Learn to write command line tools in PHP
PHP is a popular programming language used for web development and writing command-line tools. As web applications evolve, more and more developers are beginning to realize the value of command line tools. This article will introduce how to learn to write command line tools using PHP.
Command line capabilities of PHP
PHP is a powerful web programming language, but it can also be used to write command line tools. PHP version 5.0 introduced the CLI (Command Line Interface), which allows PHP code to be run from the command line without the need for a web server. The PHP CLI allows you to write web server-independent applications.
PHP CLI provides many functions, such as:
- Command line parameter processing: You can use PHP's CLI function to pass parameters when executing a PHP program.
- Multi-Threading: The PHP CLI allows you to create multiple processes and threads.
- Interactive shell: The PHP CLI provides an interactive shell that you can work with using the PHP language.
When writing command line tools, you can use various features and functions of PHP. You can use PHP's file operation functions, database functions, network functions, and other functions to enhance your applications.
Writing a Hello World program
We will start by creating a simple Hello World program. Here is our code:
<?php echo "Hello, World!"; ?>
To run this program, you need to open a command line terminal and enter the following command:
php helloworld.php
This will run the code in the helloworld.php file. You should see the output "Hello, World!" displayed in the terminal.
Handling command line arguments
Your CLI application may need to handle command line arguments. PHP provides several functions to help you handle command line parameters. PHP scripts can use parameters passed by the user from the command line. We'll see how to use them in the examples below.
<?php // 获取命令行参数 $name = $argv[1]; $age = $argv[2]; // 显示输出 echo "Hello $name, you are $age years old!"; ?>
To run this script, you need to execute the following command:
php user.php John 25
This will pass two parameters, 'John' and '25' to our program, and then the program will output "Hello John, you are 25 years old!".
Handling User Input
When writing a CLI application, you may need to get input from the user. PHP provides some functions to read user input from the command line. Here is a simple example:
<?php echo "What is your name? "; $name = trim(fgets(STDIN)); echo "Hello, $name"; ?>
This script will prompt the user for their name and then will read their input and use it for the output. To run this script, type the following command in the command line terminal:
php getinput.php
You should see the "what is your name?" message, enter your name, and the script will output "Hello, your name ".
Debugging CLI Applications
When you write a CLI application, as with web applications, debugging is a common task. PHP provides some debugging features, such as xdebug, which can be used to detect and fix errors. xdebug can use PHP's command line environment for debugging. You can enable xdebug by:
- First enable xdebug on your PHP. You can edit the php.ini file and add the following code in the file:
zend_extension="C: mppphpextphp_xdebug.dll" xdebug.remote_enable=1 xdebug.remote_autostart=1
- Then you can start xdebug by running the following command:
php -dxdebug.remote_autostart=1 -dxdebug.remote_mode=req test.php
This xdebug will be started and then the code in the test.php file will be run.
Conclusion
PHP is a popular programming language that can be used to write web applications and command line tools. The PHP CLI feature provides many features that allow you to write powerful CLI applications. In this article we saw how to write a simple CLI application and handle command line arguments and user input. Finally, we covered how to use xdebug to debug CLI applications. This can help you fix errors in your code and achieve better performance.
The above is the detailed content of Learn to write command line tools in PHP. For more information, please follow other related articles on the PHP Chinese website!
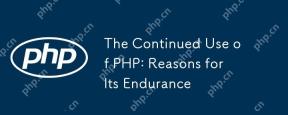
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
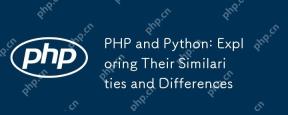
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
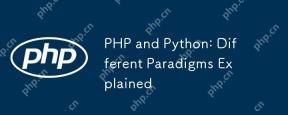
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
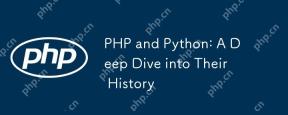
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
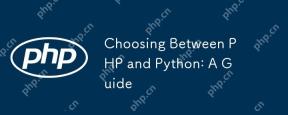
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
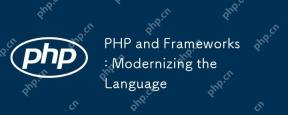
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
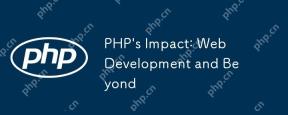
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
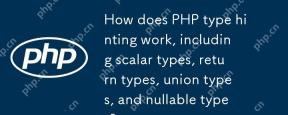
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
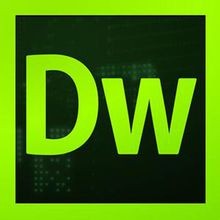
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
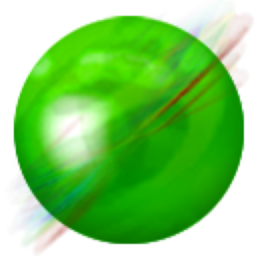
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment