In what scenarios does IllegalAccessException occur in Java?
Java is a widely used programming language, and many developers encounter various exceptions when using Java. One of them is the IllegalAccessException exception, which usually occurs in the access control context of Java classes.
The IllegalAccessException exception in Java is an unauthorized access exception. In a Java program, this exception is thrown if you try to access a restricted method, constructor, or field. Usually, this exception occurs in the following situations:
- Access Control
When a method, constructor, or field is private, protected, or package-private , they can only be accessed within the same class or package, if they are accessed elsewhere, an IllegalAccessException will be thrown.
Look at the following example:
public class MyClass { private int myPrivateField; protected int myProtectedField; int myPackagePrivateField; private void myPrivateMethod() { System.out.println("This is a private method."); } protected void myProtectedMethod() { System.out.println("This is a protected method."); } void myPackagePrivateMethod() { System.out.println("This is a package-private method."); } } public class AnotherClass { public static void main(String[] args) { MyClass myObject = new MyClass(); // myObject.myPrivateField = 10; // IllegalAccessException异常 // myObject.myProtectedMethod(); // IllegalAccessException异常 // myObject.myPackagePrivateMethod(); // 可以被访问 } }
In the above code, the myPrivateField field and myProtectedMethod method in the MyClass class are private or protected and therefore cannot be accessed in the AnotherClass class. Only myPackagePrivateMethod can be accessed.
- Code Reflection
Code reflection is a common advanced feature in Java that can dynamically access and manipulate Java objects at runtime. When using the reflection API to access private members, an IllegalAccessException may also be thrown.
Look at the following example:
public class MyClass { private int myPrivateField = 5; } public class AnotherClass { public static void main(String[] args) { MyClass myClassObject = new MyClass(); try { Field privateField = MyClass.class.getDeclaredField("myPrivateField"); privateField.setAccessible(true); System.out.println(privateField.get(myClassObject)); // 5 } catch (NoSuchFieldException | IllegalAccessException e) { e.printStackTrace(); } } }
In the above code, reflection is used to obtain the myPrivateField field in the MyClass class, and its access level is set to accessible through the setAccessible method. However, in this case, if myPrivateField is final, an IllegalAccessException will be thrown because the value of the final field cannot be modified.
- Class loader
The class loader is responsible for loading compiled Java classes into the Java virtual machine. When using the ClassLoader.loadClass() method to load a class, if the class defines a private or protected constructor, an IllegalAccessException exception will be thrown when using the newInstance() method to create a new instance of the class.
Look at the following example:
public class MyClass { private MyClass() { System.out.println("MyClass instantiated."); } } public class AnotherClass { public static void main(String[] args) { try { Class<?> myClass = Class.forName("MyClass"); Constructor<?> cons = myClass.getDeclaredConstructor(); cons.setAccessible(true); MyClass myClassObject = (MyClass)cons.newInstance(); } catch (InstantiationException | IllegalAccessException | IllegalArgumentException | InvocationTargetException | NoSuchMethodException | SecurityException | ClassNotFoundException e) { e.printStackTrace(); } } }
In the above code, the reflection API is used to obtain the constructor of the class MyClass, and the access modifier of the constructor is set to accessible. However, if the constructor in the MyClass class is private, the newInstance() method will throw an IllegalAccessException.
When developing Java applications, always pay attention to code access control to avoid IllegalAccessException exceptions. If you encounter this exception, you can solve the problem by modifying code access permissions or using an appropriate reflection API.
The above is the detailed content of In what scenarios does IllegalAccessException occur in Java?. For more information, please follow other related articles on the PHP Chinese website!
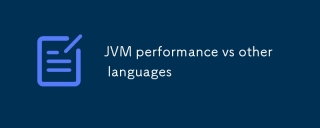
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
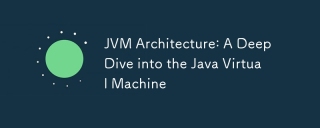
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
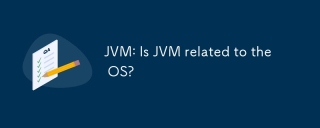
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
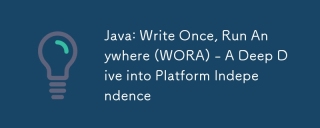
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
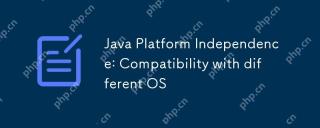
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
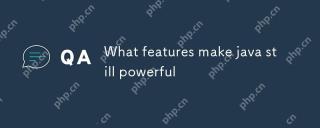
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
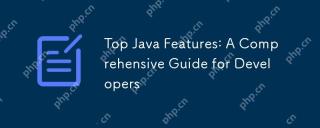
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
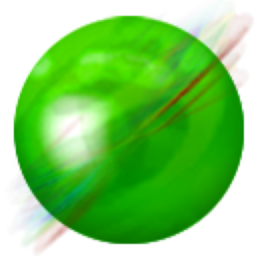
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
