How to solve network programming problems encountered in Java
How to solve network programming problems encountered in Java
In recent years, network programming has become more and more important in the field of Java development. With the rapid development of the Internet, the demand for network applications is also increasing. However, network programming often encounters some problems, such as connection timeout, inaccurate request response, etc. This article will introduce some methods to solve network programming problems encountered in Java.
1. Handling connection timeout
In network programming, connection timeout is a common problem. When we try to connect to a server, if the connection times out, we need to take appropriate measures. Java provides a simple and effective solution, which is to use the setSoTimeout method of Socket to set the timeout.
try { Socket socket = new Socket(); socket.connect(new InetSocketAddress("ip地址", 端口号), 超时时间); } catch (IOException e) { // 处理连接超时的异常 }
After setting the connection timeout, when the connection times out, a SocketTimeoutException will be thrown. We can add corresponding processing logic in the exception handling code block, such as retrying the connection or prompting the user for a connection timeout.
2. Solve the problem of inaccurate request response
In network programming, it is often necessary to interact with requests and responses. Sometimes, we may encounter request and response inaccuracies, where the response is not received correctly after sending a request. This is usually caused by network delays or data transmission errors.
To solve this problem, we can use the HttpURLConnection class in Java to send requests and receive responses. The HttpURLConnection class provides a series of methods to handle network requests and responses. You can refer to the following sample code:
try { URL url = new URL("请求的URL"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("GET"); // 设置请求方法 connection.setConnectTimeout(5000); // 设置连接超时时间 connection.setReadTimeout(5000); // 设置读取超时时间 // 发送请求并接收响应 int responseCode = connection.getResponseCode(); if (responseCode == HttpURLConnection.HTTP_OK) { // 处理响应数据 } else { // 处理响应错误 } } catch (IOException e) { // 处理异常 }
By using HttpURLConnection to send requests and receive responses, we can set the connection timeout and read timeout, thus Avoid the problem of inaccurate requests and responses.
3. Problems with handling concurrent requests
In actual development, we often need to send multiple concurrent requests at the same time. However, the processing of network requests is blocking, that is, each request must wait for the previous request to complete before it can start. This may result in long response times for requests, affecting application performance.
In order to improve the efficiency of concurrent requests, we can use Java's thread pool technology. The thread pool can handle multiple concurrent requests with limited thread resources, thereby improving the concurrent performance of network requests.
The following is a sample code for using the thread pool to implement concurrent requests:
ExecutorService executorService = Executors.newFixedThreadPool(10); // 创建线程池 // 提交多个任务 List<Future<Object>> futures = new ArrayList<>(); for (int i = 0; i < 10; i++) { Future<Object> future = executorService.submit(new Callable<Object>() { @Override public Object call() throws Exception { // 处理网络请求 return null; } }); futures.add(future); } // 处理任务的返回结果 for (Future<Object> future : futures) { try { Object result = future.get(); // 处理任务的返回结果 } catch (Exception e) { // 处理异常 } } executorService.shutdown(); // 关闭线程池
By using the thread pool, we can execute multiple network requests at the same time to improve the efficiency of concurrent requests.
Summary:
This article introduces some methods to solve network programming problems encountered in Java, including handling connection timeouts, solving the problem of inaccurate request responses, and handling concurrent requests. In actual development, we often encounter various network programming problems. By learning and mastering these solutions, we can improve the performance and stability of network applications. I hope this article will be helpful to readers when solving network programming problems.
The above is the detailed content of How to solve network programming problems encountered in Java. For more information, please follow other related articles on the PHP Chinese website!
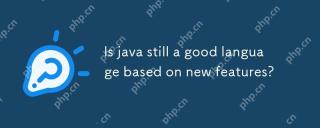
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
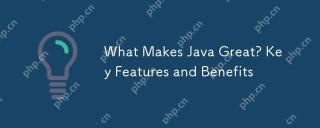
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
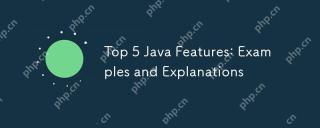
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.
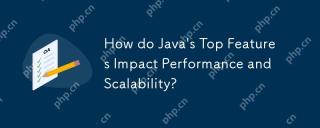
Java'stopfeaturessignificantlyenhanceitsperformanceandscalability.1)Object-orientedprincipleslikepolymorphismenableflexibleandscalablecode.2)Garbagecollectionautomatesmemorymanagementbutcancauselatencyissues.3)TheJITcompilerboostsexecutionspeedafteri
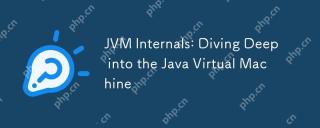
The core components of the JVM include ClassLoader, RuntimeDataArea and ExecutionEngine. 1) ClassLoader is responsible for loading, linking and initializing classes and interfaces. 2) RuntimeDataArea contains MethodArea, Heap, Stack, PCRegister and NativeMethodStacks. 3) ExecutionEngine is composed of Interpreter, JITCompiler and GarbageCollector, responsible for the execution and optimization of bytecode.
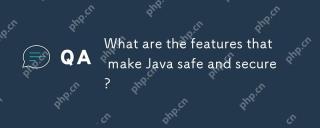
Java'ssafetyandsecurityarebolsteredby:1)strongtyping,whichpreventstype-relatederrors;2)automaticmemorymanagementviagarbagecollection,reducingmemory-relatedvulnerabilities;3)sandboxing,isolatingcodefromthesystem;and4)robustexceptionhandling,ensuringgr
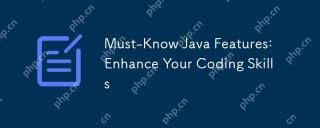
Javaoffersseveralkeyfeaturesthatenhancecodingskills:1)Object-orientedprogrammingallowsmodelingreal-worldentities,exemplifiedbypolymorphism.2)Exceptionhandlingprovidesrobusterrormanagement.3)Lambdaexpressionssimplifyoperations,improvingcodereadability
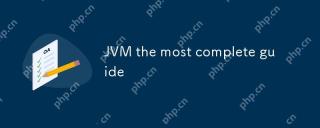
TheJVMisacrucialcomponentthatrunsJavacodebytranslatingitintomachine-specificinstructions,impactingperformance,security,andportability.1)TheClassLoaderloads,links,andinitializesclasses.2)TheExecutionEngineexecutesbytecodeintomachineinstructions.3)Memo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
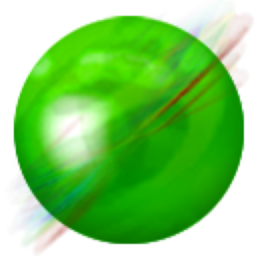
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version
