How to implement a Youdao dictionary-like page design in Vue?
Vue is a very popular JavaScript framework for building modern, high-performance web applications. In Vue, using component-based development to build pages makes it very convenient to implement various complex UI designs. This article will introduce how to use Vue to implement a page design similar to Youdao Dictionary.
1. Page design
Before starting the implementation of Vue, let’s take a look at the page design of imitating Youdao Dictionary. The design is divided into three main parts: the top navigation bar, the search box, and the search results list.
Top navigation bar: It consists of a logo and two navigation links. The logo can be clicked to return to the homepage. Navigation links generally include "Home" and "Translation".
Search box: includes an input box and a search button. Users can enter the word or phrase they want to query in the input box and click the search button to query.
Search result list: Query results are presented in list form, and each query result includes the definition and example sentences of the word or phrase.
2. Division of components
According to the page design, the entire page can be divided into three components: navigation bar component, search box component and search result component.
Navigation bar component: Mainly responsible for the style and logic of the top navigation bar.
Search box component: Mainly responsible for the style and logic of the search box.
Search result component: Mainly responsible for displaying the style and logic of query results.
3. Component implementation
- Navigation bar component
First, you need to introduce the navigation bar component into Vue's App.vue.
<template> <div id="app"> <nav-bar></nav-bar> <router-view></router-view> </div> </template> <script> import NavBar from './components/NavBar.vue' export default { name: 'App', components: { NavBar } } </script> <style> /* 全局样式 */ </style>
Next, create the NavBar.vue component and define the style and logic of the navigation bar.
<template> <div id="nav-bar"> <div class="nav-left"> <img src="/static/imghwm/default1.png" data-src="./assets/logo.png" class="lazy" alt="logo" @click="backToHome"> <div class="nav-link" @click="$router.push('/')">首页</div> <div class="nav-link" @click="$router.push('/translate')">翻译</div> </div> <div class="nav-right"> <!-- 登录按钮等右侧组件 --> </div> </div> </template> <script> export default { name: 'NavBar', methods: { backToHome() { this.$router.push('/') } } } </script> <style> /* 导航栏样式 */ </style>
- Search box component
The search box component contains an input box and a search button. The user enters the word or phrase to be queried in the input box and clicks the search button. Make an inquiry. Similar to the navigation bar component, first introduce the search box component in App.vue.
<template> <div id="app"> <nav-bar></nav-bar> <search-box></search-box> <router-view></router-view> </div> </template> <script> import NavBar from './components/NavBar.vue' import SearchBox from './components/SearchBox.vue' export default { name: 'App', components: { NavBar, SearchBox } } </script> <style> /* 全局样式 */ </style>
Next, create the SearchBox.vue component and define the style and logic of the search box.
<template> <div id="search-box"> <input type="text" class="search-input" v-model="searchWord"> <button class="search-btn" @click="search">搜索</button> </div> </template> <script> export default { name: 'SearchBox', data() { return { searchWord: '' } }, methods: { search() { // 跳转到搜索结果页面,将searchWord作为查询参数 this.$router.push({path: '/search', query: {word: this.searchWord}}) } } } </script> <style> /* 搜索框样式 */ </style>
- Search result component
The search result component is mainly responsible for displaying the style and logic of query results. First introduce the search result component in App.vue.
<template> <div id="app"> <nav-bar></nav-bar> <search-box></search-box> <search-result v-if="$route.path === '/search'"></search-result> <router-view></router-view> </div> </template> <script> import NavBar from './components/NavBar.vue' import SearchBox from './components/SearchBox.vue' import SearchResult from './components/SearchResult.vue' export default { name: 'App', components: { NavBar, SearchBox, SearchResult } } </script> <style> /* 全局样式 */ </style>
Next, create the SearchResult.vue component and define the style and logic of the search results. Get the query parameter word in the created cycle hook, and get the query results by calling the external API.
<template> <div id="search-result"> <div v-if="resultLoading" class="result-loading">正在查询...</div> <ul v-else class="result-list"> <li v-for="(item, index) in resultList" :key="index"> <h3 id="item-word">{{item.word}}</h3> <p class="result-translation">{{item.translation}}</p> <p class="result-example">{{item.example}}</p> </li> </ul> </div> </template> <script> export default { name: 'SearchResult', data() { return { resultLoading: true, resultList: [] } }, created() { const query = this.$route.query if (query.word) { // 调用API查询结果 this.resultLoading = false this.resultList = [...] } } } </script> <style> /* 搜索结果样式 */ </style>
Here we only briefly introduce the implementation method of the component. In actual development, it needs to be adjusted and improved according to specific needs.
4. Summary
Vue’s component-based development method is very flexible and can easily implement various complex UI designs. This article introduces how to use Vue to implement a high-quality search application by imitating the page design of Youdao Dictionary. In addition to the above three components, functions such as history records and vocabulary books can also be added to enhance the user experience. I hope this article will be helpful to readers who are new to Vue.
The above is the detailed content of How to implement a Youdao dictionary-like page design in Vue?. For more information, please follow other related articles on the PHP Chinese website!

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
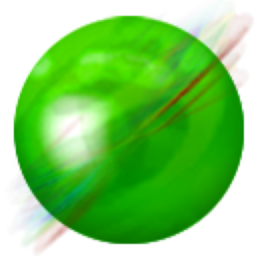
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor