


PHP development tips: How to use Gearman scheduled tasks to process MySQL database
PHP development skills: How to use Gearman scheduled tasks to process MySQL database
Introduction:
Gearman is an open source distributed task scheduling system that can be used to execute tasks in parallel and improve the processing capabilities of the system . In PHP development, we often use Gearman to handle some time-consuming tasks or asynchronous tasks. This article will introduce how to use Gearman to implement scheduled tasks to handle MySQL database operations.
1. Install Gearman
- In Linux systems, you can install Gearman directly through the package management tool. For example, on Ubuntu you can use the following command to install:
sudo apt-get install gearman-job-server sudo apt-get install php-gearman
- On Windows you can install Gearman through PECL. First, you need to install the PECL extension, and then use the following command:
pecl install gearman
- After the installation is complete, you need to add the Gearman extension configuration item in the php.ini file. For example:
extension=gearman.so
2. Create Gearman Worker
- Create a PHP file and name it worker.php. Write the following code in the file:
<?php $worker = new GearmanWorker(); $worker->addServer(); // 添加Gearman服务器信息 $worker->addFunction("mysql_query", "process_query"); // 添加Gearman任务处理函数 while ($worker->work()) { if ($worker->returnCode() != GEARMAN_SUCCESS) { echo "Worker failed: " . $worker->error() . " "; break; } } function process_query($job) { // 处理数据库操作的逻辑 $mysqli = new mysqli('localhost', 'username', 'password', 'database'); // 执行数据库操作 $result = $mysqli->query($job->workload()); $mysqli->close(); return $result; } ?>
- In the above code, a GearmanWorker object is first created, and the Gearman server information and task processing functions are added. In the task processing function, we can write specific MySQL database operation logic.
3. Create Gearman Client
- Create a PHP file and name it client.php. Write the following code in the file:
<?php $client = new GearmanClient(); $client->addServer(); // 添加Gearman服务器信息 $query = "SELECT * FROM table"; // 待处理的数据库查询语句 $client->doBackground("mysql_query", $query); // 发送Gearman任务 echo "Task sent to Gearman server. "; ?>
- In the above code, a GearmanClient object is first created and the Gearman server information is added. We then specify the database query to be processed and use the doBackground method to send the task to the Gearman server.
4. Run the program
- Run the worker.php file in the terminal and start the Gearman Worker:
php worker.php
- In the terminal Run the client.php file and send the task to the Gearman server:
php client.php
- At this time, the Gearman Worker will receive the task from the Gearman server and execute it to process the database operation.
Summary:
By using Gearman scheduled tasks to process the MySQL database, we can allocate some time-consuming database operations to different Workers for parallel processing, improving the system's processing capabilities and response speed. Gearman's flexibility and extensibility make it one of the most useful tools in PHP development.
Note: In actual development, timing tasks and database operation logic need to be reasonably designed and adjusted based on specific business needs and system architecture.
The above is the detailed content of PHP development tips: How to use Gearman scheduled tasks to process MySQL database. For more information, please follow other related articles on the PHP Chinese website!
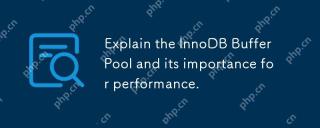
InnoDBBufferPool reduces disk I/O by caching data and indexing pages, improving database performance. Its working principle includes: 1. Data reading: Read data from BufferPool; 2. Data writing: After modifying the data, write to BufferPool and refresh it to disk regularly; 3. Cache management: Use the LRU algorithm to manage cache pages; 4. Reading mechanism: Load adjacent data pages in advance. By sizing the BufferPool and using multiple instances, database performance can be optimized.
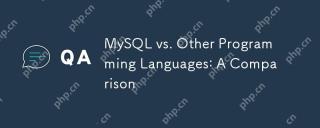
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
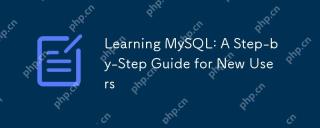
MySQL is worth learning because it is a powerful open source database management system suitable for data storage, management and analysis. 1) MySQL is a relational database that uses SQL to operate data and is suitable for structured data management. 2) The SQL language is the key to interacting with MySQL and supports CRUD operations. 3) The working principle of MySQL includes client/server architecture, storage engine and query optimizer. 4) Basic usage includes creating databases and tables, and advanced usage involves joining tables using JOIN. 5) Common errors include syntax errors and permission issues, and debugging skills include checking syntax and using EXPLAIN commands. 6) Performance optimization involves the use of indexes, optimization of SQL statements and regular maintenance of databases.
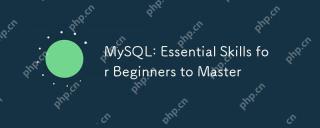
MySQL is suitable for beginners to learn database skills. 1. Install MySQL server and client tools. 2. Understand basic SQL queries, such as SELECT. 3. Master data operations: create tables, insert, update, and delete data. 4. Learn advanced skills: subquery and window functions. 5. Debugging and optimization: Check syntax, use indexes, avoid SELECT*, and use LIMIT.
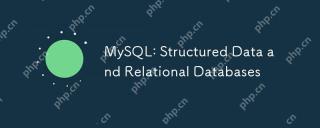
MySQL efficiently manages structured data through table structure and SQL query, and implements inter-table relationships through foreign keys. 1. Define the data format and type when creating a table. 2. Use foreign keys to establish relationships between tables. 3. Improve performance through indexing and query optimization. 4. Regularly backup and monitor databases to ensure data security and performance optimization.
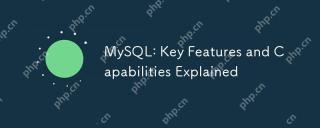
MySQL is an open source relational database management system that is widely used in Web development. Its key features include: 1. Supports multiple storage engines, such as InnoDB and MyISAM, suitable for different scenarios; 2. Provides master-slave replication functions to facilitate load balancing and data backup; 3. Improve query efficiency through query optimization and index use.
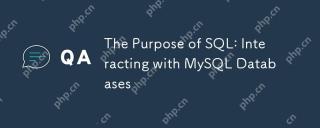
SQL is used to interact with MySQL database to realize data addition, deletion, modification, inspection and database design. 1) SQL performs data operations through SELECT, INSERT, UPDATE, DELETE statements; 2) Use CREATE, ALTER, DROP statements for database design and management; 3) Complex queries and data analysis are implemented through SQL to improve business decision-making efficiency.
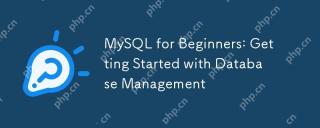
The basic operations of MySQL include creating databases, tables, and using SQL to perform CRUD operations on data. 1. Create a database: CREATEDATABASEmy_first_db; 2. Create a table: CREATETABLEbooks(idINTAUTO_INCREMENTPRIMARYKEY, titleVARCHAR(100)NOTNULL, authorVARCHAR(100)NOTNULL, published_yearINT); 3. Insert data: INSERTINTObooks(title, author, published_year)VA


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
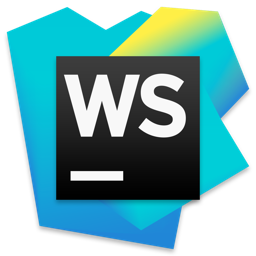
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment