Use PHP to build the sales module function in the ERP system
Use PHP to build the sales module function in the ERP system
Introduction:
Enterprise resource planning (ERP) systems are widely used to manage all aspects of enterprises, of which the sales module function is one of them. The functions of the sales module involve sales order processing, customer management, inventory management, and the generation of related reports. In this article, we will explore how to code a sales module functionality using PHP.
Sales order processing:
In the ERP system, sales orders are one of the core functions in the sales module. The following is a simple PHP code example for creating and processing sales orders:
<?php // 创建销售订单 function createSalesOrder($customerName, $product, $quantity) { // 在数据库中插入订单数据 $sql = "INSERT INTO sales_orders (customer_name, product, quantity) VALUES ('$customerName', '$product', $quantity)"; mysqli_query($conn, $sql); // 更新库存数量 updateStock($product, $quantity); // 生成订单号 $orderId = generateOrderId(); return $orderId; } // 更新库存数量 function updateStock($product, $quantity) { // 查找当前库存数量 $sql = "SELECT quantity FROM stock WHERE product = '$product'"; $result = mysqli_query($conn, $sql); $row = mysqli_fetch_assoc($result); // 更新库存数量 $newQuantity = $row['quantity'] - $quantity; $sql = "UPDATE stock SET quantity = $newQuantity WHERE product = '$product'"; mysqli_query($conn, $sql); } // 生成订单号 function generateOrderId() { // 可以根据一定的规则生成订单号 $orderId = uniqid(); return $orderId; } // 示例用法 $customerName = "John Doe"; $product = "Example Product"; $quantity = 10; $orderId = createSalesOrder($customerName, $product, $quantity); echo "销售订单已成功创建,订单号为:$orderId"; ?>
Customer Management:
In the sales module, customer management is a very important part. The following is a sample code for creating and managing customer information:
<?php // 创建客户 function createCustomer($name, $email, $phone) { // 在数据库中插入客户数据 $sql = "INSERT INTO customers (name, email, phone) VALUES ('$name', '$email', '$phone')"; mysqli_query($conn, $sql); // 返回新创建客户的ID $customerId = mysqli_insert_id($conn); return $customerId; } // 更新客户信息 function updateCustomer($customerId, $name, $email, $phone) { // 更新数据库中客户数据 $sql = "UPDATE customers SET name = '$name', email = '$email', phone = '$phone' WHERE id = $customerId"; mysqli_query($conn, $sql); } // 删除客户 function deleteCustomer($customerId) { // 从数据库中删除客户数据 $sql = "DELETE FROM customers WHERE id = $customerId"; mysqli_query($conn, $sql); } // 示例用法 $name = "John Doe"; $email = "johndoe@example.com"; $phone = "1234567890"; $customerId = createCustomer($name, $email, $phone); echo "新客户已创建,客户ID为:$customerId"; ?>
Inventory Management:
Inventory management is a very important function in the ERP system. The following is a sample code for managing inventory quantities:
<?php // 查询库存数量 function getStockQuantity($product) { // 查询数据库中当前库存数量 $sql = "SELECT quantity FROM stock WHERE product = '$product'"; $result = mysqli_query($conn, $sql); $row = mysqli_fetch_assoc($result); return $row['quantity']; } // 示例用法 $product = "Example Product"; $quantity = getStockQuantity($product); echo "产品 '$product' 的库存数量为:$quantity"; ?>
Report generation:
In the ERP system, sales reports are the key to helping enterprises manage sales. The following is a sample code for generating a sales report:
<?php // 生成销售报表 function generateSalesReport($startDate, $endDate) { // 查询数据库中的销售订单数据 $sql = "SELECT * FROM sales_orders WHERE order_date BETWEEN '$startDate' AND '$endDate'"; $result = mysqli_query($conn, $sql); // 处理查询结果并生成报表 $salesReport = array(); while ($row = mysqli_fetch_assoc($result)) { $salesReport[] = array( 'order_id' => $row['order_id'], 'customer_name' => $row['customer_name'], 'product' => $row['product'], 'quantity' => $row['quantity'] ); } return $salesReport; } // 示例用法 $startDate = "2022-01-01"; $endDate = "2022-01-31"; $salesReport = generateSalesReport($startDate, $endDate); echo "销售报表如下:"; var_dump($salesReport); ?>
Conclusion:
This article introduces a code example for writing sales module functions using PHP. These examples cover functionality such as sales order processing, customer management, inventory management, and report generation. By using these sample codes, you can better understand and implement the related functions of the sales module in the ERP system. Of course, these examples only provide a basic framework, and more logic and security considerations may be required in actual production environments. I hope this article will be helpful to everyone in building the sales module function in the ERP system.
The above is the detailed content of Use PHP to build the sales module function in the ERP system. For more information, please follow other related articles on the PHP Chinese website!
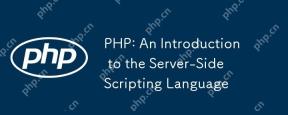
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
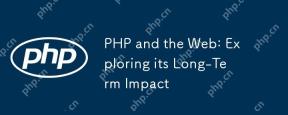
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
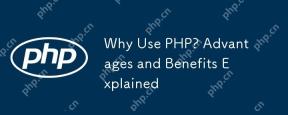
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
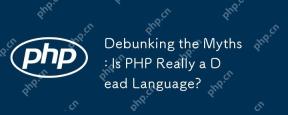
PHP is not dead. 1) The PHP community actively solves performance and security issues, and PHP7.x improves performance. 2) PHP is suitable for modern web development and is widely used in large websites. 3) PHP is easy to learn and the server performs well, but the type system is not as strict as static languages. 4) PHP is still important in the fields of content management and e-commerce, and the ecosystem continues to evolve. 5) Optimize performance through OPcache and APC, and use OOP and design patterns to improve code quality.
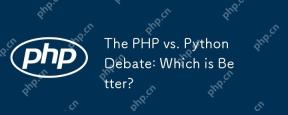
PHP and Python have their own advantages and disadvantages, and the choice depends on the project requirements. 1) PHP is suitable for web development, easy to learn, rich community resources, but the syntax is not modern enough, and performance and security need to be paid attention to. 2) Python is suitable for data science and machine learning, with concise syntax and easy to learn, but there are bottlenecks in execution speed and memory management.
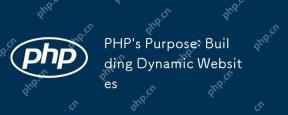
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
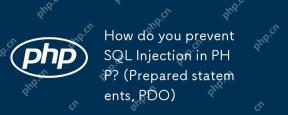
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
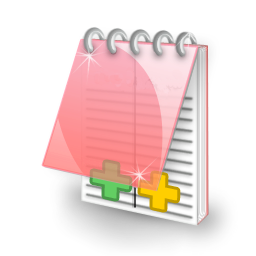
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
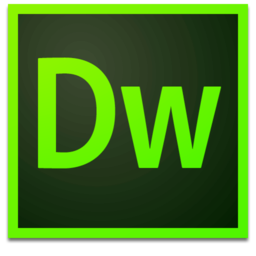
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor