How to handle pop-up confirmation boxes in Vue development
How to deal with the pop-up confirmation box problem encountered in Vue development
Introduction:
In Vue development, the pop-up confirmation box is a common functional requirement. When users perform some key operations, such as deleting data, submitting forms, etc., we often need to pop up a confirmation box to allow users to confirm that the operation is meaningful and prevent misoperations. This article will introduce how to deal with pop-up confirmation box problems encountered in Vue development.
1. Use the MessageBox component in the element-ui component library
element-ui is a Vue-based UI component library that provides a wealth of components for us to use in Vue. Among them, the MessageBox component can easily implement the function of a pop-up confirmation box.
The steps are as follows:
- Install element-ui: Introduce the element-ui component library into the project, and configure and install it according to the official documentation.
- In the component that needs to use the pop-up window confirmation box, introduce the MessageBox component:
import { MessageBox } from 'element-ui'; - In the event that needs to trigger the pop-up window, Call the MessageBox.confirm method:
MessageBox.confirm('Are you sure you want to perform this operation?', 'Prompt', {
confirmButtonText: 'OK',
cancelButtonText: 'Cancel',
type : 'warning'
}).then(() => {
// The user clicked the confirmation button and performed the confirmation operation
}).catch(() => {
/ / The user clicks the cancel button and performs the cancel operation
});
In the above code, the MessageBox.confirm method accepts three parameters, namely the pop-up window content, pop-up window title and configuration items . After the user clicks the confirm button, the callback function in then will be executed; after the user clicks the cancel button, the callback function in catch will be executed.
2. Customized pop-up confirmation box component
Sometimes, we may need to customize the style and interactive effect of the pop-up confirmation box according to business needs. At this time, we can customize a pop-up confirmation box component and call it where we need to use it.
The steps are as follows:
-
Create a component named ConfirmDialog:
<div class="content">{{ content }}</div> <div class="buttons"> <button @click="confirm">确定</button> <button @click="cancel">取消</button> </div>
<script><br>export default {<br> props: ['content'],<br> methods: {</script>
confirm() { // 用户点击了确认按钮,执行确认操作 this.$emit('confirm'); }, cancel() { // 用户点击了取消按钮,执行取消操作 this.$emit('cancel'); }
}
} -
Use the ConfirmDialog component in the parent component:
<button @click="showConfirmDialog">点击确认按钮</button> <ConfirmDialog v-if="showDialog" :content="dialogContent" @confirm="handleConfirm" @cancel="handleCancel" />
<script><br>import ConfirmDialog from './components/ConfirmDialog';</script>
export default {
components: {ConfirmDialog
},
data() {return { showDialog: false, dialogContent: '' }
},
methods: {showConfirmDialog() { this.showDialog = true; this.dialogContent = '确定要执行此操作吗?'; }, handleConfirm() { // 用户点击了确认按钮,执行确认操作 this.showDialog = false; }, handleCancel() { // 用户点击了取消按钮,执行取消操作 this.showDialog = false; }
}
}
In the above code, we use a showDialog variable to control whether to display the pop-up window. When the confirm button is clicked, we execute the handleConfirm method; when the cancel button is clicked, we execute the handleCancel method.
Summary:
This article introduces two methods to deal with pop-up confirmation box problems encountered in Vue development. Using element-ui's MessageBox component can easily implement the pop-up confirmation box function, while customizing the pop-up confirmation box component can meet business needs more flexibly. In actual development, we can choose the appropriate method to deal with the pop-up confirmation box problem according to the specific situation.
The above is the detailed content of How to handle pop-up confirmation boxes in Vue development. For more information, please follow other related articles on the PHP Chinese website!
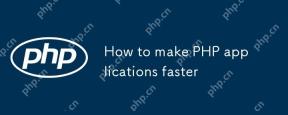
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
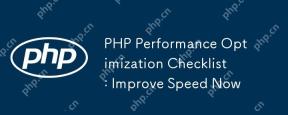
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
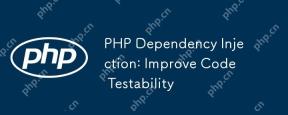
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
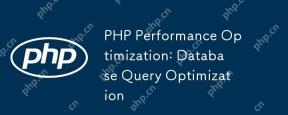
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
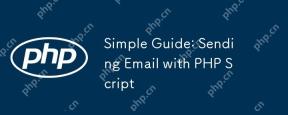
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
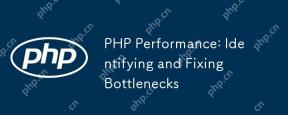
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
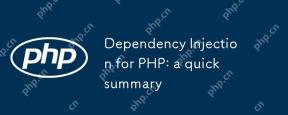
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
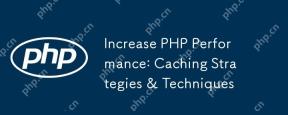
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
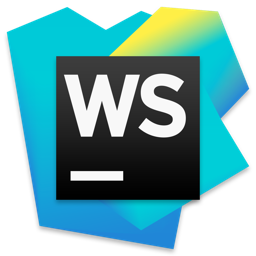
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
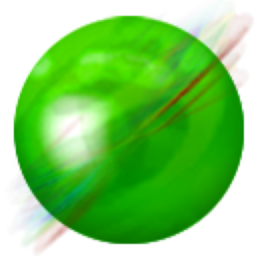
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
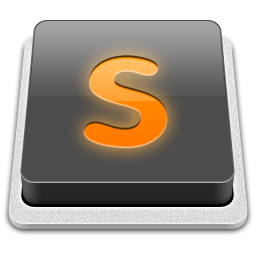
SublimeText3 Mac version
God-level code editing software (SublimeText3)
