With the development of web applications, labeled input boxes are becoming more and more popular. This kind of input box allows users to input data more conveniently, and also facilitates users to manage and search the entered data. Vue is a very powerful JavaScript framework that can help us quickly implement labeled input boxes. This article will introduce how to use Vue to implement a labeled input box.
Step one: Create a Vue instance
First, we need to create a Vue instance on the page, the code is as follows:
<template> <div> <div> <label>标签:</label> <input type="text" v-model="newTag" v-on:keyup.enter="addTag"> </div> <div> <label>标签列表:</label> <div v-for="(tag, index) in tags" :key="index"> {{ tag }} <button v-on:click="removeTag(index)">删除</button> </div> </div> </div> </template> <script> export default { data() { return { tags: ["标签1", "标签2", "标签3"], newTag: "" }; }, methods: { addTag() { if (this.newTag.trim() !== "") { this.tags.push(this.newTag.trim()); this.newTag = ""; } }, removeTag(index) { this.tags.splice(index, 1); } } }; </script>
In the code, we create a Vue instance containing a Vue component with input box and a list of tags. The input box is used to add new tags, and the tag list is used to display existing tags and provides the function of deleting tags.
In the data
method of the component, we define two data items: tags
is used to store the data of all tags. The initial value is one containing three tags. The array; newTag
is used to store the data of the new tag entered by the user, and the initial value is an empty string.
In methods
, we define two methods: addTag
is used to add a new tag, which will add the new tag after the user presses the Enter key. to the tags
array, and set newTag
to an empty string; removeTag
is used to delete tags, it will be removed from based on the incoming tag index tags
Delete the corresponding tag from the array.
Step 2: Display the tag list
Next, we need to display the tag list. To achieve this functionality, we use the v-for
directive in Vue. The v-for
directive can loop through the data in an array and display it on the page.
In the code, we use v-for="(tag, index) in tags"
to loop through all the tags in the tags
array, tag
represents each tag in the array, and index
represents the index of the tag in the array. We use the :key="index"
attribute to uniquely identify the index of the tag, which helps Vue optimize the performance of the component.
Step 3: Add a new tag
When the user enters a new tag in the input box and presses the Enter key, we need to add the new tag to tags
in the array. To achieve this functionality, we use the v-model
directive in Vue. v-model
The directive can bidirectionally bind the data in the component to the form elements on the page.
In the code, we use v-model="newTag"
to bidirectionally bind the value in the input box to the newTag
data in the component. In this way, when the user enters a new tag in the input box, the value of newTag
will also change accordingly.
We also used the v-on
directive in Vue to listen for user key events. When the user presses the Enter key, v-on:keyup.enter
will trigger the addTag
method to add the new tag to the tags
array.
Step 4: Delete Tags
Finally, we need to allow users to delete tags. To achieve this functionality, we use a button, and when the user clicks the button, we remove the corresponding tag from the tags
array.
In the code, we use the v-on
directive in Vue to listen to the user's click event. When the user clicks the delete button, v-on:click
will trigger the removeTag
method, taking the incoming tag index as a parameter and removing the corresponding tag from the tags
array. Tag of.
Summary
Through the above demonstration, we learned how to use Vue to implement a labeled input box. We used the v-for
, v-model
and v-on
directives in Vue to display the label list and the value of the two-way binding input box. As well as listening to keyboard and mouse events for input boxes and buttons. In actual projects, we can customize the label style and operation method according to needs to achieve a more flexible labeled input box.
The above is the detailed content of How to implement a labeled input box using Vue?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is loved by developers because it is easy to use and powerful. 1) Its responsive data binding system automatically updates the view. 2) The component system improves the reusability and maintainability of the code. 3) Computing properties and listeners enhance the readability and performance of the code. 4) Using VueDevtools and checking for console errors are common debugging techniques. 5) Performance optimization includes the use of key attributes, computed attributes and keep-alive components. 6) Best practices include clear component naming, the use of single-file components and the rational use of life cycle hooks.

Vue.js is a progressive JavaScript framework suitable for building efficient and maintainable front-end applications. Its key features include: 1. Responsive data binding, 2. Component development, 3. Virtual DOM. Through these features, Vue.js simplifies the development process, improves application performance and maintainability, making it very popular in modern web development.

Vue.js and React each have their own advantages and disadvantages, and the choice depends on project requirements and team conditions. 1) Vue.js is suitable for small projects and beginners because of its simplicity and easy to use; 2) React is suitable for large projects and complex UIs because of its rich ecosystem and component design.

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.

Vue.js' role in web development is to act as a progressive JavaScript framework that simplifies the development process and improves efficiency. 1) It enables developers to focus on business logic through responsive data binding and component development. 2) The working principle of Vue.js relies on responsive systems and virtual DOM to optimize performance. 3) In actual projects, it is common practice to use Vuex to manage global state and optimize data responsiveness.

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
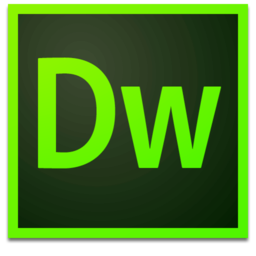
Dreamweaver Mac version
Visual web development tools
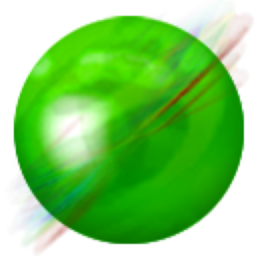
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software