


Using swoole extension in PHP to achieve high concurrency processing
With the continuous development of the Internet, there are more and more websites and web applications of various types. At this time, dealing with high concurrency has become an inevitable problem. High concurrency is not just a problem of large visits, but also the problem of multiple requests being initiated at the same time. For web applications, the ability to handle multiple requests is also the ability to process concurrently.
In PHP, the swoole extension provides a very excellent model to handle high concurrent requests. The Swoole extension is open source and is similar to an extension of the PHP language. It is very suitable for high-performance TCP/UDP server development. This extension is based on event-driven and asynchronous I/O technology and can greatly improve PHP's processing of network requests. performance.
Now, let’s take a look at how to use swoole to handle high concurrent requests.
Install swoole extension
First, we need to install the swoole extension. Before installing, you need to make sure you have PHP installed. The swoole extension is already registered with PECL and is very easy to install. Just run the following command in the terminal:
pecl install swoole
If PECL is not installed, you need to install PECL first:
yum install php-pear
or
apt-get install php-pear
Configure swoole
After the installation is complete, you can enable the swoole extension in the php.ini file. Just add the following configuration to the php.ini file:
extension=swoole.so
Now, we have installed and configured the swoole extension. Next, we will introduce how to use swoole to handle high concurrent requests.
Implement HTTP server
To implement HTTP server, we need to use Swoole's swoole_http_server class. The HTTP server can be implemented using the following code:
<?php // 创建Server对象,监听 127.0.0.1:9501端口 $serv = new SwooleHttpServer("127.0.0.1", 9501); //监听请求事件 $serv->on('Request', function ($request, $response) { $response->header("Content-Type", "text/html; charset=utf-8"); $response->end("Hello World"); }); //启动服务器 $serv->start(); ?>
In the above code, we have created a Swoole HTTP server and bound it on the 127.0.0.1:9501 port. We then handle the request in the request event using the on method and send the response to the client.
At the same time, swoole supports asynchronous request processing, which maximizes the processing capabilities of concurrent requests.
In addition to the swoole_http_server class, Swoole also provides many other classes to handle different request types. These classes include swoole_websocket_server, swoole_redis_server, swoole_mysql_server, etc.
Using coroutine technology
Coroutine technology is a very important part when using swoole to handle high concurrent requests. A coroutine is a lightweight thread that can run within a single thread and can be suspended and resumed when needed. Using coroutines can maximize the number of requests processed at the same time.
In order to use coroutines, we need to set asynchronous mode when starting the server. When the server is in async mode, coroutines can easily be used to handle requests. The following is a piece of code that enables asynchronous mode:
<?php // 创建Server对象,监听 127.0.0.1:9501端口 $serv = new SwooleHttpServer("127.0.0.1", 9501); //设置异步模式 $serv->set(array( 'worker_num' => 4, 'enable_coroutine' => true, )); //监听请求事件 $serv->on('Request', function ($request, $response) { //处理请求 go(function () use ($response) { $result = file_get_contents('https://www.example.com'); $response->end($result); }); }); //启动服务器 $serv->start(); ?>
In the above code, we set worker_num to 4, and Swoole will process 4 requests concurrently. At the same time, by enabling coroutine technology, requests are processed more efficiently and performance is improved.
Using swoole_client
In some cases, we need to connect to other servers to handle requests, which requires the use of the swoole_client class. The swoole_client class provides network client functions and can communicate with other TCP/UDP servers.
The following is a sample code that uses the swoole_client class to handle requests:
<?php $client = new SwooleClient(SWOOLE_SOCK_TCP); //连接到服务器 if (!$client->connect('127.0.0.1', 9501, 0.5)) { die("连接失败!"); } //发送请求 $client->send('Hello World'); //接收响应 $response = $client->recv(); //关闭连接 $client->close(); echo $response; ?>
In the above code, we create a swoole_client object and connect to the server. We then send the request to the server and wait for the server to send the response. Finally, we close the connection and print the response.
Summary
Using swoole extension can handle high concurrent requests well. By using the asynchronous processing technology and coroutine technology provided by swoole, we can greatly improve the performance of PHP in processing network requests. At the same time, swoole also provides many other classes to handle different request types, such as TCP/UDP server, WebSocket server, Redis server, MySQL server, etc.
In actual development, it is very important to use swoole to handle high concurrent requests. Not only does it improve program runtime performance, it also improves efficiency and reliability when handling large numbers of requests. I hope this article can help you better understand how to use swoole to handle high concurrent requests.
The above is the detailed content of Using swoole extension in PHP to achieve high concurrency processing. For more information, please follow other related articles on the PHP Chinese website!
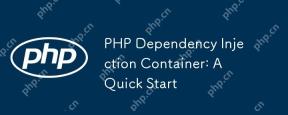
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
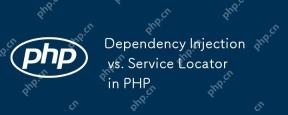
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
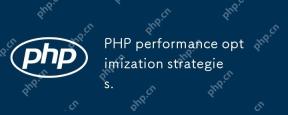
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
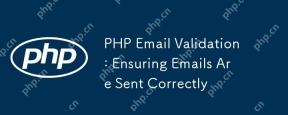
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
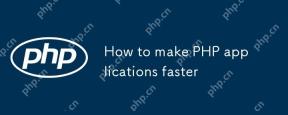
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
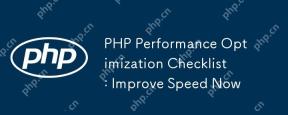
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
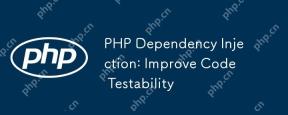
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
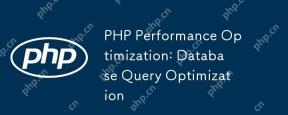
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
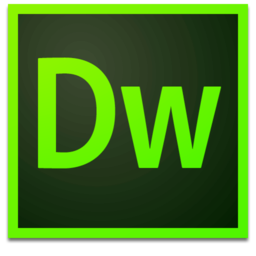
Dreamweaver Mac version
Visual web development tools
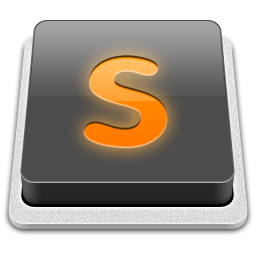
SublimeText3 Mac version
God-level code editing software (SublimeText3)
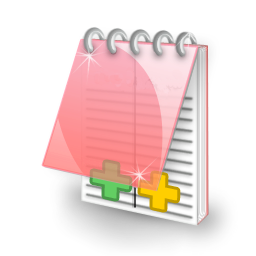
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
