


What should I do if 'Error: Access Denied' appears when using vue-resource in a Vue application?
Vue is a popular JavaScript framework that helps developers build modern web applications. In Vue, vue-resource is a commonly used HTTP library that provides a convenient way to send HTTP requests and handle responses.
However, when using vue-resource, you may encounter a common error: Error: Access Denied. This error usually occurs when trying to send an HTTP request and it means that you do not have sufficient permissions to access the resource. Below we will introduce some solutions.
1. Check the server settings
"Error: Access Denied" is usually caused by CORS (Cross-Origin Resource Sharing) issues. To resolve this issue, you need to make sure your server is set up correctly. On the server side, you need to allow cross-origin requests. This can be achieved by setting the correct response headers. In the HTTP response, you need to set header information such as Access-Control-Allow-Origin and Access-Control-Allow-Methods to allow requests to be sent via CORS. If you are using Node.js as your server, you can easily achieve this using CORS middleware.
2. Use a proxy
Another way to solve the CORS problem is to use a proxy in the Vue application. In development mode, you can use the configuration file vue.config.js to set the proxy. For example, you can configure your Vue application to run on localhost:8080 and forward all API requests to http://example.com/api:
module.exports = {
devServer: {
proxy: { '^/api': { target: 'http://example.com', changeOrigin: true, pathRewrite: { '^/api': '/api' } } }
}
}
This will tell the Vue application to redirect API requests to http://example.com/api when sending them, thus avoiding CORS question.
3. Use JSONP
JSONP is another way to solve CORS problems. It allows you to request resources from different domains directly in your browser. In a Vue application, you can use the vue-jsonp plugin to implement JSONP. First, you need to install vue-jsonp:
npm install vue-jsonp --save
Then use it in your Vue application:
import Vue from 'vue'
import VueJsonp from 'vue-jsonp'
Vue.use(VueJsonp)
Now you can use Vue's VueJsonp method to send requests to different domain names.
Summary
"Error: Access Denied" when using vue-resource in a Vue application usually means that you do not have sufficient permissions to access the resource. This problem is usually caused by CORS issues. To solve this problem, you can check the server settings, use a proxy or use JSONP. Using these techniques, you can easily solve the "Error: Access Denied" problem and make your Vue application work properly.
The above is the detailed content of What should I do if 'Error: Access Denied' appears when using vue-resource in a Vue application?. For more information, please follow other related articles on the PHP Chinese website!

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
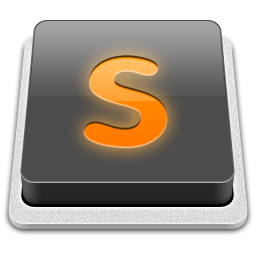
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
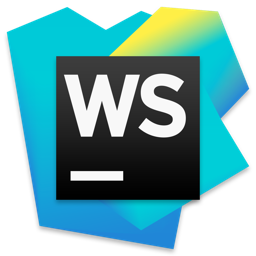
WebStorm Mac version
Useful JavaScript development tools
