How to use Mysql for multi-table joint query in ThinkPHP6
With the rapid development of the Internet, data storage and processing are becoming more and more important. For large websites, multi-table joint query is a very common operation. In this article, we will explore how to use Mysql in ThinkPHP6 to perform multi-table joint queries to obtain the data we need.
1. Create data tables
First, we need to create multiple tables and connect them. We can first create two tables: user and order. The user table contains the user's basic information (such as name, age, gender, etc.). The order table records the user's order information (such as order number, order time, order amount, etc.). These two tables can be linked by user ID. In the user table, we need to add a userID field as the primary key. In the order table, we need to add a userID field as a foreign key to associate with the userID field in the user table.
2. Writing the model
In ThinkPHP6, we can access the database by defining a model. We need to create a user model and an order model, corresponding to the above two tables respectively. In the user model, we need to define a method getUserJoinOrder() to jointly query the user table and order table and return the required data. The code is implemented as follows:
<?php namespace appmodel; use thinkacadeDb; use thinkModel; class UserModel extends Model { protected $table = 'user'; protected $pk = 'userID'; public function getUserJoinOrder() { $result = Db::table('user') ->alias('u') ->join('order o', 'u.userID = o.userID', 'left') ->select(); return $result; } }
In the above code, we use the table and alias methods under the think acadeDb namespace to specify the table name and alias. The join method is used to perform joint queries on multiple tables. It should be noted that in the join method, we need to specify the related fields of the two tables and the related method (left join, right join or inner join). Finally, we use the select method to execute the query and return the results.
3. Write the controller
In the controller, we can call methods in the model to access the database and pass the obtained data to the view layer. In this example, we create a UserController and write the getUserJoinOrder() method to call the getUserJoinOrder() method in the model. The code is implemented as follows:
<?php namespace appcontroller; use appmodelUserModel; use thinkController; class UserController extends Controller { public function getUserJoinOrder() { $userModel = new UserModel(); $users = $userModel->getUserJoinOrder(); $this->assign('users', $users); return $this->fetch('user_list'); } }
In the above code, we use the assign and fetch methods under the thinkController namespace to pass data to the view layer and render the view.
4. Write the view
Finally, we need to create a view to display the data we obtained. In this example, we create a user_list.html file for display. The code is implemented as follows:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>用户列表</title> </head> <body> <table border="1"> <tr> <th>用户ID</th> <th>姓名</th> <th>年龄</th> <th>性别</th> <th>订单号</th> <th>订单时间</th> <th>订单金额</th> </tr> <?php foreach($users as $user): ?> <tr> <td><?php echo $user['userID']; ?></td> <td><?php echo $user['name']; ?></td> <td><?php echo $user['age']; ?></td> <td><?php echo $user['gender']; ?></td> <td><?php echo $user['orderID']; ?></td> <td><?php echo $user['order_time']; ?></td> <td><?php echo $user['order_price']; ?></td> </tr> <?php endforeach; ?> </table> </body> </html>
In the above code, we use PHP's foreach loop to traverse the obtained data and display it in an HTML table.
Summary
The above are the steps and code implementation for using Mysql to perform multi-table joint queries in ThinkPHP6. By building related tables, writing models, controllers, and views, we can get the data we need quickly and efficiently. At the same time, when writing models, we need to pay attention to the fields and related methods associated with multiple tables to ensure the accuracy of query results.
The above is the detailed content of How to use Mysql for multi-table joint query in ThinkPHP6. For more information, please follow other related articles on the PHP Chinese website!
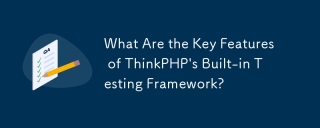
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
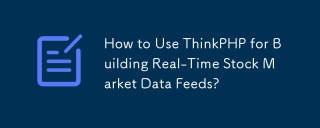
Article discusses using ThinkPHP for real-time stock market data feeds, focusing on setup, data accuracy, optimization, and security measures.

The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
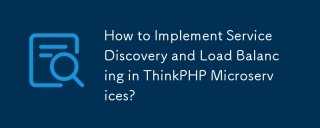
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
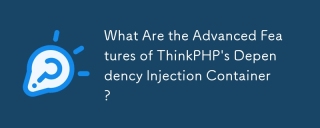
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
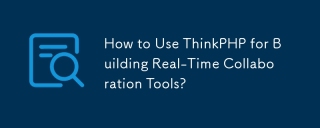
The article discusses using ThinkPHP to build real-time collaboration tools, focusing on setup, WebSocket integration, and security best practices.
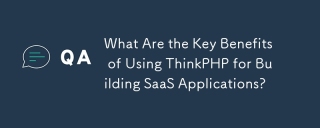
ThinkPHP benefits SaaS apps with its lightweight design, MVC architecture, and extensibility. It enhances scalability, speeds development, and improves security through various features.
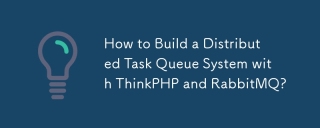
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
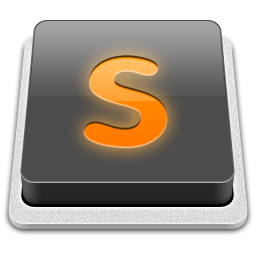
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
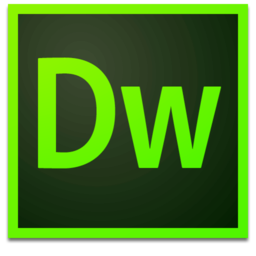
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool