other related articles on the PHP Chinese website!
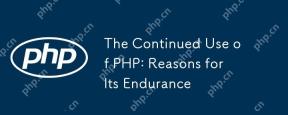
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
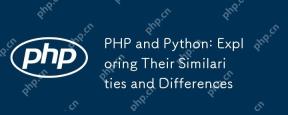
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
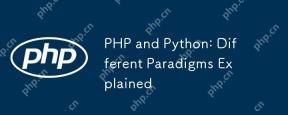
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
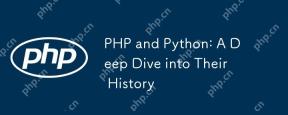
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
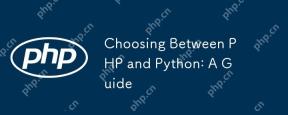
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
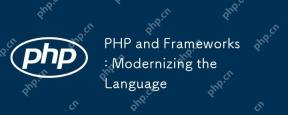
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
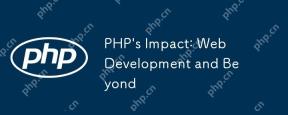
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
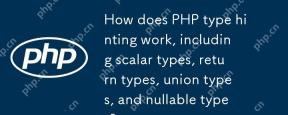
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.