


How to use Google BigQuery for big data calculation and storage in PHP development
As the amount of data continues to increase, traditional database management systems can no longer meet the storage and computing needs of big data. Google BigQuery, as a new type of cloud storage and computing service, is used by more and more enterprises and developers. This article will introduce how to use Google BigQuery for big data calculation and storage in PHP development.
1. What is Google BigQuery
Google BigQuery is a powerful cloud big data analysis service that can efficiently query massive data through SQL statements and store it through Google Cloud Storage. Google BigQuery enables rapid analysis of data without the expense and stress of any server or database management. Google BigQuery supports SQL language and can handle petabyte-level data.
2. What preparations are needed to use Google BigQuery
- Google Cloud Platform account: Before using Google BigQuery, you need to apply for a Google Cloud Platform account and activate the Google BigQuery service.
- Google Cloud SDK installation: Using Google BigQuery in local development requires installing the Google Cloud SDK and making the necessary configurations. Installation and configuration can be done via the official website or the command line.
- API Credentials: Before developing with the Google BigQuery API, you need to obtain Google API credentials. You need to enable the Google BigQuery API on the Google Cloud Console first and create an API credential. Types of credentials include OAuth2.0 client ID, service account key, and API key. Among them, the OAuth2.0 client ID is suitable for use in web applications, the service account key is suitable for use in applications executed on the backend, and the API key is suitable for use in simple HTTP/REST applications.
3. Use Google BigQuery for data query
In PHP development, we can use the Google API client library to use the Google BigQuery API. First, you need to create a project in the Google Cloud Console and enable the BigQuery API service in the project. Then, create a Service Account in Google Cloud Console and obtain the credentials.json credentials file. Finally, download and install the Google API PHP client library.
The following is the code implementation of using Google BigQuery for data query:
<?php require_once __DIR__ . '/vendor/autoload.php'; putenv('GOOGLE_APPLICATION_CREDENTIALS=' . __DIR__ . '/credentials.json'); $client = new Google_Client(); $client->useApplicationDefaultCredentials(); $client->addScope(Google_Service_Bigquery::BIGQUERY); // 设置查询选项 $options = [ 'useLegacySql' => false ]; // 查询SQL语句 $sql = 'SELECT count(*) as count FROM `project.dataset.table`'; // 创建BigQuery服务对象 $service = new Google_Service_Bigquery($client); // 从BigQuery查询数据 $results = $service->jobs->query('project-id', new Google_Service_Bigquery_QueryJobConfiguration([ 'query' => $sql, 'useLegacySql' => false ])); // 从结果中获取行数据 if ($rows = $results->getRows()) { $count = $rows[0]['f'][0]['v']; echo 'count: ' . $count . PHP_EOL; }
In the above code, we use the Google API PHP client library to create a BigQuery service object. Then, we set the query options, query the data through SQL statements, and obtain row data from the query results. Finally, we can perform data processing on the query results as needed.
4. Use Google BigQuery for data storage
In Google BigQuery, we can store data into the data set in various ways, including batch insertion, streaming data insertion, table import, etc. Below we will take batch insertion as an example to introduce how to use Google BigQuery for data storage.
- Create datasets and tables
Before using Google BigQuery for data storage, you need to create a dataset and table in the Google Cloud Console. By creating datasets and tables, we can specify the data type and structure for the data we want to store.
- Install the PHP extension of Google Cloud BigQuery
Using Google BigQuery for data storage requires the installation of the PHP extension of Google Cloud BigQuery, which can be installed on the official website or the command line. After the installation is complete, you need to add the Google Cloud BigQuery extension to the PHP configuration file.
- Writing a PHP program
The following is an example of a PHP program using Google BigQuery for data storage:
<?php require_once __DIR__ . '/vendor/autoload.php'; putenv('GOOGLE_APPLICATION_CREDENTIALS=' . __DIR__ . '/credentials.json'); $client = new Google_Client(); $client->useApplicationDefaultCredentials(); $client->addScope(Google_Service_Bigquery::BIGQUERY); // 数据集和表的名称 $datasetName = 'project.dataset'; $tableName = 'table'; // 插入数据 $service = new Google_Service_Bigquery($client); $rows = [ ['column1' => 'value1', 'column2' => 123], ['column1' => 'value2', 'column3' => 'value3'] ]; $service->tabledata->insertAll($projectId, $datasetName, $tableName, new Google_Service_Bigquery_TableDataInsertAllRequest([ 'rows' => $rows ]));
In the above example, we use Google API The PHP client library creates a BigQuery service object, specifies the name of the data set and table to be stored, and inserts data into the data table through the tabledata->insertAll()
method. Among them, $rows
is the row data to be inserted, and each row data is an associative array (the key is the column name and the value is the column value).
Summary
As a new type of cloud storage and computing service, Google BigQuery provides developers with powerful big data analysis capabilities. In PHP development, we can use the Google BigQuery API through the Google API PHP client library to achieve efficient query and storage of data. I hope this article will help you use Google BigQuery in PHP development.
The above is the detailed content of How to use Google BigQuery for big data calculation and storage in PHP development. For more information, please follow other related articles on the PHP Chinese website!
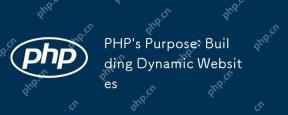
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
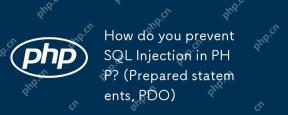
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
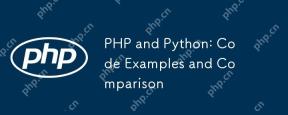
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
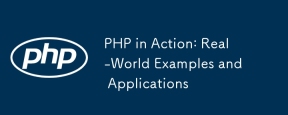
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
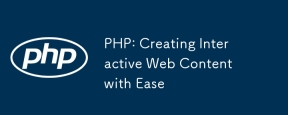
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
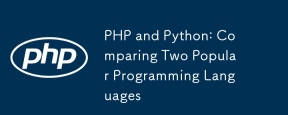
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
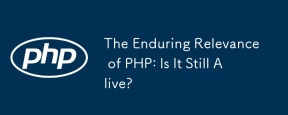
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
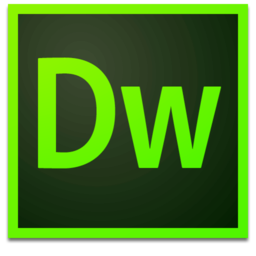
Dreamweaver Mac version
Visual web development tools