


How to implement high-performance concurrent queues in Go language development
How to implement high-performance concurrent queues in Go language development
Introduction:
With the development of applications and the increase in demand, the need for high-performance concurrent queues is becoming more and more urgent. . As a language with high concurrency characteristics, Go language provides some powerful tools and mechanisms to implement high-performance concurrent queues. This article will explore how to use the Go language to implement a high-performance concurrent queue.
1. Background
In concurrent programming, queue is a commonly used data structure, which can be used to store and process a series of tasks or messages to be processed. For high-performance concurrent queues, its main indicators include the following aspects:
- High throughput: The queue should be able to efficiently handle a large number of tasks or messages.
- Low latency: The queue should be able to process each task or message quickly.
- Concurrency safety: The queue should be able to safely share and process data between multiple goroutines.
2. Design principles
When designing a high-performance concurrent queue, we can design it based on the following principles:
- Lock-free design: Using a lock-free design can improve performance by avoiding lock contention in concurrent operations.
- Collaborative design: Using coroutines allows multiple goroutines to process tasks concurrently, improving concurrency performance.
- Buffer design: Using buffers can improve the processing speed of tasks and decouple the processing speed of producers and consumers.
- Based on channel communication: Using go's channel mechanism can facilitate communication and synchronization between goroutines.
3. Implementation steps
Below we will gradually introduce the implementation of a high-performance concurrent queue based on the above design principles:
- Define the task structure: First we need to define a task structure, which contains the specific content and processing logic of the task. For example:
type Task struct {
// 任务内容 Data interface{} // 处理逻辑 HandleFunc func(interface{})
}
- Create a queue structure: Create a queue structure that contains a task queue, and Some control variables for concurrent processing. For example:
type ConcurrentQueue struct {
// 任务队列 tasks chan Task // 结束信号量 exitChan chan struct{} // 等待组 wg sync.WaitGroup
}
- Add task: Add the Add method in the queue structure to add tasks to the queue . This method can directly add the task to the task queue.
func (q *ConcurrentQueue) Add(task Task) {
q.tasks <- task
}
- Concurrent processing tasks: Add the Start method in the queue structure, Used to process tasks concurrently.
func (q *ConcurrentQueue) Start(concurrency int) {
for i := 0; i < concurrency; i++ { go func() { defer q.wg.Done() for { select { case task := <-q.tasks: task.HandleFunc(task.Data) case <-q.exitChan: return } } }() } q.wg.Wait()
}
- Initialization and exit: Add Init and Stop to the queue structure Methods, respectively used to initialize the queue and stop the work of the queue.
func (q *ConcurrentQueue) Init() {
q.tasks = make(chan Task) q.exitChan = make(chan struct{})
}
func (q *ConcurrentQueue) Stop() {
close(q.exitChan)
}
4. Usage Example
The following is a usage example that shows how to use the high-performance concurrent queue implemented above:
func main() {
// 创建并发队列 queue := ConcurrentQueue{} queue.Init() // 向队列中添加任务 queue.Add(Task{ Data: 1, HandleFunc: func(data interface{}) { fmt.Println(data) time.Sleep(time.Second) }, }) queue.Add(Task{ Data: 2, HandleFunc: func(data interface{}) { fmt.Println(data) time.Sleep(time.Second) }, }) // 启动队列并发处理任务 queue.Start(3) // 停止队列 queue.Stop()
}
5. Summary
In this article, we introduced how to use the Go language to implement a high-performance concurrent queue. By using lock-free design, collaborative design, buffer design and channel-based communication mechanism, we can achieve a high-throughput, low-latency concurrent queue. I hope this article can inspire Go language developers and enable them to continuously optimize and improve in practice.
The above is the detailed content of How to implement high-performance concurrent queues in Go language development. For more information, please follow other related articles on the PHP Chinese website!
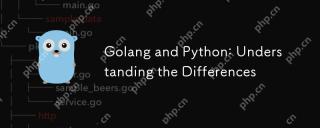
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
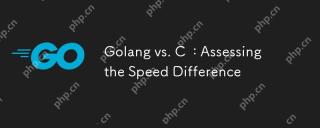
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
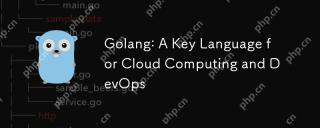
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
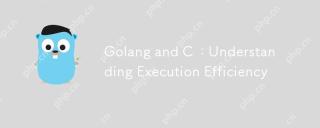
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
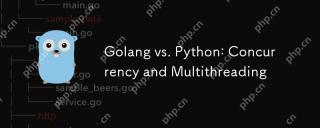
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
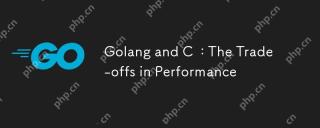
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
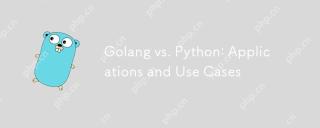
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
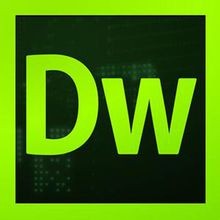
Dreamweaver CS6
Visual web development tools