


PHP Development Guide: How to implement user registration and login functions
In modern Web applications, user registration and login functions are indispensable basic functions. This article will introduce how to use PHP development to implement user registration and login functions, and attach corresponding code examples.
- Create database table
First, we need to create a user table to store registered user information. Suppose our table is named "user" and contains the following fields:
id - user ID (auto-incremented primary key)
username - username
password - password (encrypted and stored)
email - Email
The SQL statement to create the table is as follows:
CREATE TABLE `user` ( `id` INT(11) NOT NULL AUTO_INCREMENT, `username` VARCHAR(50) NOT NULL, `password` VARCHAR(50) NOT NULL, `email` VARCHAR(100) NOT NULL, PRIMARY KEY (`id`), UNIQUE KEY `username` (`username`), UNIQUE KEY `email` (`email`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
- Registration function implementation
Next, we implement the user registration function. After the user submits the registration form, we need to verify the legitimacy of the form data and insert the user name, password and email entered by the user into the database table.
PHP code example:
<?php // 连接数据库 $connection = mysqli_connect("localhost", "root", "password", "database"); if (!$connection) { die("数据库连接失败:" . mysqli_connect_error()); } // 获取用户提交的表单数据 $username = $_POST['username']; $password = $_POST['password']; $email = $_POST['email']; // 验证表单数据的合法性 // ... // 使用预处理语句插入数据到数据库 $stmt = $connection->prepare("INSERT INTO user (username, password, email) VALUES (?, ?, ?)"); $stmt->bind_param("sss", $username, $password, $email); $stmt->execute(); // 关闭数据库连接 $stmt->close(); $connection->close(); ?>
- Login function implementation
To implement the user login function, you need to verify whether the user name and password entered by the user match those saved in the database data. If the match is successful, the user login is successful; otherwise, the login is considered failed.
PHP code example:
<?php // 连接数据库 $connection = mysqli_connect("localhost", "root", "password", "database"); if (!$connection) { die("数据库连接失败:" . mysqli_connect_error()); } // 获取用户提交的表单数据 $username = $_POST['username']; $password = $_POST['password']; // 使用预处理语句查询数据库 $stmt = $connection->prepare("SELECT * FROM user WHERE username = ?"); $stmt->bind_param("s", $username); $stmt->execute(); $result = $stmt->get_result(); if ($result->num_rows == 1) { // 验证密码是否正确 $row = $result->fetch_assoc(); if (password_verify($password, $row['password'])) { // 登录成功 // ... } else { // 密码错误 // ... } } else { // 用户名不存在 // ... } // 关闭数据库连接 $stmt->close(); $connection->close(); ?>
Through the above code example, we can implement simple user registration and login functions. Of course, more security and user experience details may need to be considered in actual projects. I hope this article will be helpful to you in learning and practicing PHP development.
The above is the detailed content of PHP Development Guide: How to implement user registration and login functions. For more information, please follow other related articles on the PHP Chinese website!
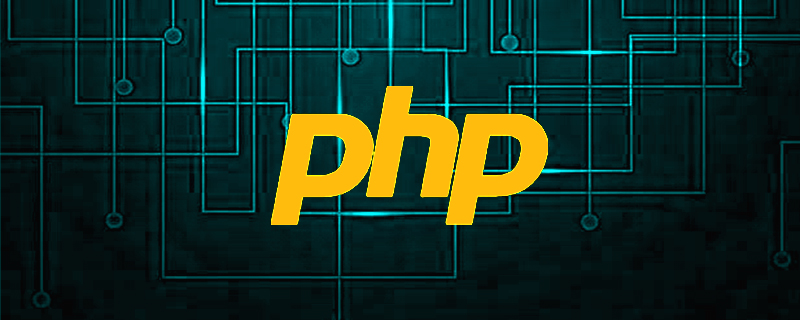
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
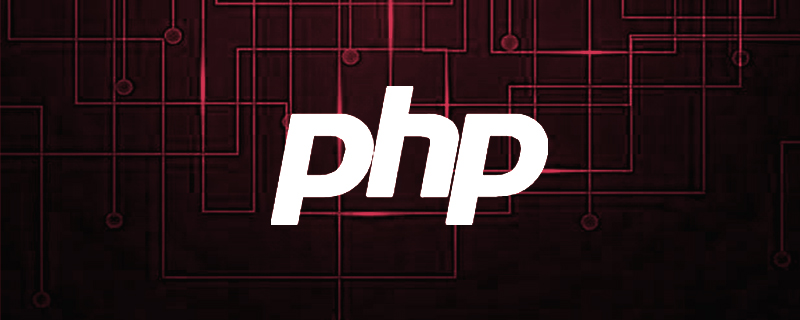
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
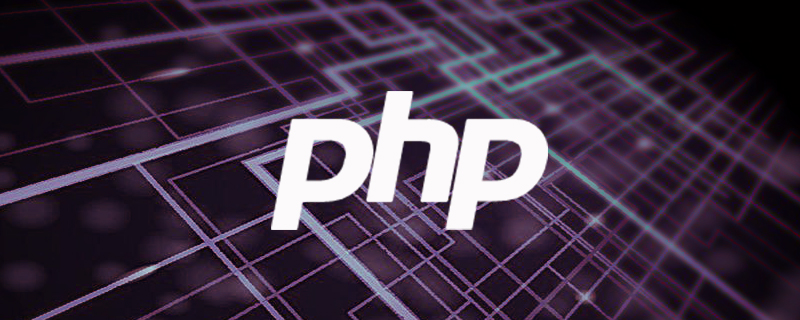
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
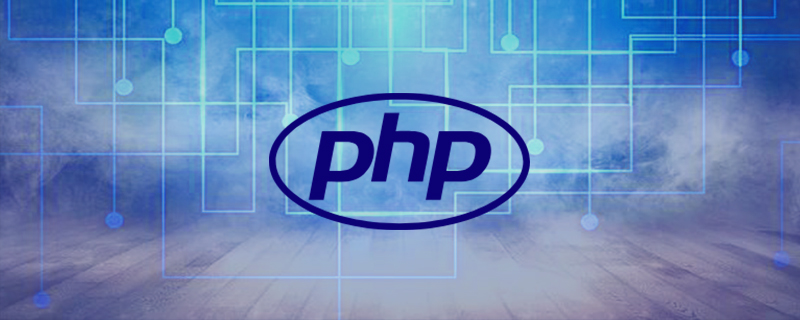
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
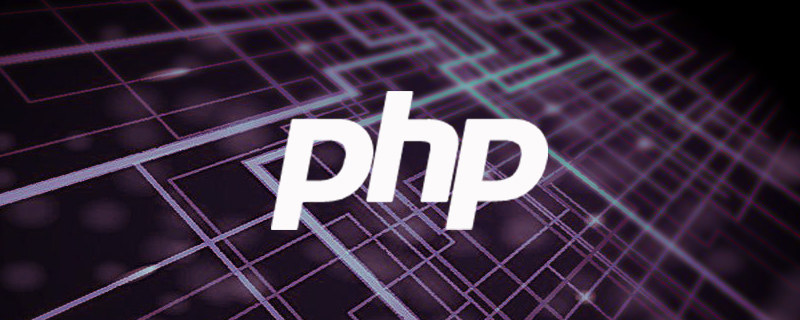
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
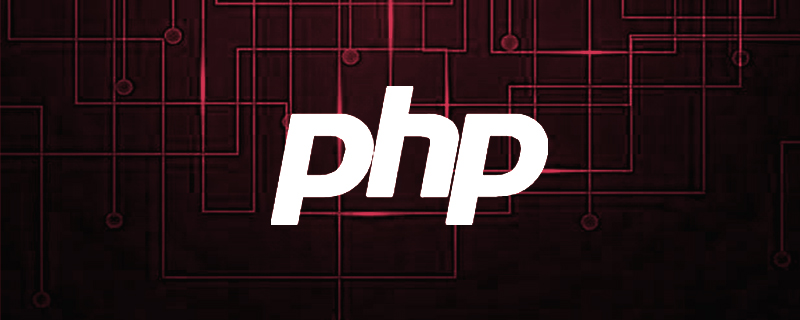
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
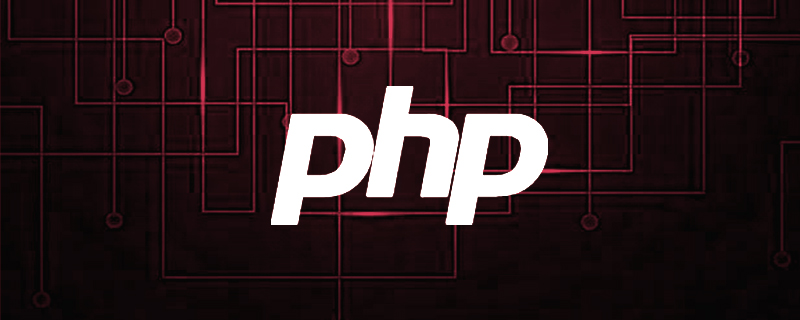
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
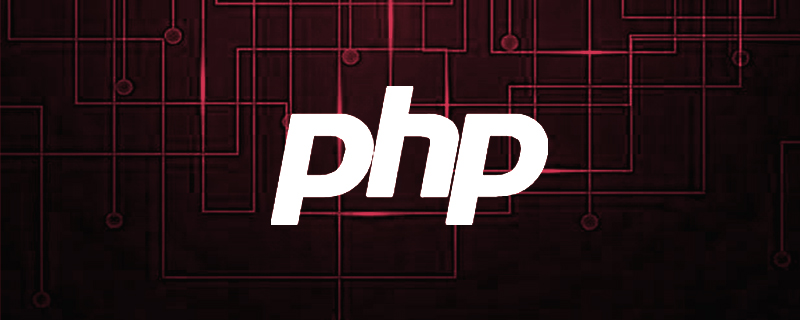
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
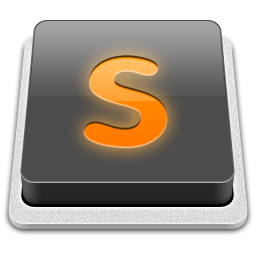
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
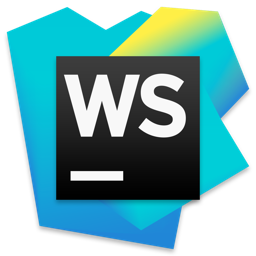
WebStorm Mac version
Useful JavaScript development tools
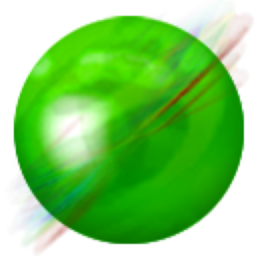
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.