How to use Redis to implement delay queue in Golang
Delay queue is a very practical message processing method. It delays the message for a period of time before processing. It is generally used to implement tasks such as task scheduling and scheduled tasks. . In actual development, Redis is a very commonly used cache database. It provides functions similar to message queues, so we can use Redis to implement delay queues. This article will introduce how to implement a delay queue using Golang and Redis.
- ZSET of Redis
Redis provides the data structure of sorted sets (ordered sets), which we can use to implement a delay queue. In sorted sets, each element has a score attribute, which is used to indicate the weight of the element. Sorted sets store elements in ascending order of score. Elements with the same score will be sorted according to their members. We can encapsulate each task into an element and use the time it takes to execute the task as the score of the element.
- Implementation of delay queue
Specifically, we can use Redis’s ZADD command to add tasks to the delay queue. For example:
//添加任务到延迟队列 func AddTaskToDelayQueue(taskId string, delayTime int64) error { _, err := redisClient.ZAdd("DelayedQueue", redis.Z{ Score: float64(time.Now().Unix() + delayTime), Member: taskId, }).Result() if err != nil { return err } return nil }
In the above code, we use Redis's ZADD command to add a task to the sorted set named "DelayedQueue". Among them, delayTime indicates the time the task needs to be postponed, and Score is the current time plus the delay time, that is, the timestamp when the task needs to be executed.
In actual business scenarios, we can obtain the element with the smallest score in the delay queue before task execution, that is, the most recent task that needs to be executed:
//获取延迟任务队列中最近需要执行的任务id func GetNextTaskFromDelayQueue() (string, error) { now := time.Now().Unix() items, err := redisClient.ZRangeByScore("DelayedQueue", redis.ZRangeBy{ Min: "-inf", Max: strconv.FormatInt(now, 10), Offset: 0, Count: 1, }).Result() if err != nil { return "", err } if len(items) == 0 { return "", nil } return items[0], nil }
In the above code, we use Redis's ZRangeByScore command obtains the elements in the delay queue whose score is less than or equal to the current timestamp, and then takes the first element in the list as the next task to be executed.
- Processing after task execution
After we obtain the tasks that need to be executed from the delay queue, we can move the tasks from the to-be-executed list to the executed list , so that we can count the execution of tasks.
//将已经执行的任务移除 func RemoveTaskFromDelayQueue(taskId string) error { _, err := redisClient.ZRem("DelayedQueue", taskId).Result() if err != nil { return err } return nil }
- Complete code example
We integrated the above code together and added some error handling and log information to get a complete code example:
package delayqueue import ( "strconv" "time" "github.com/go-redis/redis" ) var redisClient *redis.Client //初始化redis连接 func InitRedis(redisAddr string, redisPassword string) error { redisClient = redis.NewClient(&redis.Options{ Addr: redisAddr, Password: redisPassword, DB: 0, }) _, err := redisClient.Ping().Result() if err != nil { return err } return nil } //添加任务到延迟队列 func AddTaskToDelayQueue(taskId string, delayTime int64) error { _, err := redisClient.ZAdd("DelayedQueue", redis.Z{ Score: float64(time.Now().Unix() + delayTime), Member: taskId, }).Result() if err != nil { return err } return nil } //获取延迟任务队列中最近需要执行的任务id func GetNextTaskFromDelayQueue() (string, error) { now := time.Now().Unix() items, err := redisClient.ZRangeByScore("DelayedQueue", redis.ZRangeBy{ Min: "-inf", Max: strconv.FormatInt(now, 10), Offset: 0, Count: 1, }).Result() if err != nil { return "", err } if len(items) == 0 { return "", nil } return items[0], nil } //将已经执行的任务移除 func RemoveTaskFromDelayQueue(taskId string) error { _, err := redisClient.ZRem("DelayedQueue", taskId).Result() if err != nil { return err } return nil }
- Summary
This article introduces how to use Golang and Redis to implement a delay queue. By using the ZSET data structure, we can easily implement a delay queue, which is very practical in actual development. In addition to delay queues, Redis also provides many other data structures and functions, which are worth exploring and using.
The above is the detailed content of How to use redis to implement delay queue in Golang.. For more information, please follow other related articles on the PHP Chinese website!
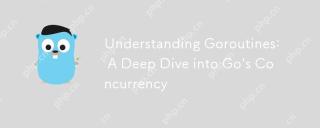
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
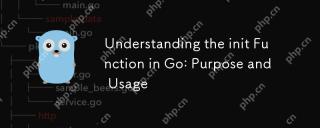
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
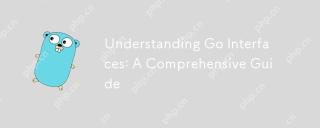
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
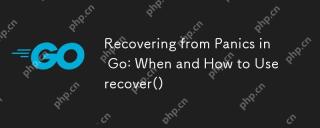
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
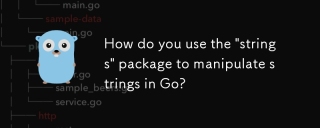
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
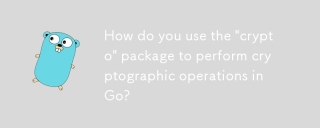
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
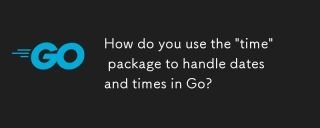
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
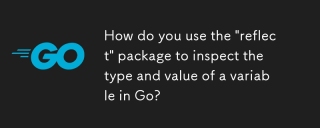
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
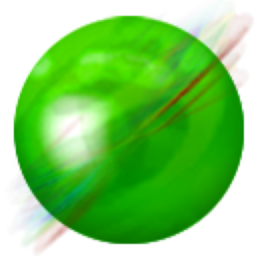
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
