


How PHP implements full-text search function and provides convenient information search
In modern network application development, full-text search function has become an indispensable part. As a language widely used to develop web applications, PHP naturally provides some powerful libraries to support full-text search. In this article, we will delve into how to use PHP to implement full-text search functionality, and provide some tips to make your information search easier.
1. What is full-text search?
Full-text search refers to the ability to retrieve a certain keyword or phrase in a document. Traditional search engines usually simply match keywords without considering the context and association of words. Full-text search technology will analyze the relevance of keywords from multiple aspects and provide more accurate search results. Full-text search can usually be performed in large databases. It takes advantage of the characteristics of large amounts of text data to quickly find documents related to the keywords entered by the user.
2. Use PHP to implement full-text search function
PHP provides some built-in full-text search functions and methods. For small websites, it is sufficient to use these functions and methods for full-text search. But for large projects, you need to use more professional full-text search libraries, such as Solr and Elasticsearch.
- Use built-in functions and methods
(1) strpos() function
The strpos() function can check a certain string in a string The location where it appears. Use this function to build a simple full-text search function. Here is an example:
<?php $text = "This is an example text"; $pos = strpos($text, "example"); if ($pos !== false) { echo "Word found!"; } else { echo "Word not found!"; } ?>
The above code will check whether a string contains a certain string. If it exists, it will print "Word found!"; if it does not exist, it will print "Word not found!". The problem with this function is that it can only find the location where the specified string appears, but cannot find related words. For example, if the user enters "text example", this function cannot find them.
(2) preg_match() function
The preg_match() function can use regular expressions to find a pattern. This function is more powerful than strpos(), can find a certain word, and supports fuzzy matching and ignoring case. The following is an example:
<?php $text = "This is an example text"; $pattern = "/example/i"; if (preg_match($pattern, $text)) { echo "Word found!"; } else { echo "Word not found!"; } ?>
The above example uses regular expressions to find the string "example" in the string, where "/i" means case insensitivity. If the search is successful, "Word found!" will be output; if not found, "Word not found!" will be output.
- Full-text search using Solr
Solr is a high-performance, open source full-text search engine based on Lucene. Its search efficiency is very high and can support high concurrency, large data volume and fast response. Solr can be searched using an HTTP interface, which means you can use any language to interact with it. PHP has a good Solr client library - Solarium, which can help you simplify your work with Solr.
The following is an example of full-text search using Solarium:
<?php // include the Solarium autoloader require_once('vendor/autoload.php'); // create a client instance $client = new SolariumClient([ 'endpoint' => [ 'localhost' => [ 'host' => '127.0.0.1', 'port' => 8983, 'path' => '/solr/', 'core' => 'mycore' ] ] ]); // create a select query $query = $client->createSelect(); $query->setQuery('title:example'); // execute the query $resultset = $client->execute($query); // show the results echo 'Number of results: '.$resultset->getNumFound(); foreach ($resultset as $document) { echo '<hr/><table>'; foreach ($document as $field => $value) { echo '<tr><th>' . $field . '</th><td>' . $value . '</td></tr>'; } echo '</table>'; } ?>
The above example uses the Solarium client library. It first creates a client instance, then creates a SELECT query and sets the query conditions. Finally, it executes the query and outputs the results.
- Full-text search using Elasticsearch
Elasticsearch is an open source full-text search engine built on Lucene. Elasticsearch can be searched and managed through a RESTful API. There is also a good Elasticsearch client library in PHP - Elasticsearch-PHP, which can help you interact with Elasticsearch.
The following is an example of using Elasticsearch-PHP for full-text search:
<?php // include the Elasticsearch-PHP autoloader require_once('vendor/autoload.php'); // create a client instance $client = ElasticsearchClientBuilder::create() ->setHosts(['http://localhost:9200']) ->build(); // search documents $params = [ 'index' => 'myindex', 'type' => 'mytype', 'body' => [ 'query' => [ 'match' => [ 'title' => 'example' ] ] ] ]; $response = $client->search($params); // show the results echo 'Number of results: '.$response['hits']['total']; foreach ($response['hits']['hits'] as $hit) { foreach ($hit['_source'] as $field => $value) { echo '<hr/>'.$field.': '.$value; } } ?>
The above example uses the Elasticsearch-PHP client library. It first creates a client instance and then uses query statements to search for documents. Finally, it outputs the search results.
3. Improve the efficiency of full-text search
When your website becomes larger, the efficiency of full-text search may become a problem. Here are some tips to help you improve the efficiency of full-text search:
- Use indexes
For large data sets, full-text search requires a lot of resources and time. To speed up searches, you can use an index to maintain keywords and their location in the document. When making a query, you only need to search in the index rather than in the original data, which can greatly speed up the search.
- Storing data
The way you store data will affect the speed of full-text search. For example, using local files to store data is faster than using a database to store data because it avoids database connection overhead and SQL parsing overhead.
- Optimized search algorithm
Optimized search algorithm can help you get search results quickly. For example, using an inverted index can greatly simplify search operations because it can look for just one word in a keyword list instead of checking all words.
4. Summary
Full-text search is an indispensable part of modern network development. PHP provides many powerful libraries to support full-text search, such as Solr and Elasticsearch. Using these libraries can help you quickly build efficient full-text search capabilities. In addition, you can also use some tips to improve the efficiency of full-text search, such as using indexes, optimizing search algorithms, etc.
The above is the detailed content of How PHP implements full-text search function and provides convenient information search. For more information, please follow other related articles on the PHP Chinese website!
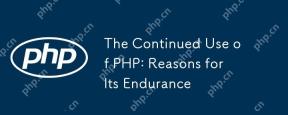
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
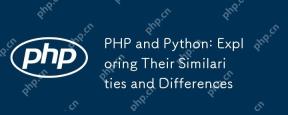
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
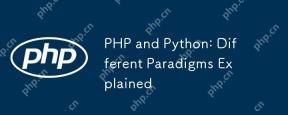
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
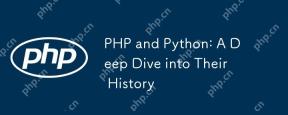
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
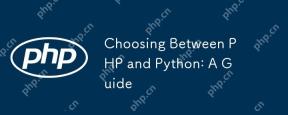
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
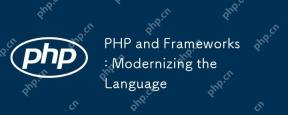
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
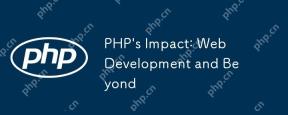
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
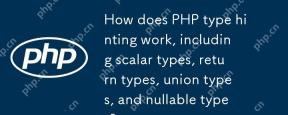
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
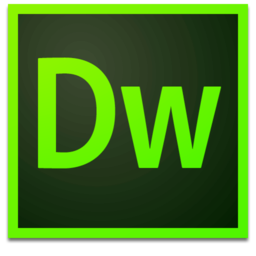
Dreamweaver Mac version
Visual web development tools
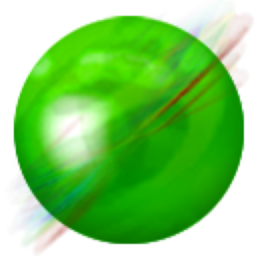
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software