With the rapid development of Internet technology, the importance of Web applications is becoming more and more important. In order to ensure the quality and reliability of web applications, testing is inevitable. In Golang learning, web application testing is also a part that needs to be focused on and studied. This article will describe the testing of web applications in Golang learning, including unit testing, integration testing and end-to-end testing.
- Unit testing
Unit testing refers to testing the smallest testable unit in the program to ensure that each unit of the program can run normally. In web applications, unit testing generally targets logical units such as routing and processors.
The following is a simple example showing how to use the testing package of the Go language for unit testing:
package main import ( "net/http" "net/http/httptest" "testing" ) func TestHelloHandler(t *testing.T) { req, err := http.NewRequest("GET", "/hello", nil) if err != nil { t.Fatal(err) } rr := httptest.NewRecorder() handler := http.HandlerFunc(helloHandler) handler.ServeHTTP(rr, req) if status := rr.Code; status != http.StatusOK { t.Errorf("handler returned wrong status code: got %v want %v", status, http.StatusOK) } if rr.Body.String() != "Hello, World!" { t.Errorf("handler returned unexpected body: got %v want %v", rr.Body.String(), "Hello, World!") } } func helloHandler(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Hello, World!")) }
Above we created the TestHelloHandler test function using the testing.T structure type of the testing package. This function will initiate a GET request to the "/hello" route of the application and check whether the response status code and response body are correct.
- Integration testing
Integration testing refers to integrating the dependencies between unit tests to ensure that the entire program can run normally. In web applications, integration testing generally tests SQL statements, stored procedures, etc.
The following is a simple example showing how to use the Go language's net/http and database/sql packages for integration testing:
package main import ( "database/sql" "log" "net/http" "net/http/httptest" "os" "testing" _ "github.com/lib/pq" ) var ( db *sql.DB ts *httptest.Server ) func TestMain(m *testing.M) { db, _ = sql.Open("postgres", "user=postgres password=postgres host=localhost port=5432 dbname=test sslmode=disable") defer db.Close() if err := db.Ping(); err != nil { log.Fatalf("Could not connect to database: %v", err) } log.Println("Database connected") ts = httptest.NewServer(http.HandlerFunc(helloHandler)) defer ts.Close() code := m.Run() os.Exit(code) } func TestDatabase(t *testing.T) { if err := db.Ping(); err != nil { t.Errorf("failed to ping database: %v", err) } } func TestHelloHandler(t *testing.T) { resp, err := http.Get(ts.URL + "/hello") if err != nil { t.Errorf("failed to send GET request to server: %v", err) } if resp.StatusCode != http.StatusOK { t.Fatalf("expected status code %d but got %d", http.StatusOK, resp.StatusCode) } if resp.Header.Get("Content-Type") != "text/plain; charset=utf-8" { t.Errorf("unexpected response content type") } }
Above we use the Go language's database/sql package to connect and The connection to the PostgreSQL database was tested; at the same time, we also used the net/http package to simulate the web server and send a GET request, and tested whether the response to the request was correct. Before testing the function, we initialized the database and ran the test server using the TestMain function.
- End-to-end testing
End-to-end testing refers to testing the entire application and simulating user operations to ensure that the program works as the user expects. In web applications, end-to-end testing generally tests the application's interface and interactions through automated tools.
The following is a simple example showing how to use the selenium package of the Go language and the chrome driver for end-to-end testing:
package main import ( "testing" "github.com/tebeka/selenium" ) func TestWebInterface(t *testing.T) { caps := selenium.Capabilities{"browserName": "chrome"} wd, err := selenium.NewRemote(caps, "") if err != nil { t.Fatalf("failed to create WebDriver: %v", err) } defer wd.Quit() if err := wd.Get("http://localhost:8080"); err != nil { t.Errorf("failed to visit homepage: %v", err) } elem, err := wd.FindElement(selenium.ByCSSSelector, "h1") if err != nil { t.Errorf("failed to find header element: %v", err) } txt, err := elem.Text() if err != nil { t.Errorf("failed to get header text: %v", err) } if txt != "Welcome to my website!" { t.Errorf("unexpected header text") } }
Above we use the selenium package of the Go language and the chrome driver The Web interface was simulated, the interface and interaction of the application were tested, and the h1 element in the page was checked to see if it met the requirements.
Summary
When testing web applications, unit testing, integration testing and end-to-end testing are all indispensable. Among them, unit testing is mainly used to test the smallest logical unit in the program; integration testing is used to test the dependencies and overall operation of the program; and end-to-end testing is used to test the user interface and interaction of the application. The above three testing methods can all be implemented using the testing package of Go language.
The above is the detailed content of Golang learning web application testing. For more information, please follow other related articles on the PHP Chinese website!
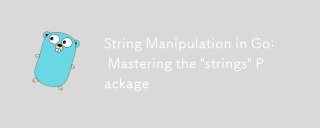
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
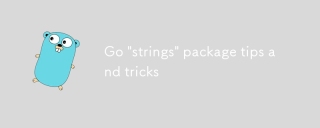
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
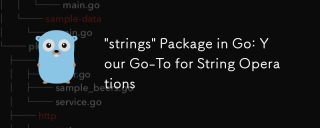
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
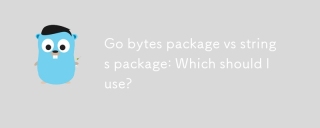
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
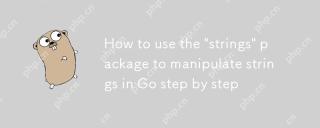
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
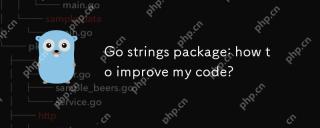
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
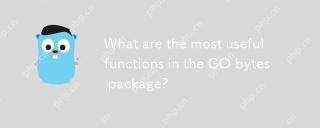
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
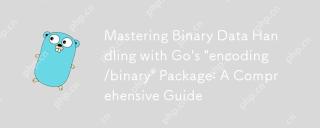
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
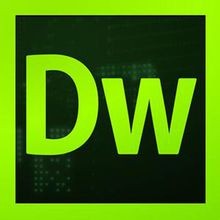
Dreamweaver CS6
Visual web development tools
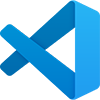
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
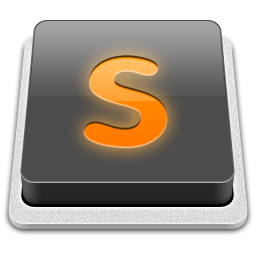
SublimeText3 Mac version
God-level code editing software (SublimeText3)
