Laravel's MVC architecture offers enhanced code organization, improved maintainability, and a robust separation of concerns. 1) It keeps code organized, making navigation and teamwork easier. 2) It compartmentalizes the application, simplifying troubleshooting and maintenance. 3) It separates data, business, and presentation logic, enhancing resilience and allowing parallel development.
When diving into Laravel's MVC architecture, the question often arises: what are the best benefits it offers? From my experience, the standout advantages include enhanced code organization, improved maintainability, and a robust separation of concerns. Let's unpack these benefits and explore how they can transform your development process.
Laravel's MVC (Model-View-Controller) architecture isn't just a fancy buzzword; it's a game-changer for developers. Imagine your code as a well-organized library where every book is in its right place. That's what Laravel's MVC does for your codebase. It splits your application into three distinct parts: Models for handling data and business logic, Views for presenting data to the user, and Controllers for managing the flow between Models and Views. This separation isn't just about neatness; it's about efficiency and scalability.
Take a look at this simple example of how a basic MVC structure might look in Laravel:
// app/Http/Controllers/UserController.php namespace App\Http\Controllers; use App\Models\User; use Illuminate\Http\Request; class UserController extends Controller { public function index() { $users = User::all(); return view('users.index', ['users' => $users]); } } // app/Models/User.php namespace App\Models; use Illuminate\Database\Eloquent\Model; class User extends Model { protected $fillable = ['name', 'email']; } // resources/views/users/index.blade.php <!DOCTYPE html> <html> <body> @foreach ($users as $user) <p>{{ $user->name }} - {{ $user->email }}</p> @endforeach </body> </html>
This example showcases how Laravel's MVC keeps things tidy. The UserController
fetches data from the User
model and passes it to the view, which then displays the data. It's like having a clear roadmap for your application's logic and presentation.
Now, let's delve deeper into the benefits:
Enhanced Code Organization: When your project grows, maintaining a monolithic codebase becomes a nightmare. Laravel's MVC architecture keeps your code organized from the get-go. Models handle database interactions, Controllers manage the logic, and Views take care of the presentation. This organization makes it easier to navigate and understand your project, especially when working in a team. I've seen projects where developers can jump in and start contributing almost immediately because the structure is so clear.
Improved Maintainability: Ever tried to fix a bug in a spaghetti codebase? It's like finding a needle in a haystack. With Laravel's MVC, each part of your application is neatly compartmentalized. If there's an issue with data handling, you go straight to the Model. If it's about how data is displayed, you check the View. This separation makes troubleshooting and maintenance a breeze. I once had to refactor a large application, and thanks to Laravel's MVC, it was like performing surgery with a laser instead of a chainsaw.
Robust Separation of Concerns: This is the cornerstone of MVC. By keeping the data logic, business logic, and presentation logic separate, you're not just making your code cleaner; you're making it more resilient to changes. If you need to update your database schema, you touch the Model, not the View. If you want to change how data is displayed, you modify the View without affecting the data logic. This separation allows for parallel development, where different team members can work on different parts of the application without stepping on each other's toes.
Reusability and Flexibility: With a well-structured MVC, components become reusable. Need to use the same data handling logic in another part of your application? Just reuse the Model. Want to display the same data in different formats? Create different Views. This flexibility is a godsend when you're scaling your application or building a suite of related applications.
Easier Testing: Unit testing becomes much simpler with MVC. You can test your Models, Controllers, and Views independently. This isolation makes it easier to write and maintain tests, ensuring your application remains robust and bug-free. I've seen projects where the test coverage increased significantly after adopting Laravel's MVC, leading to fewer production issues.
While Laravel's MVC offers these stellar benefits, it's not without its challenges. One potential pitfall is overcomplicating your application by creating too many layers. It's tempting to create a new Model or Controller for every little thing, but this can lead to a bloated codebase. My advice? Keep it simple. Use MVC to guide your structure, but don't let it dictate every decision. Another common mistake is neglecting the separation of concerns, where business logic creeps into Controllers or Views. Stay vigilant and keep your layers clean.
In conclusion, Laravel's MVC architecture is a powerful tool that can significantly enhance your development workflow. It offers a structured approach to building applications that are easier to maintain, scale, and test. From my experience, embracing MVC not only improves the quality of your code but also makes the development process more enjoyable and collaborative. So, dive into Laravel's MVC, and watch your projects transform into well-organized, maintainable masterpieces.
The above is the detailed content of Laravel MVC : What are the best benefits?. For more information, please follow other related articles on the PHP Chinese website!
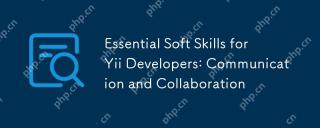
Soft skills are crucial to Yii developers because they facilitate team communication and collaboration. 1) Effective communication ensures that the project is progressing smoothly, such as through clear API documentation and regular meetings. 2) Collaborate to enhance team interaction through Yii's tools such as Gii to improve development efficiency.
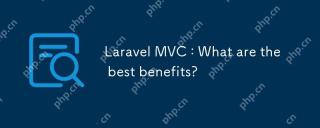
Laravel'sMVCarchitectureoffersenhancedcodeorganization,improvedmaintainability,andarobustseparationofconcerns.1)Itkeepscodeorganized,makingnavigationandteamworkeasier.2)Itcompartmentalizestheapplication,simplifyingtroubleshootingandmaintenance.3)Itse
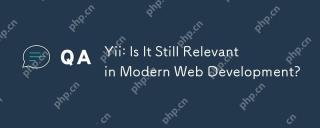
Yiiremainsrelevantinmodernwebdevelopmentforprojectsneedingspeedandflexibility.1)Itoffershighperformance,idealforapplicationswherespeediscritical.2)Itsflexibilityallowsfortailoredapplicationstructures.However,ithasasmallercommunityandsteeperlearningcu
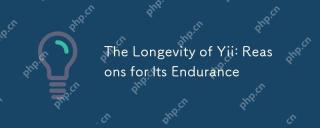
Yii frameworks remain strong in many PHP frameworks because of their efficient, simplicity and scalable design concepts. 1) Yii improves development efficiency through "conventional optimization over configuration"; 2) Component-based architecture and powerful ORM system Gii enhances flexibility and development speed; 3) Performance optimization and continuous updates and iterations ensure its sustained competitiveness.
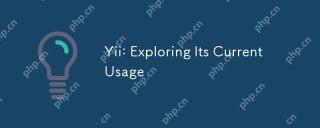
Yii is still suitable for projects that require high performance and flexibility in modern web development. 1) Yii is a high-performance framework based on PHP, following the MVC architecture. 2) Its advantages lie in its efficient, simplified and component-based design. 3) Performance optimization is mainly achieved through cache and ORM. 4) With the emergence of the new framework, the use of Yii has changed.
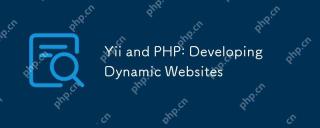
Yii and PHP can create dynamic websites. 1) Yii is a high-performance PHP framework that simplifies web application development. 2) Yii provides MVC architecture, ORM, cache and other functions, which are suitable for large-scale application development. 3) Use Yii's basic and advanced features to quickly build a website. 4) Pay attention to configuration, namespace and database connection issues, and use logs and debugging tools for debugging. 5) Improve performance through caching and optimization queries, and follow best practices to improve code quality.
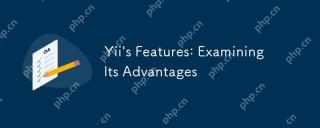
The Yii framework stands out in the PHP framework, and its advantages include: 1. MVC architecture and component design to improve code organization and reusability; 2. Gii code generator and ActiveRecord to improve development efficiency; 3. Multiple caching mechanisms to optimize performance; 4. Flexible RBAC system to simplify permission management.
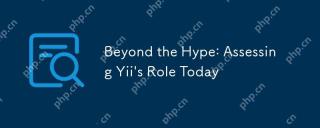
Yii remains a powerful choice for developers. 1) Yii is a high-performance PHP framework based on the MVC architecture and provides tools such as ActiveRecord, Gii and cache systems. 2) Its advantages include efficiency and flexibility, but the learning curve is steep and community support is relatively limited. 3) Suitable for projects that require high performance and flexibility, but consider the team technology stack and learning costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
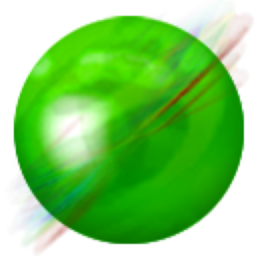
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
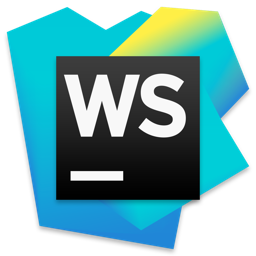
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
