


How to deal with concurrent data update synchronization exceptions in Java development
In Java development, sometimes we need multiple threads to operate shared data at the same time. However, concurrency problems can arise when multiple threads update shared data at the same time. For example, if one thread is reading shared data while another thread is writing at the same time, this can easily lead to data inconsistency or even unexpected exceptions.
In order to solve this problem, Java provides some mechanisms to handle update synchronization exceptions of concurrent data. We can use these mechanisms to ensure that operations on shared data between threads are safe and orderly.
1. Use the keyword synchronized
The keyword synchronized can be used to modify methods or code blocks. Its function is to ensure that only one thread can execute the modified method or code block at the same time. When a thread enters a method or code block modified by synchronized, it automatically acquires the object's lock, and other threads must wait for the thread to release the lock before they can continue execution. This ensures that operations on shared data by multiple threads are mutually exclusive, thereby avoiding concurrency problems.
For example, the following code demonstrates how to use the synchronized keyword to ensure thread safety:
public class Counter { private int count = 0; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } }
In this example, the increment() and getCount() methods in the Counter class are synchronized Modification, thus ensuring that operations on the count variable by multiple threads are mutually exclusive.
2. Use Lock lock
In addition to the synchronized keyword, Java also provides a more flexible lock mechanism-Lock lock. Lock is a synchronization mechanism in the Java.util.concurrent package that allows more fine-grained control of thread access to shared data.
Compared with the synchronized keyword, Lock has better scalability and flexibility. It provides more features such as reentrancy, conditional waits, and timeout waits. With Lock, we can more precisely control thread access to shared data, thereby reducing the occurrence of concurrency problems.
The following is a sample code for using Lock lock:
import java.util.concurrent.locks.Lock; import java.util.concurrent.locks.ReentrantLock; public class Counter { private int count = 0; private Lock lock = new ReentrantLock(); public void increment() { lock.lock(); try { count++; } finally { lock.unlock(); } } public int getCount() { lock.lock(); try { return count; } finally { lock.unlock(); } } }
In this example, we create a ReentrantLock object and use the lock() method to acquire the lock and the unlock() method to release the lock . By using Lock, we can control resource access more accurately and ensure thread safety.
3. Use thread-safe data structures
Another way to deal with the problem of concurrent data update synchronization exceptions is to use thread-safe data structures. Java provides many thread-safe data structures, such as Vector, Hashtable, ConcurrentHashMap, etc. These data structures are naturally thread-safe and can avoid concurrency issues.
For situations where data needs to be updated frequently, we can consider using thread-safe collection classes. For example, the ConcurrentHashMap class is provided in the Java.util.concurrent package, which is a thread-safe hash table implementation that can perform concurrent read and write operations in a high-concurrency environment.
The following is a sample code using the ConcurrentHashMap class:
import java.util.concurrent.ConcurrentHashMap; public class Counter { private ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>(); public void increment(String key) { map.putIfAbsent(key, 0); map.compute(key, (k, v) -> v + 1); } public int getCount(String key) { return map.getOrDefault(key, 0); } }
In this example, we use ConcurrentHashMap to store the counter, add key-value pairs through the putIfAbsent() method, and use the compute() method to Values are accumulated. Since ConcurrentHashMap is thread-safe, we don't need to worry about concurrency issues.
Summary:
In Java development, it is very important to deal with concurrent data update synchronization exceptions. We can use the keyword synchronized, Lock or thread-safe data structure to ensure thread safety. The keyword synchronized is suitable for simple situations, Lock is suitable for complex situations, and thread-safe data structures are suitable for situations where data is frequently updated. Reasonable selection of the appropriate mechanism can improve the concurrency performance of the program and avoid the occurrence of concurrency problems.
The above is the detailed content of How to deal with concurrent data update synchronization exceptions in Java development. For more information, please follow other related articles on the PHP Chinese website!
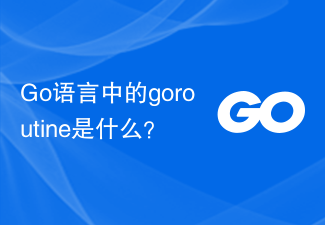
Go语言是一种开源编程语言,由Google开发并于2009年面世。这种语言在近年来越发受到关注,并被广泛用于开发网络服务、云计算等领域。Go语言最具特色的特点之一是它内置了goroutine(协程),这是一种轻量级的线程,可以在代码中方便地实现并发和并行计算。那么goroutine到底是什么呢?简单来说,goroutine就是Go语言中的
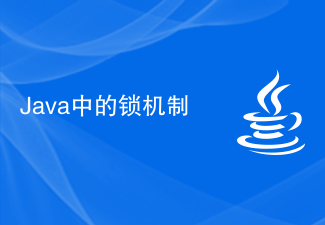
Java作为一种高级编程语言,在并发编程中有着广泛的应用。在多线程环境下,为了保证数据的正确性和一致性,Java采用了锁机制。本文将从锁的概念、类型、实现方式和使用场景等方面对Java中的锁机制进行探讨。一、锁的概念锁是一种同步机制,用于控制多个线程之间对共享资源的访问。在多线程环境下,线程的执行是并发的,多个线程可能会同时修改同一数据,这就会导致数
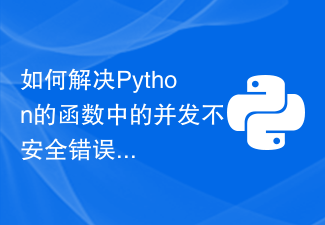
Python是一门流行的高级编程语言,它具有简单易懂的语法、丰富的标准库和开源社区的支持,而且还支持多种编程范式,例如面向对象编程、函数式编程等。尤其是Python在数据处理、机器学习、科学计算等领域有着广泛的应用。然而,在多线程或多进程编程中,Python也存在一些问题。其中之一就是并发不安全。本文将从以下几个方面介绍如何解决Python的函数中的并发不安
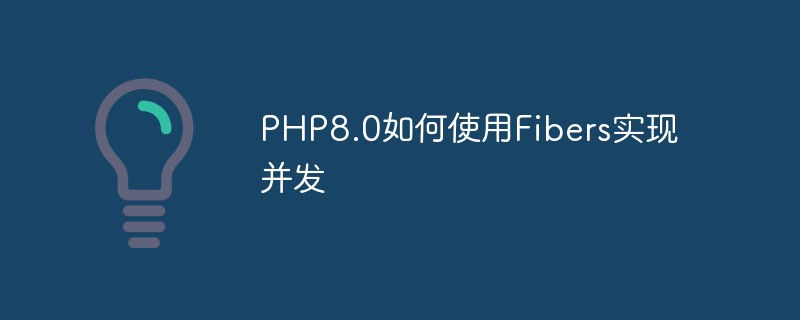
随着现代互联网技术的不断发展,网站访问量越来越大,对于服务器的并发处理能力也提出了更高的要求。如何提高服务器的并发处理能力是每个开发者需要面对的问题。在这个背景下,PHP8.0引入了Fibers这一全新的特性,让PHP开发者掌握一种全新的并发处理方式。Fibers是什么?首先,我们需要了解什么是Fibers。Fibers是一种轻量级的线程,可以高效地支持PH
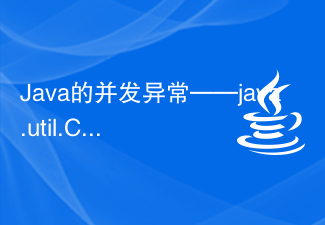
Java作为一种高级语言,在编程语言中使用广泛。在Java的应用程序和框架的开发中,我们经常会碰到并发的问题。并发问题是指当多个线程同时对同一个对象进行操作时,会产生一些意想不到的结果,这些问题称为并发问题。其中的一个常见的异常就是java.util.ConcurrentModificationException异常,那么我们在开发过程中如何有效地解决这个异
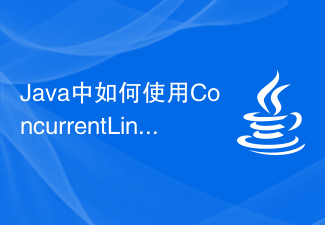
Java中的ConcurrentLinkedQueue函数为开发者提供了一种线程安全的、高效的队列实现方式,它支持并发读写操作,并且执行效率较高。在本文中,我们将介绍Java中如何使用ConcurrentLinkedQueue函数进行并发队列操作,帮助开发者更好地利用其优势。ConcurrentLinkedQueue是Java中的一个线程安全、非阻塞的队列实
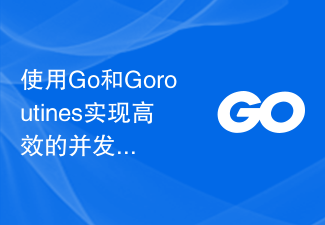
使用Go和Goroutines实现高效的并发图计算引言:随着大数据时代的到来,图计算问题也成为了一个热门的研究领域。在图计算中,图的顶点和边之间的关系非常复杂,因此如果采用传统的串行方法进行计算,往往会遇到性能瓶颈。为了提高计算效率,我们可以利用并发编程的方法使用多个线程同时进行计算。今天我将向大家介绍使用Go和Goroutines实现高效的并发图计算的方法
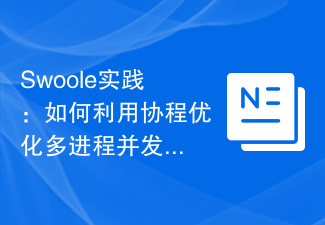
随着Web应用程序越来越复杂,访问并发处理和性能优化变得越来越重要。在许多情况下,使用多进程或线程处理并发请求是解决方案。然而,在这种情况下,需要考虑上下文切换和内存占用等问题。在本文中,我们将介绍如何使用Swoole和协程来优化多进程并发访问。Swoole是一个基于PHP的协程异步网络通信引擎,它允许我们非常方便地实现高性能的网络通信。Swoole协程简


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
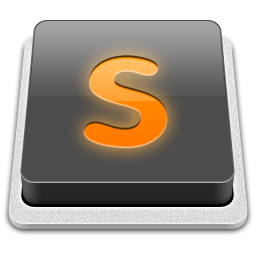
SublimeText3 Mac version
God-level code editing software (SublimeText3)
