Using Zipkin and Jaeger to implement distributed tracing in Beego
Using Zipkin and Jaeger to implement distributed tracing in Beego
With the popularity of microservices, the development of distributed systems has become more and more common. However, distributed systems also bring new challenges, such as how to track the flow of requests among various services, how to analyze and optimize the performance of services, etc. In these respects, distributed tracing solutions have become an increasingly important component. This article will introduce how to use Zipkin and Jaeger to implement distributed tracing in Beego.
Request tracing across multiple services is the primary goal of distributed tracing. Centralized log streams or metrics streams cannot solve this problem because these streams cannot provide correlation between services. A request may require multiple services to work together, and these services must be aware of the response times and behavior of other services. The traditional approach is to log various metrics and then relax the thresholds to avoid blocking when receiving requests. But this approach can hide problems such as glitches and performance issues. Distributed tracing is a solution for cross-service request tracing. In this approach, as a request flows between services, each service generates a series of IDs that will track the entire request.
Let's see how to implement distributed tracing in Beego.
Zipkin and Jaeger are currently the most popular distributed tracing solutions. Both tools support the OpenTracing API, enabling developers to log and trace requests across services in a consistent manner.
First, we need to install and start Zipkin or Jaeger, and then configure distributed tracing in the Beego application. In this article, we will use Zipkin.
Install Zipkin:
curl -sSL https://zipkin.io/quickstart.sh | bash -s java -jar zipkin.jar
Once Zipkin is launched, you can access its web UI via http://localhost:9411.
Next, we need to add support for the OpenTracing API in Beego. We can use the opentracing-go package and log cross-service requests and other events using the API it provides. An example tracking code is as follows:
import ( "github.com/opentracing/opentracing-go" ) func main() { // Initialize the tracer tracer, closer := initTracer() defer closer.Close() // Start a new span span := tracer.StartSpan("example-span") // Record some events span.SetTag("example-tag", "example-value") span.LogKV("example-key", "example-value") // Finish the span span.Finish() } func initTracer() (opentracing.Tracer, io.Closer) { // Initialize the tracer tracer, closer := zipkin.NewTracer( zipkin.NewReporter(httpTransport.NewReporter("http://localhost:9411/api/v2/spans")), zipkin.WithLocalEndpoint(zipkin.NewEndpoint("example-service", "localhost:80")), zipkin.WithTraceID128Bit(true), ) // Set the tracer as the global tracer opentracing.SetGlobalTracer(tracer) return tracer, closer }
In the above example, we first initialize the Zipkin tracker and then use it to record some events. We can add tags and key-value pairs and end the span by calling span.Finish().
Now, let’s add distributed tracing to our Beego application.
First, let’s add the opentracing-go and zipkin-go-opentracing dependencies. We can do this using go mod or manually installing packages.
go get github.com/opentracing/opentracing-go go get github.com/openzipkin/zipkin-go-opentracing
Then, we need to initialize the Zipkin tracker and Beego tracker middleware in the Beego application. The following is a sample code for Beego tracer middleware:
import ( "net/http" "github.com/astaxie/beego" opentracing "github.com/opentracing/opentracing-go" "github.com/openzipkin/zipkin-go-opentracing" ) func TraceMiddleware() func(http.ResponseWriter, *http.Request, http.HandlerFunc) { return func(w http.ResponseWriter, r *http.Request, next http.HandlerFunc) { // Initialize the tracer tracer, closer := initTracer() defer closer.Close() // Extract the span context from the HTTP headers spanCtx, err := tracer.Extract(opentracing.HTTPHeaders, opentracing.HTTPHeadersCarrier(r.Header)) if err != nil && err != opentracing.ErrSpanContextNotFound { beego.Error("failed to extract span context:", err) } // Start a new span span := tracer.StartSpan(r.URL.Path, ext.RPCServerOption(spanCtx)) // Set some tags span.SetTag("http.method", r.Method) span.SetTag("http.url", r.URL.String()) // Inject the span context into the HTTP headers carrier := opentracing.HTTPHeadersCarrier(r.Header) if err := tracer.Inject(span.Context(), opentracing.HTTPHeaders, carrier); err != nil { beego.Error("failed to inject span context:", err) } // Set the span as a variable in the request context r = r.WithContext(opentracing.ContextWithSpan(r.Context(), span)) // Call the next middleware/handler next(w, r) // Finish the span span.Finish() } } func initTracer() (opentracing.Tracer, io.Closer) { // Initialize the Zipkin tracer report := zipkinhttp.NewReporter("http://localhost:9411/api/v2/spans") defer report.Close() endpoint, err := zipkin.NewEndpoint("example-service", "localhost:80") if err != nil { beego.Error("failed to create Zipkin endpoint:", err) } nativeTracer, err := zipkin.NewTracer( report, zipkin.WithLocalEndpoint(endpoint), zipkin.WithTraceID128Bit(true)) if err != nil { beego.Error("failed to create Zipkin tracer:", err) } // Initialize the OpenTracing API tracer tracer := zipkinopentracing.Wrap(nativeTracer) // Set the tracer as the global tracer opentracing.SetGlobalTracer(tracer) return tracer, report }
In the above sample code, we define a middleware named TraceMiddleware. This middleware will extract the existing tracking context from the HTTP headers (if any) and use it to create a new tracker for the request. We also set the span in the request context so that all other middleware and handlers can access it. Finally, after the handler execution ends, we call the finish() method on the span so that Zipkin can record interdependency tracking across all services requested.
We also need to attach this middleware to our Beego router. We can do this using the following code in the router initialization code:
beego.InsertFilter("*", beego.BeforeRouter, TraceMiddleware())
Now, launch your Beego application and visit http://localhost:9411 to open the Zipkin UI to view the tracking data.
Implementing distributed tracing in a Beego application may seem complicated, but by using the opentracing-go and zipkin-go-opentracing libraries, we can easily add this functionality. This becomes increasingly important as we continue to increase the number and complexity of our services, allowing us to understand how our services work together and ensure they perform well throughout the request handling process.
The above is the detailed content of Using Zipkin and Jaeger to implement distributed tracing in Beego. For more information, please follow other related articles on the PHP Chinese website!
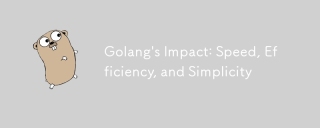
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
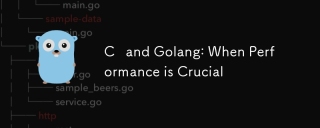
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
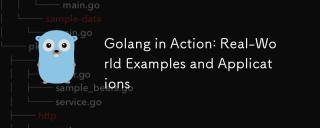
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
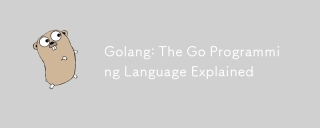
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
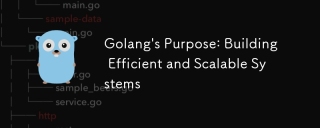
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
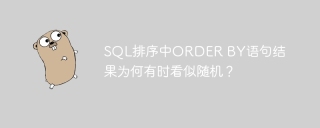
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
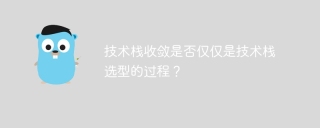
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
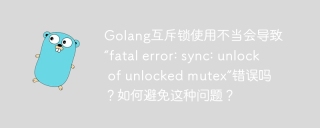
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
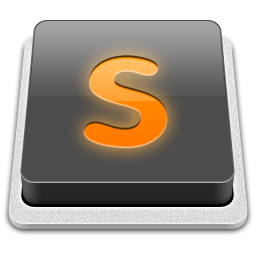
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
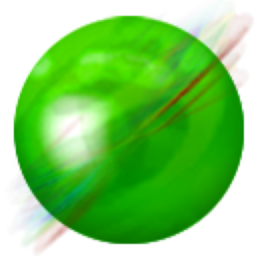
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment