Catching Panics in Go
In Golang, panics are exceptional conditions that can cause abnormal program termination. By default, panics halt execution, displaying a stack trace. However, it is possible to "catch" these panics and handle them gracefully.
Scenario:
Consider the following code:
package main import ( "fmt" "os" ) func main() { file, err := os.Open(os.Args[1]) if err != nil { fmt.Println("Could not open file") } fmt.Printf("%s", file) }
If no file argument is provided, a panic is thrown at line 9: "panic: runtime error: index out of range."
Solution:
To catch this panic, we can utilize the recover() function. recover() allows a goroutine to recover from a panic and return the value passed to the panic call.
package main import ( "fmt" "os" ) func main() { defer func() { if err := recover(); err != nil { fmt.Println("Error:", err) } }() file, err := os.Open(os.Args[1]) if err != nil { panic(err) } fmt.Printf("%s", file) }
With this modification, the code can now catch the panic and handle it by printing an error message.
Caveat:
Panicking is not an ideal solution for all error handling scenarios. Go's design philosophy emphasizes explicit error checking rather than relying on panics. However, the recover() mechanism provides a way to capture unexpected panics and perform cleanup operations.
The above is the detailed content of How Can I Catch and Handle Panics in Go?. For more information, please follow other related articles on the PHP Chinese website!
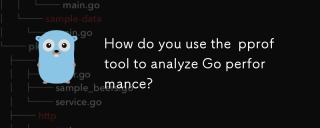
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
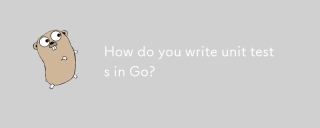
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
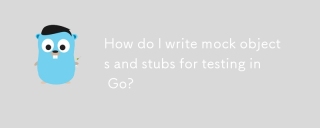
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
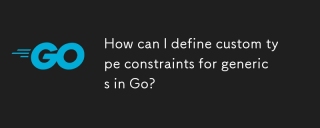
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
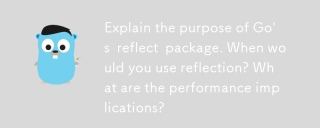
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
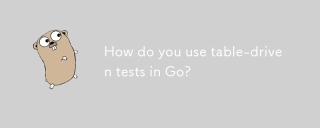
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
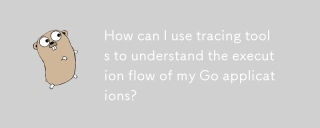
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
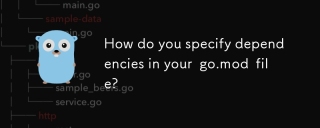
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
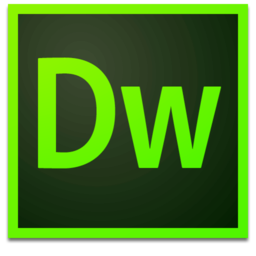
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
