


How to use PHP regular expressions to verify whether an email address actually exists
As email becomes an indispensable part of modern people's daily lives, it is increasingly important to verify the authenticity of email addresses. When developing web applications, regular expressions can be used to easily verify the validity of email addresses. However, verifying the authenticity of an email address requires more sophisticated techniques. In this article, we will introduce how to use PHP regular expressions to verify the authenticity of an email address.
Before we begin, we need to know what regular expressions are. A regular expression is a text pattern that is used to match a specific pattern of strings. In PHP, regular expressions can be matched using the preg_match() function. Here is a simple example:
$email = "example@email.com"; $pattern = "/[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,}/"; if (preg_match($pattern, $email)) { echo "Valid email."; } else { echo "Invalid email."; }
In the above example, we use regular expressions to verify whether an email address is valid. Regular expression patterns use the RFC 2822 specification. This specification defines the format of an email address, for example: username@domainname.com. The above regular expression uses character sets, repeating metacharacters, and character classes to match qualified email addresses. If the match is successful, the email address will be considered a valid email address.
However, this verification method only determines whether the format of the email address conforms to the specifications, and cannot verify the true existence of the email address. In order to verify the real existence of the email address, we need to interact with the email server.
The method to implement this process is to send an email to the mail server and wait for the server to return a response. If the server returns a response indicating that the email address is invalid, then the email address is considered invalid. Otherwise, the email address is considered valid. This process can be accomplished through PHP's SMTP class.
First, we need to establish a connection with the mail server through the SMTP class. The following is an example of establishing a connection with the Gmail SMTP server:
$host = "smtp.gmail.com"; $port = 587; $username = "example@gmail.com"; $password = "password"; $socket = fsockopen($host, $port, $errno, $errstr, 10); if (!is_resource($socket)) { die("$errstr ($errno)"); } if (substr(fgets($socket, 1024), 0, 3) !== "220") { die("Failed to connect to $host:$port"); } fwrite($socket, "EHLO example.com "); if (substr(fgets($socket, 1024), 0, 3) !== "250") { die("Failed to send EHLO command"); } fwrite($socket, "STARTTLS "); if (substr(fgets($socket, 1024), 0, 3) !== "220") { die("Failed to initiate TLS"); } stream_socket_enable_crypto($socket, true, STREAM_CRYPTO_METHOD_TLS_CLIENT); fwrite($socket, "AUTH LOGIN "); if (substr(fgets($socket, 1024), 0, 3) !== "334") { die("Failed to send AUTH LOGIN command"); } fwrite($socket, base64_encode($username)." "); if (substr(fgets($socket, 1024), 0, 3) !== "334") { die("Failed to send username"); } fwrite($socket, base64_encode($password)." "); if (substr(fgets($socket, 1024), 0, 3) !== "235") { die("Failed to authenticate"); }
In the above example, we used the Gmail SMTP server as an example. Through the fsockopen() function, we establish a socket connection with the mail server and send an identifier to the server through the EHLO command, indicating that our starttls command can be accepted. We then use the STARTTLS command to encrypt the socket connection. Next, we send the AUTH LOGIN command to the server and use base64 encoding to send the username and password to the server for authentication.
If the authentication is successful, we can start sending emails to the server. The following is an example of checking whether the email address actually exists:
$email = "example@email.com"; $host = "mx1.example.com"; fwrite($socket, "MAIL FROM:<me@example.com> "); if (substr(fgets($socket, 1024), 0, 3) !== "250") { die("Failed to send MAIL FROM command"); } fwrite($socket, "RCPT TO:<$email> "); $response = substr(fgets($socket, 1024), 0, 3); if ($response === "250" || $response === "251") { echo "Valid email."; } else { echo "Invalid email."; }
In the above example, we use the fwrite() function to send the MAIL FROM and RCPT TO commands to the mail server. The RCPT TO command is used to verify the true existence of the recipient address. If the response code returned by the server is 250 or 251, it means that the email address is valid. Otherwise, the email address is considered invalid.
Through the above method, we can use a combination of PHP regular expressions and SMTP classes to verify the true existence of the email address. Although this method requires interaction with the mail server, its verification results are more accurate and have important practical value.
The above is the detailed content of How to use PHP regular expressions to verify whether an email address actually exists. For more information, please follow other related articles on the PHP Chinese website!
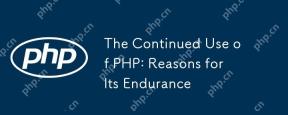
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
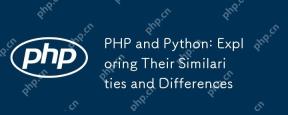
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
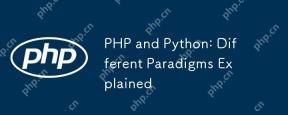
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
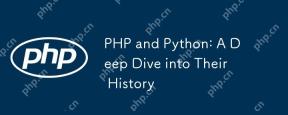
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
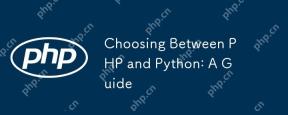
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
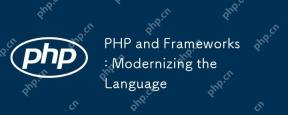
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
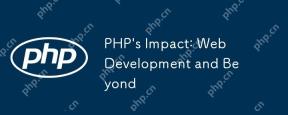
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
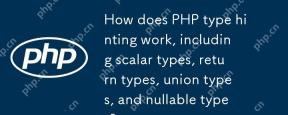
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
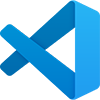
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software