With the development of the Internet, microservice architecture is becoming more and more popular among Internet companies. Spring Cloud provides a complete microservice solution based on Spring Boot. This article will introduce how to use the Spring Cloud framework to practice microservices.
1. Overview of microservice architecture
The so-called microservice architecture is to split the application into some smaller, autonomous service units, and use lightweight communication mechanisms between services. To work together, individual services can be built, deployed, tested, and scaled independently. The advantage of microservice architecture is that it can improve the productivity of the team and the elasticity and scalability of the system.
2. Spring Cloud Microservice Framework
Spring Cloud is the microservice solution of the Spring family. It provides a complete and rich toolkit based on Spring Boot. Spring Cloud uses multiple components (such as Eureka, Zuul, Hystrix, etc.) to build a complete microservice environment. The following are the main components of Spring Cloud:
1. Eureka: implements service registration and discovery, and provides a service registration center;
2. Feign: implements communication between services, simplifying RESTful calls;
3. Hystrix: Implement functions such as circuit breakers and downgrades to improve the robustness of the system;
4. Zuul: Provide API gateway services to achieve unified access and load balancing;
5. Config: Implement configuration Management provides a distributed configuration center.
3. Spring Cloud Microservice Practice
The following is a simple example to demonstrate how to use the Spring Cloud framework to build microservices. This example contains two services: a user service and an order service. The user service provides CRUD operations for user information, and the order service needs to call the user service to obtain user information.
1. Create a service registration center
First, we create a service registration center for registering and discovering services. Create a Spring Boot project to implement service registration and discovery through Spring Cloud's Eureka component.
Add dependencies:
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka-server</artifactId> </dependency>
Add the @EnableEurekaServer annotation on the startup class.
@SpringBootApplication @EnableEurekaServer public class EurekaServerApplication { public static void main(String[] args) { SpringApplication.run(EurekaServerApplication.class, args); } }
In the application.properties configuration file, configure the relevant information of Eureka Server.
2. Create a user service
Create a user service to provide CRUD operations for user information. Create a Spring Boot project to implement communication between services through Spring Cloud's Feign component.
Add dependencies:
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-openfeign</artifactId> </dependency>
Add the @EnableFeignClients annotation on the startup class.
@SpringBootApplication @EnableFeignClients public class UserServiceApplication { public static void main(String[] args) { SpringApplication.run(UserServiceApplication.class, args); } }
Create the UserService interface and define CRUD operations for user information. Use the @FeignClient annotation to specify the service to be called. In this interface, a call to OrderService is defined.
@FeignClient(name = "order-service", fallback = OrderServiceFallback.class) public interface OrderService { @GetMapping("/user/{userId}") UserDTO getUserById(@PathVariable("userId") Long userId); @PostMapping("/user") UserDTO createUser(@RequestBody UserDTO user); @PutMapping("/user") UserDTO updateUser(@RequestBody UserDTO user); @DeleteMapping("/user/{userId}") void deleteUser(@PathVariable("userId") Long userId); }
In the application.properties configuration file, configure Eureka related information and Feign's timeout.
3. Create an order service
Create an order service for processing order information. Create a Spring Boot project and use Spring Cloud's Ribbon and Feign components to call user services and achieve load balancing between services.
Add dependencies:
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-openfeign</artifactId> </dependency> org.springframework.cloud spring-cloud-starter-ribbon
Add @EnableFeignClients and @EnableDiscoveryClient annotations on the startup class.
@SpringBootApplication @EnableFeignClients @EnableDiscoveryClient public class OrderServiceApplication { public static void main(String[] args) { SpringApplication.run(OrderServiceApplication.class, args); } }
Create the OrderService interface and define CRUD operations for order information. In this interface, a call to UserService is defined.
public interface OrderService { @GetMapping("/order/{orderId}") OrderDTO getOrderById(@PathVariable("orderId") Long orderId); @PostMapping("/order") OrderDTO createOrder(@RequestBody OrderDTO order); @PutMapping("/order") OrderDTO updateOrder(@RequestBody OrderDTO order); @DeleteMapping("/order/{orderId}") void deleteOrder(@PathVariable("orderId") Long orderId); }
In the implementation class, use @Autowired to inject the UserService interface.
@Service public class OrderServiceImpl implements OrderService { @Autowired private UserService userService; // ... }
In the application.properties configuration file, configure Eureka-related information and Ribbon's load balancing method.
4. Summary
This article introduces how to build an environment based on microservice architecture through the Spring Cloud framework, and uses a simple example to demonstrate how to implement service registration and discovery, service Inter-communication, load balancing and other functions. Of course, this is just a simple starting point, and more scenarios and issues need to be considered in practical applications. Only through deeper learning and practice can the reliability and scalability of the system be better improved.
The above is the detailed content of Spring Cloud's elegant microservice practice. For more information, please follow other related articles on the PHP Chinese website!
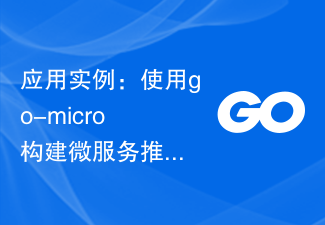
随着互联网应用的普及,微服务架构已成为目前比较流行的一种架构方式。其中,微服务架构的关键就是将应用拆分为不同的服务,通过RPC方式进行通信,实现松散耦合的服务架构。在本文中,我们将结合实际案例,介绍如何使用go-micro构建一款微服务推荐系统。一、什么是微服务推荐系统微服务推荐系统是一种基于微服务架构的推荐系统,它将推荐系统中的不同模块(如特征工程、分类
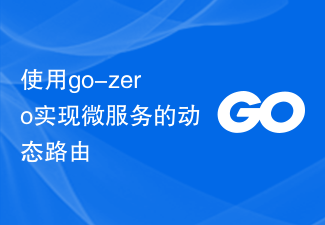
随着云计算和容器化技术的普及,微服务架构已成为现代化软件开发中的主流方案。而动态路由技术则是微服务架构中必不可少的一环。本文将介绍如何使用go-zero框架实现微服务的动态路由。一、什么是动态路由在微服务架构中,服务的数量和种类可能非常多,如何管理和发现这些服务是一项非常棘手的任务。传统的静态路由并不适用于微服务架构,因为服务数量以及运行时的状态都是动态变化
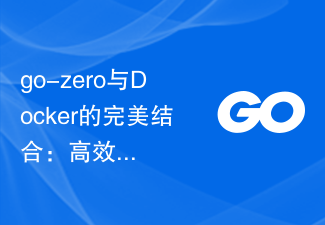
随着互联网的快速发展,微服务架构渐渐成为了业界的热门话题,而Docker作为容器化的利器,更是被广泛应用于微服务架构中的部署和运维。而今天我要介绍的是另一款非常优秀的微服务框架——go-zero,以及它与Docker的完美结合。一、什么是go-zerogo-zero是一款由饿了么点评公司开源的,基于Go语言构建的微服务框架。它的特点是高性能、易于使用和功能全
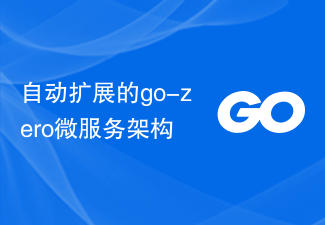
近年来,随着云计算和微服务架构的普及,越来越多的企业和开发者开始使用微服务架构来搭建自己的应用。然而,微服务架构也存在着一些问题,比如服务的扩展、管理、监控等方面。为了解决这些问题,很多开发者开始使用go-zero微服务框架。go-zero是一款基于Go语言开发的微服务框架,它提供了一系列的组件和工具,帮助开发者快速构建、管理和扩展自己的微服务。其中最重要的
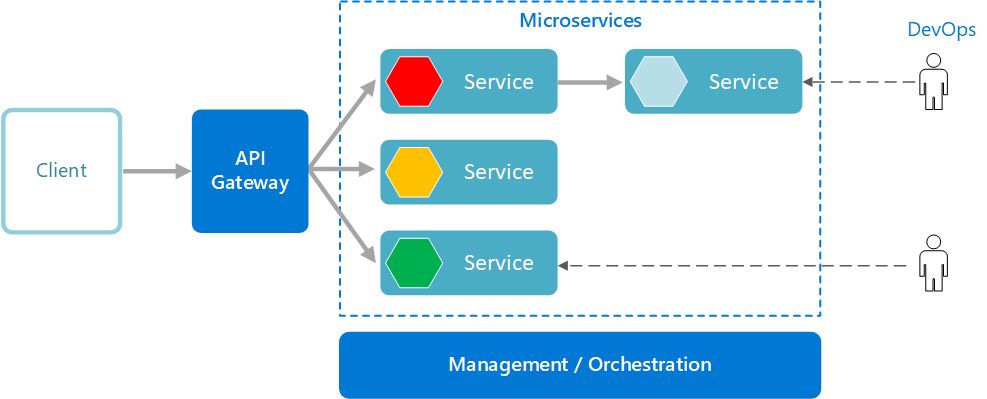
在选择适合微服务架构的编程语言时,Python是其中一种选择。它具有活跃的社区、更好的原型设计以及在开发人员中受欢迎等好处。它有一些限制,因此可以使用其他语言来避免它们。快速开发架构风格回顾与统计两种主要的开发架构风格是单体架构和微服务架构。Monolithic具有一体化的原则,并作为一个整体结构发挥作用,最适合小型开发项目或初创企业。当一个平台增长并且业务需要复杂的应用程序时,将其拆分为微服务架构是合理的。一些语言和框架更适合构建微服务架构。Java、Javascript和Python被列为微
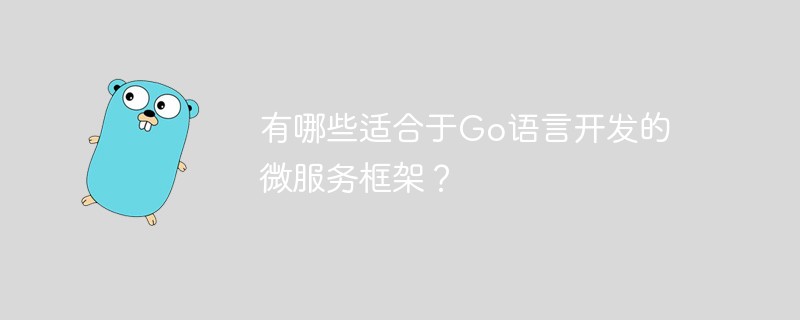
随着微服务架构的兴起,越来越多的开发者开始探索如何将应用程序拆分成小而独立的服务,并将它们组合成一个更大的应用。Go语言因其高效、简洁和并发性能出色的特点,成为了其中一个热门的用于微服务开发的语言。而本文将介绍一些适合于Go语言开发的微服务框架。GinGin是一款快速、灵活和轻量级的Web框架,具有丰富的功能和优雅的API。它通过HTTP路由机制和中间件来帮
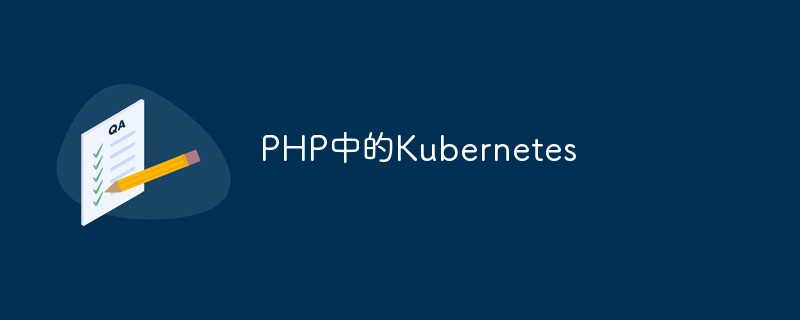
Kubernetes是近年来非常火热的容器编排和管理工具,PHP作为一种非常流行的Web开发语言,也需要适应这个趋势,通过Kubernetes来管理自己的应用。在本文中,我们将探讨如何在PHP应用中使用Kubernetes。一、Kubernetes概述Kubernetes是由Google公司开发的一个容器编排和管理工具,用于管理容器化应用。Kubernete
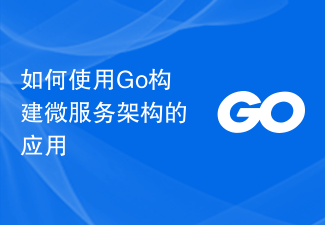
随着软件开发的不断发展,微服务架构已经逐渐成为了一种非常流行的架构模式。而在微服务架构中,Go语言作为一种高性能的编程语言也逐渐受到了越来越多的关注。那么,如何使用Go构建微服务架构的应用呢?下面将通过几个步骤来详细介绍。1.选择合适的Go框架选择合适的Go框架非常重要,它能够让我们更快地构建出一些基础服务,比如HTTP服务、日志服务、数据库服务等等。当前,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
