When developing using PHP, we often encounter the problem of "Undefined index". This problem usually occurs when we try to access an undefined element when dealing with an array or object. This article will introduce the solution to this problem and help you better develop PHP.
- Understand the "Undefined index" error
First, we need to understand the meaning of the "Undefined index" error. This error occurs when we try to access an undefined array or object element. For example:
$sample_array = array( 'name' => 'John', 'age' => 30, ); echo $sample_array['email'];
In the above code, we are trying to access the email
element of the $sample_array
array, but the element does not exist. Therefore, PHP will return an "Undefined index" error.
- Cause of error
When an element is not defined, an "Undefined index" error occurs when we try to access it. This error usually occurs in the following situations:
- An element in the array is undefined;
- An attribute in the object is undefined;
- Key name Typos;
- The specific element in the data source (such as a form) does not exist.
After understanding the cause of the error, we need to solve the problem. Here are some solutions that may help.
- Check if an element exists
Luckily, we can check for an element before accessing it. This can be achieved by using PHP's isset()
function. For example:
$sample_array = array( 'name' => 'John', 'age' => 30, ); if(isset($sample_array['email'])) { echo $sample_array['email']; } else { echo 'Email is not defined.'; }
In the above code, we use the isset()
function to check whether the email
element in the $sample_array
array exists . If the element exists, we can safely access it. Otherwise, we will get an error message telling us that the element is undefined.
Similar checking methods can also be used for objects. For example:
class Person { public $name; public $age; } $person = new Person(); $person->name = 'John'; if(isset($person->email)) { echo $person->email; } else { echo 'Email is not defined.'; }
In the above code, we use the isset()
function to check whether the email
attribute in the $person
object exists . If the property exists, we can safely access it. Otherwise, we will get an error message telling us that the property is undefined.
- For array access errors
When the element is not defined, array access errors may appear in the loop body. For example:
$sample_array = array( 'name' => 'John', 'age' => 30, 'email' => 'john@example.com', ); foreach($sample_array as $key => $value) { echo $sample_array['phone']; }
In the above code, we are trying to use an undefined phone
element in the $sample_array
array. Since the element does not exist, PHP will return an "Undefined index" error.
In order to solve this problem, we need to check whether each element exists in the loop body before accessing:
$sample_array = array( 'name' => 'John', 'age' => 30, 'email' => 'john@example.com', ); foreach($sample_array as $key => $value) { if(isset($sample_array['phone'])) { echo $sample_array['phone']; } }
In the above code, we use isset()
Function to check whether the phone
element in the $sample_array
array exists. We will only access the element if it exists. This approach avoids "Undefined index" errors.
- Resolving data source errors
When processing form data, we often encounter "Undefined index" errors. This may be caused by missing some required fields in the submitted form. For example:
$name = $_POST['name']; $age = $_POST['age']; $email = $_POST['email'];
In the above code, we try to use the $_POST
variable to access the name
, age
, and email
Field. If any of these fields are missing from the submitted form, PHP will return an "Undefined index" error. To avoid this error, we need to check these fields and use default values to replace missing data:
$name = (isset($_POST['name'])) ? $_POST['name'] : ''; $age = (isset($_POST['age'])) ? $_POST['age'] : ''; $email = (isset($_POST['email'])) ? $_POST['email'] : '';
In the above code, we use the isset()
function to Check whether the name
, age
, and email
fields in the $_POST
variable exist. If these fields exist, we can use their values. Otherwise, we will use an empty string in place of the missing value.
- Conclusion
In PHP development, errors are always inevitable. "Undefined index" errors often appear when dealing with arrays or objects. To avoid this error, we need to check the data and replace missing data with default values. If you still run into this problem, be sure to double-check your code and check PHP's error messages for more information.
The above is the detailed content of Solution to PHP Notice: Undefined index:. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
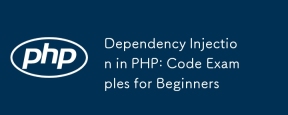
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
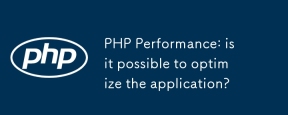
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
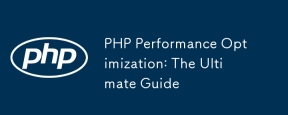
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
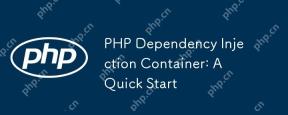
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
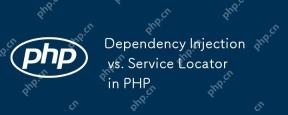
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
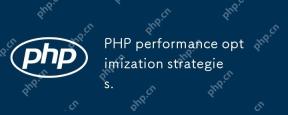
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
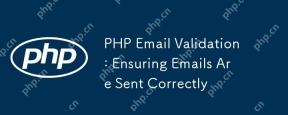
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
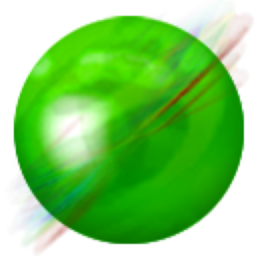
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
