Yii framework is a powerful PHP framework that can help developers quickly build high-performance, scalable web applications. This article will introduce how to use the Yii framework to create a Q&A website.
- Environment preparation
Before starting, we need to ensure that necessary software and tools such as PHP and MySQL have been correctly configured in the local development environment. At the same time, we also need to install the Yii framework to facilitate subsequent development work.
Installing the Yii framework is very simple, just execute the following command:
composer create-project yiisoft/yii2-app-basic <project_name>
where <project_name></project_name>
is the name of the current project.
- Database design
Before creating a Q&A website, we need to design the relevant database structure. In this article, we will use the following database tables:
- user: used to store user information, including user name, password, email, etc.;
- question: used to store questions Information, including question title, content, release time, etc.;
- answer: used to store answer information, including answer content, answer time, etc.
Here we use MySQL as the back-end database, and create the corresponding database and table through the following commands:
CREATE DATABASE IF NOT EXISTS my_db; USE my_db; CREATE TABLE IF NOT EXISTS `user` ( `id` INT UNSIGNED AUTO_INCREMENT, `username` VARCHAR(64) NOT NULL, `password` VARCHAR(64) NOT NULL, `email` VARCHAR(64) NOT NULL, `created_at` TIMESTAMP DEFAULT CURRENT_TIMESTAMP, PRIMARY KEY (`id`) ); CREATE TABLE IF NOT EXISTS `question` ( `id` INT UNSIGNED AUTO_INCREMENT, `title` VARCHAR(255) NOT NULL, `content` TEXT, `user_id` INT UNSIGNED NOT NULL, `created_at` TIMESTAMP DEFAULT CURRENT_TIMESTAMP, PRIMARY KEY (`id`), FOREIGN KEY (`user_id`) REFERENCES user(`id`) ); CREATE TABLE IF NOT EXISTS `answer` ( `id` INT UNSIGNED AUTO_INCREMENT, `content` TEXT, `question_id` INT UNSIGNED NOT NULL, `user_id` INT UNSIGNED NOT NULL, `created_at` TIMESTAMP DEFAULT CURRENT_TIMESTAMP, PRIMARY KEY (`id`), FOREIGN KEY (`question_id`) REFERENCES question(`id`), FOREIGN KEY (`user_id`) REFERENCES user(`id`) );
Note that we have set foreign keys in the table to associate different data sheet.
- Create a model
In the Yii framework, models are the most commonly used tools for operating databases. We need to create corresponding model files to operate the database tables created previously.
In the models
folder under the application root directory, we create three model files User.php
, Question.php
,Answer.php
. Taking User.php
as an example, the code is as follows:
<?php namespace appmodels; use yiidbActiveRecord; class User extends ActiveRecord { public function rules() { return [ [['username', 'password', 'email'], 'required'], ['email', 'email'], ['username', 'unique'], ]; } public static function findByUsername($username) { return static::findOne(['username' => $username]); } public function validatePassword($password) { return $this->password === md5($password); } public function getQuestions() { return $this->hasMany(Question::className(), ['user_id' => 'id']); } public function getAnswers() { return $this->hasMany(Answer::className(), ['user_id' => 'id']); } }
In this file, we define the attributes of the model, validation rules, query methods and relationships, etc.
- Creating Controllers
Controllers are tools used to handle routing and responding to requests. In the controllers
folder under the application root directory, we create three controller files SiteController.php
, QuestionController.php
, AnswerController.php
. Taking SiteController.php
as an example, the code is as follows:
<?php namespace appcontrollers; use yiiwebController; class SiteController extends Controller { public function actionIndex() { return $this->render('index'); } }
In this file, we define a method named actionIndex
for rendering the homepage template.
- Create a view
The view is the user interface part of the application. We need to create the corresponding view file to render the content. In the views
folder under the application root directory, we create three folders site
, question
, answer
, corresponding to the previous Create three controllers.
In the views/site
folder, we create a file named index.php
for rendering the homepage template. The code is as follows:
<h1 id="Welcome-to-the-Question-Answer-website">Welcome to the Question & Answer website!</h1>
In the views/question
folder, we create a file named index.php
for rendering the question list page. The code is as follows:
<h1 id="Questions">Questions</h1> <?php foreach ($questions as $question): ?> <div> <h2><?= $question->title ?></h2> <p><?= $question->content ?></p> </div> <?php endforeach; ?>
In the views/answer
folder, we create a file named create.php
for rendering the answer editing page. The code is as follows:
<h1 id="Create-Answer">Create Answer</h1> <?= $this->render('_form', ['model' => $model]) ?>
- Create routing
In the Yii framework, routing is used to map URL addresses to corresponding controllers and methods. We need to create the corresponding routing rules in the web.php
file in the config
folder in the application root directory. The code is as follows:
return [ 'components' => [ 'urlManager' => [ 'enablePrettyUrl' => true, 'showScriptName' => false, 'rules' => [ '' => 'site/index', 'question' => 'question/index', 'answer/create/<question_id:d+>' => 'answer/create', ], ], ], ];
Note that we used the dynamic parameter question_id
in the answer/create
route. This parameter will be used when creating the answer.
- Create an authorization system
In the Q&A website, users need to log in to ask and answer questions. We need to create a basic authorization system to implement user login and registration functions.
In SiteController.php
, we added two methods actionLogin
and actionSignup
for rendering login and registration pages. In UserController.php
, we added a method named actionCreate
to handle user registration requests. The specific code implementation is omitted.
- Implementing the Q&A function
In the Q&A website, users need to ask and answer questions. We need to create relevant functions to implement these two operations.
In QuestionController.php
, we added two methods actionIndex
and actionCreate
for rendering the question list and question editing page. In QuestionController.php
, we created a method named actionCreate
to handle question creation requests. The specific code implementation is omitted.
In AnswerController.php
, we created a method named actionCreate
to handle the answer creation request. The specific code implementation is omitted.
- Test
After the above development work, we have completed a basic Q&A website. We can open the homepage by visiting http://localhost/<project_name></project_name>
, and open the question list by visiting http://localhost/<project_name>/question</project_name>
. We can also ask and answer questions through registered users.
The above is the detailed content of Create a Q&A website using Yii framework. For more information, please follow other related articles on the PHP Chinese website!
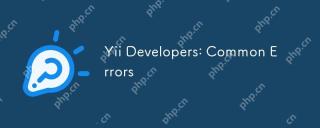
ThemostcommonerrorsinYiiframeworkare"UnknownProperty","InvalidConfiguration","ClassNotFound",and"ValidationErrors".1."UnknownProperty"errorsoccurwhenaccessingnon-existentproperties;ensurepropertiesexi
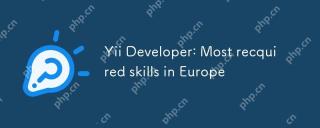
The key skills that European Yii developers need to possess include: 1. Yii framework proficiency, 2. PHP proficiency, 3. Database management, 4. Front-end skills, 5. RESTful API development, 6. Version control system, 7. Testing and debugging, 8. Security knowledge, 9. Agile methodology, 10. Soft skills, 11. Localization and internationalization, 12. Continuous learning, these skills make developers stand out in the European market.
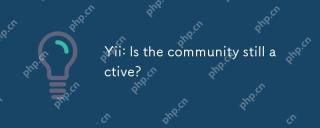
Yes,theYiicommunityisstillactiveandvibrant.1)TheofficialYiiforumremainsaresourcefordiscussionsandsupport.2)TheGitHubrepositoryshowsregularcommitsandpullrequests,indicatingongoingdevelopment.3)StackOverflowcontinuestohostYii-relatedquestionsandhigh-qu
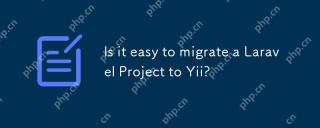
Migratingalaravel Projecttoyiiishallingbutachieffable WITHIEFLEFLANT.1) Mapoutlaravel component likeroutes, Controllers, Andmodels.2) Translatelaravel's SartisancommandeloequentTooyii's giiandetiverecordeba
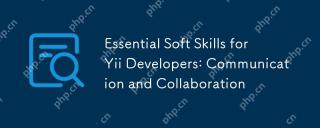
Soft skills are crucial to Yii developers because they facilitate team communication and collaboration. 1) Effective communication ensures that the project is progressing smoothly, such as through clear API documentation and regular meetings. 2) Collaborate to enhance team interaction through Yii's tools such as Gii to improve development efficiency.
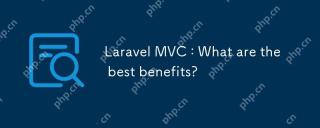
Laravel'sMVCarchitectureoffersenhancedcodeorganization,improvedmaintainability,andarobustseparationofconcerns.1)Itkeepscodeorganized,makingnavigationandteamworkeasier.2)Itcompartmentalizestheapplication,simplifyingtroubleshootingandmaintenance.3)Itse
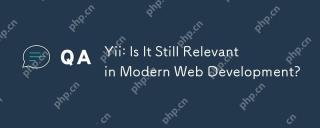
Yiiremainsrelevantinmodernwebdevelopmentforprojectsneedingspeedandflexibility.1)Itoffershighperformance,idealforapplicationswherespeediscritical.2)Itsflexibilityallowsfortailoredapplicationstructures.However,ithasasmallercommunityandsteeperlearningcu
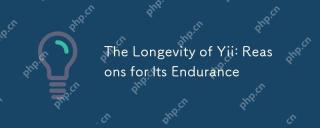
Yii frameworks remain strong in many PHP frameworks because of their efficient, simplicity and scalable design concepts. 1) Yii improves development efficiency through "conventional optimization over configuration"; 2) Component-based architecture and powerful ORM system Gii enhances flexibility and development speed; 3) Performance optimization and continuous updates and iterations ensure its sustained competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
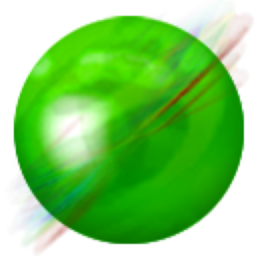
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version
