How to use MessageFormat function in Java for text formatting
With the continuous development of the Java language, the various APIs and functions provided by Java are becoming increasingly rich. Among them, the MessageFormat function is a very practical text formatting tool. The MessageFormat function can format strings in Java programs, automatically replace placeholders in messages in the generated output, and retain locale information to make the output content more accurate. This article will describe in detail how to use the MessageFormat function to format text.
1. What is the MessageFormat function
In Java, MessageFormat is a class that provides a method for formatting and outputting complex messages. MessageFormat allows you to define a message that contains parameters and then simply pass values to that message to generate a new output message. The format of the MessageFormat function is:
MessageFormat.format(String pattern, Object ... arguments)
The pattern parameter specifies the format of the message, and the arguments parameter replaces the placeholders in the message with Parameter array of actual values. For example, in the following code, we will use the MessageFormat function to format a string of name, age and occupation into a greeting:
String name = "Xiao Ming";
int age = 22;
String job = "Programmer";
String greeting = MessageFormat.format("Hello, {0}, you are {1} years old this year and you are a {2}.", name, age , job);
System.out.println(greeting);
The output result is: Hello, Xiao Ming, you are 22 years old and a programmer.
2. The format specifier of the MessageFormat function
In the MessageFormat function, the placeholder needs to be corresponding to the actual value, so the format specifier needs to be used. A format specifier is a markup syntax used to determine the format of a replacement string. The general structure of the format specifier is as follows:
{argument_index, format_type, format_style}
Note that the format specifier contains three parts, namely parameter index, format type and format style. The parameter index specifies the subscript of the parameter to be replaced in the parameter array, the format type specifies the data type of the parameter to be replaced, and the format style specifies the string format used to format the parameter.
1. Parameter index
The parameter index refers to the number in the placeholder, which specifies the subscript of the parameter to be replaced in the parameter array. For example, in the above code, the placeholder "{0}" specifies the first parameter, which is the string variable name.
2. Format type
The format type refers to the data type of the parameter to be replaced. The data types supported by the MessageFormat function include:
- Number: number
- Date and time: date, time
- Percent: percentage
- Currency: currency
- Custom type: choice
The following is an example , which illustrates how to use the format type to format a number and replace it with a placeholder:
int count = 123456;
String message = MessageFormat.format("We have {0, number} users.", count);
The output result is: We have 123,456 users.
3. Format style
Format style refers to the string format used to format parameters. The syntax of format styles depends on the format type. In the following example, we will use the format style to format the date and time into the specified format:
Date now = new Date();
String message = MessageFormat.format("The current time is { 0,time,HH:mm:ss}. The date is {0,date,yyyy-MM-dd}.", now);
The output result is: the current time is 14:58:30. The date is 2021-02-16.
3. Practical tips for using the MessageFormat function
1. Localization support
In Java, localization is a process of providing correctly formatted strings for different languages, cultures, and regions. The MessageFormat function can take localization information into account. For example, in the code below, we will use the MessageFormat function to format a date and generate a localized date based on the given region information:
int year = 2021;
int month = 2;
int day = 16;
String result = MessageFormat.format("The date is {0,date,MMM d, yyyy}.",
new GregorianCalendar(year, month - 1, day));
System.out.println(result);
The output result will be:
The date is February 16, 2021.
2. Select the format
If you need to select a different format based on the value of a certain parameter , you can use the format type choice. choice can map the value of a parameter to another value according to a range for use by the MessageFormat function. For example, in the following code, we will use the choice format type to map a score For the grade:
double score = 0.9;
String result = MessageFormat.format("This student’s score is {0,number,percent}. The grade is: {0,choice,0#failed |0
score);
System.out. println(result);
The output result will be:
This student’s score is 90.00%. The grade is: excellent.
3. Escape placeholders and modifiers
When using the MessageFormat function, characters with special meanings (such as '{', '}' and '#') need to use double curly brackets "{{" and "}}" to escape. If you need to modify the format specifier, you can use modifiers. Modifiers can add or remove formatting styles and add arbitrary text to them. For example, in the code below, we will use modifiers to add an exclamation point after the output:
String name = "Xiao Ming";
String result = MessageFormat.format("Welcome, {0}! ", new Object[] { name });
System.out.println(result "{0,choice,-1#?Please check the data|0#|1#!}");
The output result is:
Welcome, Xiao Ming!
In this article, we explain how to use the MessageFormat function for text formatting, including format specifiers, localization support, selecting formats, and escaping placeholders and modifiers. In addition, the MessageFormat function can also be used to format USB messages, format HTML pages, format emails, etc. For more information on how to use the MessageFormat function, you can learn more by viewing the official Java documentation and API documentation. The MessageFormat function is a very practical tool in Java programming, helping us format text and messages more efficiently.
The above is the detailed content of How to use MessageFormat function in Java for text formatting. For more information, please follow other related articles on the PHP Chinese website!
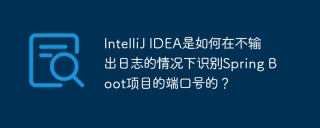
Start Spring using IntelliJIDEAUltimate version...
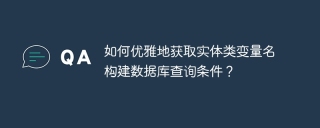
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
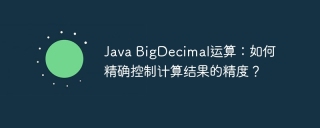
Java...
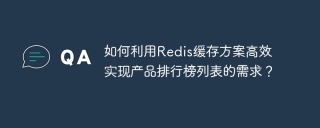
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
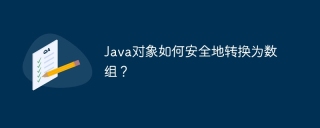
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
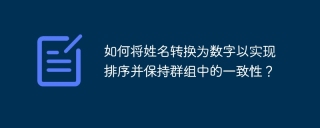
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
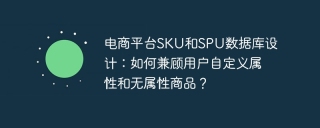
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
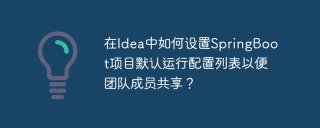
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
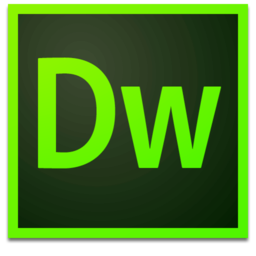
Dreamweaver Mac version
Visual web development tools
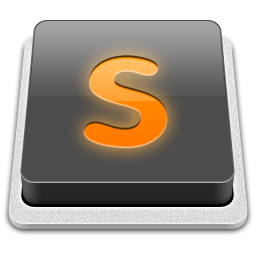
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
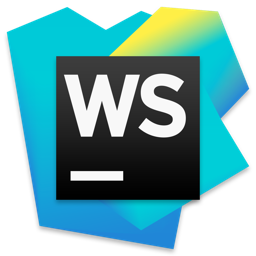
WebStorm Mac version
Useful JavaScript development tools