How to use Regex function in Java for regular expression matching
Regular expression is an expression for string operations, which can be used in various scenarios such as searching, replacing, and validating strings. In Java, you can use the Regex function for regular expression matching operations. This article will introduce how to use the Regex function in Java for regular expression matching.
1. Introduction to Regex function
The Regex function in Java belongs to the java.util.regex package and provides a variety of methods to perform regular expression matching operations. The core classes are Pattern and Matcher.
The Pattern class is used to define the pattern of regular expressions. Its constructor is Pattern.compile(String regex), where regex is the string representation of the regular expression.
The Matcher class is used to match strings. Its constructor is pattern.matcher(String input), where pattern is the Pattern object and input is the string that needs to be matched.
2. The syntax of regular expressions
Before matching regular expressions, you need to understand the syntax of regular expressions. The following are some basic elements of regular expression syntax:
- Ordinary characters: a, b, c, d and other characters represent themselves.
- Special characters: Backslash \ followed by specific characters can represent some special characters, such as . represents ., ? represents ?, etc.
- Character group: Use square brackets [] to match any character within the brackets, such as [a-z] to match any character between a to z.
- Exclusive character group: Use square brackets [^] to match any character except the characters in the brackets, such as 1 to match any non- Numeric characters.
- Quantifier: used to limit the number of occurrences of the preceding character. Commonly used quantifiers include , ,?, {m}, {m,}, {m,n}, where means Match 0 or more of the preceding characters, indicating matching of 1 or more, ? indicating matching of 0 or 1, {m} indicating that m must be matched, {m,} indicating matching of m or more, {m ,n} means matching m to n.
- Selectors and grouping: Use parentheses () to indicate grouping, and vertical bars | to indicate selectors, such as (ab|cd) to match ab or cd.
3. Use of Regex function
The general process of using the Regex function is: define the pattern of the regular expression (i.e. Pattern object), and perform matching operations on the strings that need to be matched. (i.e. Matcher object), query the matching results.
The following is a basic usage example of the Regex function:
import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexExample { public static void main(String[] args) { String regex = "cat"; String input1 = "The cat is on the mat."; String input2 = "A cat and a dog."; Pattern pattern = Pattern.compile(regex); Matcher matcher1 = pattern.matcher(input1); Matcher matcher2 = pattern.matcher(input2); System.out.println("input1中是否包含cat:" + matcher1.find()); System.out.println("input2中是否包含cat:" + matcher2.find()); } }
The output result is:
input1中是否包含cat:true input2中是否包含cat:true
In the above code, a regular expression pattern is first defined, that is, the string " cat". Then perform matching operations on two different input strings.
In the specific matching operation, first create a Pattern object through the Pattern.compile(String regex) method, and then create a Matcher object through the matcher(String input) method of the object, and then you can use the Matcher object The find() method queries whether the input string contains a sequence of characters in the pattern.
In addition to using the find() method, the Matcher object also provides many other methods for obtaining different information about the matching results, such as the starting position of the match, the matched substring, etc.
4. Commonly used regular expression examples
- Check whether it is a number:
public static boolean isDigit(String str) { Pattern pattern = Pattern.compile("[0-9]*"); return pattern.matcher(str).matches(); }
- Check whether it is a legal email address:
public static boolean isEmail(String str) { Pattern pattern = Pattern.compile("^\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*$"); return pattern.matcher(str).matches(); }
- Check whether it is a legal mobile phone number:
public static boolean isMobileNumber(String str) { Pattern pattern = Pattern.compile("^1\d{10}$"); return pattern.matcher(str).matches(); }
- Check whether it is a legal ID number:
public static boolean isIDCardNumber(String str) { Pattern pattern = Pattern.compile("^\d{17}[0-9xX]$"); return pattern.matcher(str).matches(); }
5. Notes
- To ensure the correctness of the regular expression, it is recommended to verify it on the regular expression online testing tool first.
- When performing multiple matching operations, you should use the find() method of the Matcher object in a while loop to obtain all matching positions.
- When performing regular expression matching operations, be careful not to rely too much on regular expressions. You should choose the simplest method to achieve the required functions.
The above is the introduction and examples of using the Regex function in Java for regular expression matching. I hope it will be helpful to you!
- 0-9 ↩
The above is the detailed content of How to use Regex function in Java for regular expression matching. For more information, please follow other related articles on the PHP Chinese website!
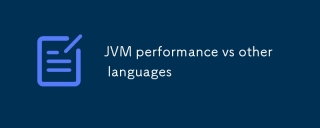
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
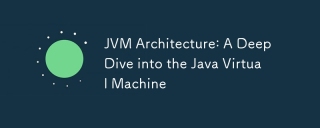
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
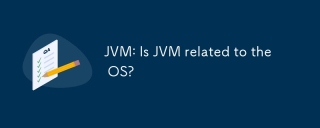
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
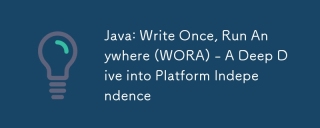
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
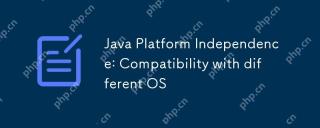
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
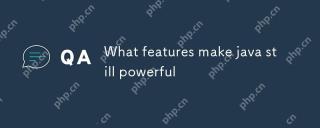
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
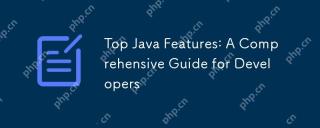
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
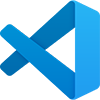
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
